How to Effectively Log Root Causes of Exceptions
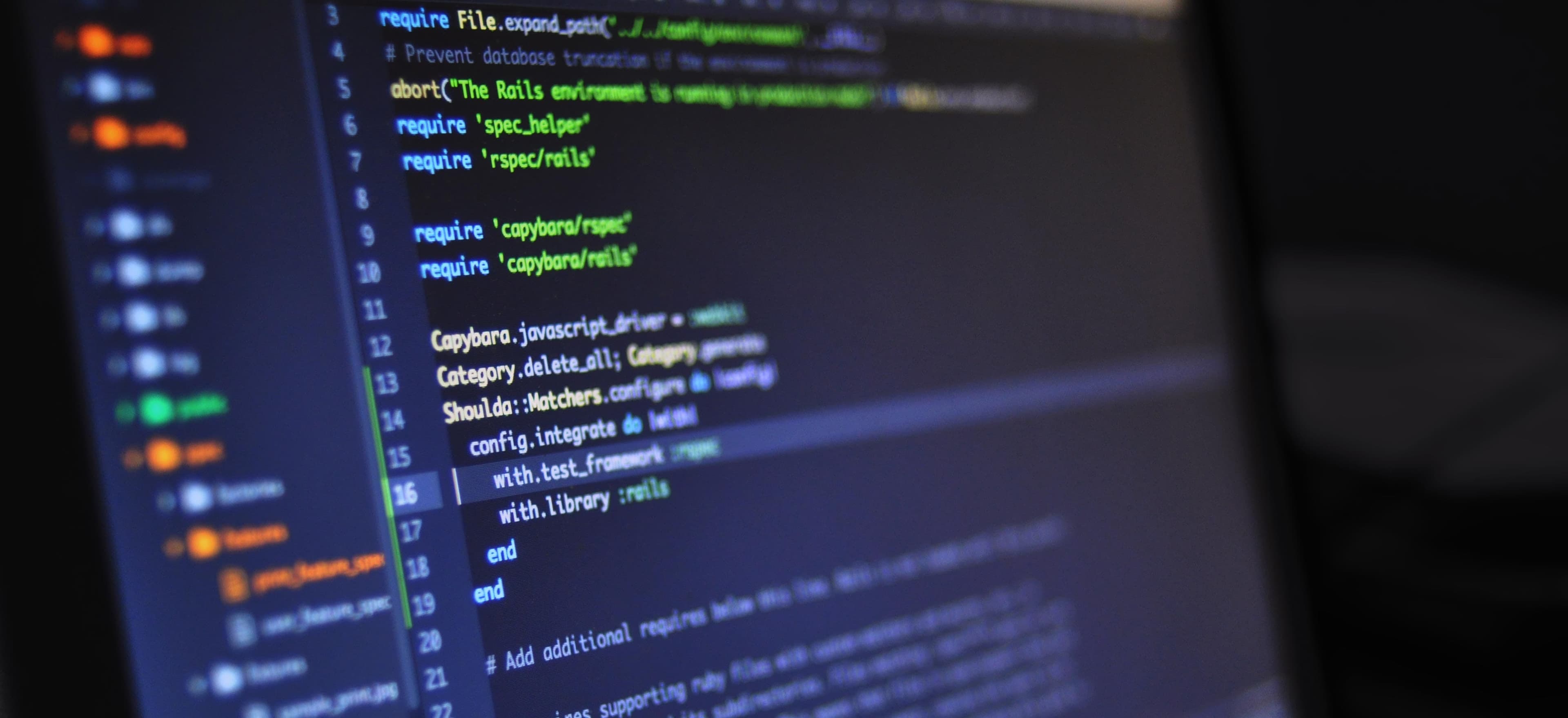
- Published on
How to Effectively Log Root Causes of Exceptions in Java
Logging exceptions in Java is a fundamental aspect of software development and maintenance. Every time a program runs into an issue, it can throw exceptions that disrupt the normal flow of the application. Understanding how to effectively log these exceptions not only helps in troubleshooting but also aids in maintaining a robust application.
In this post, we will explore strategies for logging exceptions, emphasizing the importance of capturing not just the exception but also its root cause. We’ll discuss best practices and provide code snippets that exemplify key concepts.
Understanding Exceptions in Java
Before diving into logging, let us first clarify what exceptions are in Java. Exceptions are events that occur during the execution of a program that disrupts the normal flow. They can be checked (checked exceptions) or unchecked (runtime exceptions).
Common Java Exceptions
- NullPointerException: This occurs when an application tries to use
null
in a case where an object is required. - ArrayIndexOutOfBoundsException: Thrown when trying to access an illegal index in an array.
- SQLException: Related to database access issues.
- IOException: Issues in input-output operations.
Each exception carries essential information that can be pivotal in debugging.
The Importance of Logging Exceptions
Proper logging provides insight into errors that may not be immediately apparent during development. A well-structured log can serve as a documentation trail to understand the state of your application.
Benefits of Effective Logging
- Debugging: Helps to trace back to the exact point where the error occurred.
- Performance Monitoring: Understanding the frequency and context of exceptions can illuminate performance bottlenecks.
- Audit Trails: Logs can serve as an audit trail to track application behavior over time.
Best Practices for Logging Exceptions
1. Use a Logging Framework
Java offers several logging frameworks to handle logging efficiently. Popular options include:
- Log4j
- SLF4J (Simple Logging Facade for Java)
- java.util.logging
Using a professional logging framework allows for more control, such as logging levels, message formatting, and output destinations.
Example: Setting up Log4j
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class LoggingExample {
private static final Logger logger = LogManager.getLogger(LoggingExample.class);
public void divide(int numerator, int denominator) {
try {
int result = numerator / denominator;
} catch (ArithmeticException e) {
logger.error("Error occurred while dividing numerator by denominator. " +
"Numerator: {}, Denominator: {}. Error: {}",
numerator, denominator, e.getMessage());
}
}
}
In the above example, we effectively log an arithmetic exception, capturing the numerator and denominator to provide context.
2. Capture the Full Stack Trace
When an exception occurs, capturing the stack trace is crucial. The stack trace provides a detailed report of the active stack frames at a certain point in time. This provides clues to the root cause of the exception.
Example: Logging Full Stack Trace
public void readFile(String fileName) {
try {
BufferedReader reader = new BufferedReader(new FileReader(fileName));
// Further file operations
} catch (FileNotFoundException e) {
logger.error("File not found: {}", fileName, e);
}
}
Here, we pass the exception (e
) to the logger. The logging framework will handle the stack trace for us. This ensures we have vital context available during debugging.
3. Log Context Information
Capturing additional context about the application state can significantly enhance the utility of logs. Log data like user IDs, session data, or function parameters provide critical insight when reviewing log files post-mortem.
Example: Adding Context
public void authenticateUser(String username, String password) {
try {
// Logic for user authentication
} catch (AuthenticationException e) {
logger.error("Authentication failed for user: {}. Error: {}", username, e.getMessage());
}
}
Including the username provides a direct way to correlate the log entry with a specific user.
4. Use Custom Exceptions
Creating custom exceptions can provide more clarity to your logging. Custom exceptions can encapsulate more context and be more descriptive.
Example: Custom Exception
public class DatabaseConnectionException extends Exception {
public DatabaseConnectionException(String message) {
super(message);
}
}
public void connectToDatabase() throws DatabaseConnectionException {
try {
// Database connection logic
} catch (SQLException e) {
throw new DatabaseConnectionException("Database connection failed: " + e.getMessage());
}
}
In this case, if a DatabaseConnectionException
is thrown, you can log it with a specific message tailored to your application’s needs.
5. Log Severity Levels
Use different logging levels (INFO, DEBUG, ERROR, FATAL) according to the severity of the exception. This allows for more filtered and readable logs.
Example: Using Logging Levels
public void processTransaction(Transaction transaction) {
logger.info("Processing transaction: {}", transaction.getId());
try {
// Transaction processing logic
logger.debug("Transaction details: {}", transaction);
} catch (TransactionException e) {
logger.error("Transaction processing failed for Transaction ID: {}. Error: {}", transaction.getId(), e.getMessage());
}
}
In this example, we're using INFO to log a successful transaction initiation and DEBUG to capture the transaction's details. If an error occurs, we log that with a higher severity level (ERROR).
To Wrap Things Up
Effective logging of root causes for exceptions in Java is a practice that can save countless hours during application debugging and maintenance. Utilizing a robust logging framework, capturing full stack traces, logging contextual information, creating custom exceptions, and appropriately using logging levels, are all best practices that can lead to a more resilient and easily maintainable application.
Incorporating these strategies into your Java applications will allow you to resolve issues promptly and enhance overall code quality. For further insights into Java logging and best practices, consider reviewing additional resources, such as the official Log4j documentation and the Java Exception Handling documentation.
Remember, effective logging is not just about recording errors, but about building a foundation for continuous improvement within your software lifecycle. Happy coding!