Mastering Error Handling in Spring Integration
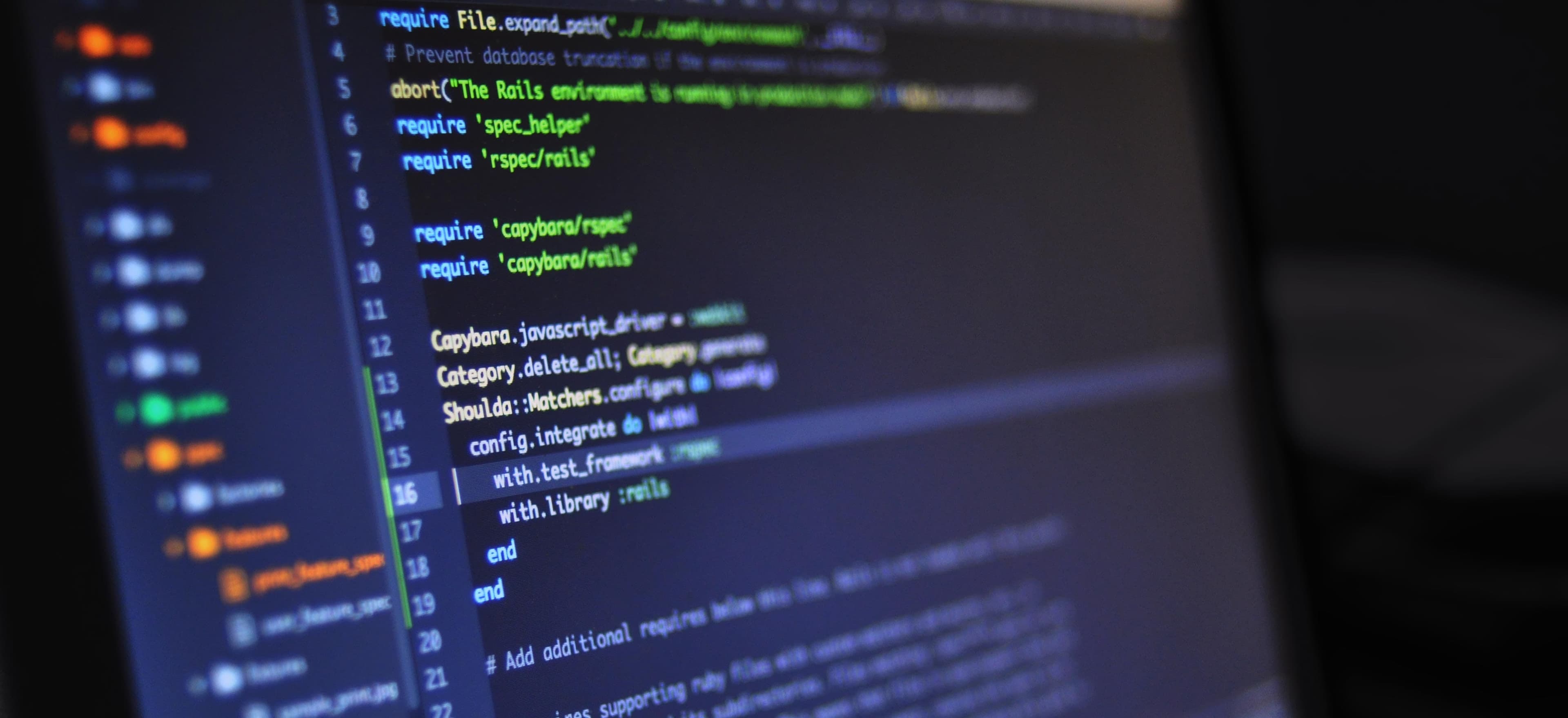
- Published on
Mastering Error Handling in Spring Integration
Spring Integration is a powerful framework that allows developers to build robust and scalable applications that communicate seamlessly in a distributed environment. While the framework provides many features, effective error handling is critical to ensuring that integrations are not only functional but also resilient and maintainable. In this blog post, we will explore various strategies for error handling in Spring Integration, complete with code snippets and best practices.
Why is Error Handling Important?
Errors are inevitable when working with integrations. Network issues, data inconsistencies, and service outages can occur, resulting in broken workflows and frustrated users. Therefore, having a solid error handling strategy is essential for maintaining system stability and providing a good user experience.
When properly designed, error handling can:
- Capture errors and log them for troubleshooting.
- Provide mechanisms for retrying operations.
- Forward errors to appropriate endpoints for monitoring or alerting.
- Ensure that messages do not get lost in transit.
Key Concepts in Spring Integration Error Handling
Before we dive into code and implementations, let's discuss some key concepts relevant to error handling in Spring Integration.
1. Error Channel
Spring Integration provides a default error channel, errorChannel
, which is a message channel used to handle error messages. By default, when an error occurs, the associated message is sent to this channel, where you can configure how to deal with the error.
2. Error Handlers
An error handler can be implemented to customize how errors are processed. You can define the behavior for errors based on various conditions, such as message type or the exception type that occurred.
3. Message Retries
Retry mechanisms are essential for scenarios where temporary issues can be resolved by re-attempting the operation. Spring Integration allows you to configure retry policies easily.
Error Handling Implementations
Let's explore some practical examples of how to implement error handling in Spring Integration.
Basic Error Handling with the Default Error Channel
Here’s a simple integration flow that handles errors using the default error channel.
<int:channel id="inputChannel"/>
<int:channel id="errorChannel"/>
<int:service-activator input-channel="inputChannel" ref="myService" method="processMessage" reply-channel="null" />
<int:service-activator input-channel="errorChannel" ref="errorHandler" method="handleError"/>
Explanation
- inputChannel: The main input channel where messages are sent.
- errorChannel: The channel that the framework will use to send messages when an error occurs.
- myService: A service bean that processes messages.
- errorHandler: This bean provides a method that will handle the error messages.
Creating a Simple Error Handler
Let's create a simple error handler to log errors.
import org.springframework.messaging.Message;
import org.springframework.integration.annotation.ServiceActivator;
import org.springframework.stereotype.Component;
@Component
public class ErrorHandler {
@ServiceActivator
public void handleError(Message<?> message) {
System.err.println("Error received: " + message.getPayload());
}
}
Why Use a Custom Error Handler?
By creating a custom error handler, you can manage the error logging and take actions based on the type of error. You can also choose to send alerts or notifications based on the errors encountered.
Configuring Retry Logic
Sometimes you may want to attempt to process a message multiple times before giving up. Spring Integration supports this through retry template configurations.
<int:service-activator input-channel="inputChannel" ref="myService" method="processMessage"
reply-channel="null" advice="retryAdvice"/>
<int:bean id="retryAdvice" class="org.springframework.integration.handler.advice.RequestHandlerRetryAdvice">
<property name="retryTemplate" ref="retryTemplate"/>
</int:bean>
<bean id="retryTemplate" class="org.springframework.retry.support.RetryTemplate">
<property name="backOffPolicy">
<bean class="org.springframework.retry.backoff.FixedBackOffPolicy">
<property name="backOffPeriod" value="5000"/> <!-- Retry every 5 seconds -->
</bean>
</property>
</bean>
Explanation
- RequestHandlerRetryAdvice: This class provides capabilities for retrying message processing operations.
- RetryTemplate: Here, we configure a retry template with a fixed back-off policy. It specifies that if an error occurs, the operation will be retried every 5 seconds.
Exception Handling
Sometimes, may want to handle specific types of exceptions differently. You can define a method for catching these exceptions and applying further logic.
import org.springframework.messaging.Message;
import org.springframework.messaging.MessageHandlingException;
import org.springframework.integration.annotation.ServiceActivator;
import org.springframework.stereotype.Component;
@Component
public class CustomErrorHandler {
@ServiceActivator(inputChannel = "errorChannel")
public void handleError(Message<?> message) {
Throwable cause = ((MessageHandlingException) message.getPayload()).getCause();
if (cause instanceof SomeSpecificException) {
// Handle specific exception
} else {
// Handle general exceptions
}
}
}
Why Differentiate Exception Types?
Distinguishing between exception types allows you to tailor responses according to the context and severity of the errors. This leads to a more robust and user-friendly error handling process.
Understanding Error Channels in Detail
Using error channels effectively can make debugging easier and improve the reliability of your integration flows. You can also route errors to a monitoring or alerting service.
<int:service-activator input-channel="inputChannel" ref="myService" method="processMessage"
reply-channel="null" />
<int:service-activator input-channel="errorChannel" ref="dbErrorHandler" method="handleDbError" />
<int:service-activator input-channel="errorChannel" ref="alertService" method="sendAlert"/>
In Conclusion, Here is What Matters
Effective error handling in Spring Integration is not just about catching exceptions; it's about managing errors throughout your integration ecosystem. Taking a thoughtful approach to error handling can enhance your application’s robustness while ensuring a better user experience.
By applying the strategies and examples outlined in this article, you will be well on your way to mastering error handling in your Spring Integration projects. For further reading, you might find Spring Integration Documentation helpful.
Happy coding!
This blog post serves as a comprehensive introduction to mastering error handling in Spring Integration. You can adapt these snippets and strategies based on your specific requirements to achieve efficient error handling in your applications.