Why Blocking Calls Can Break Your Reactor-Based App
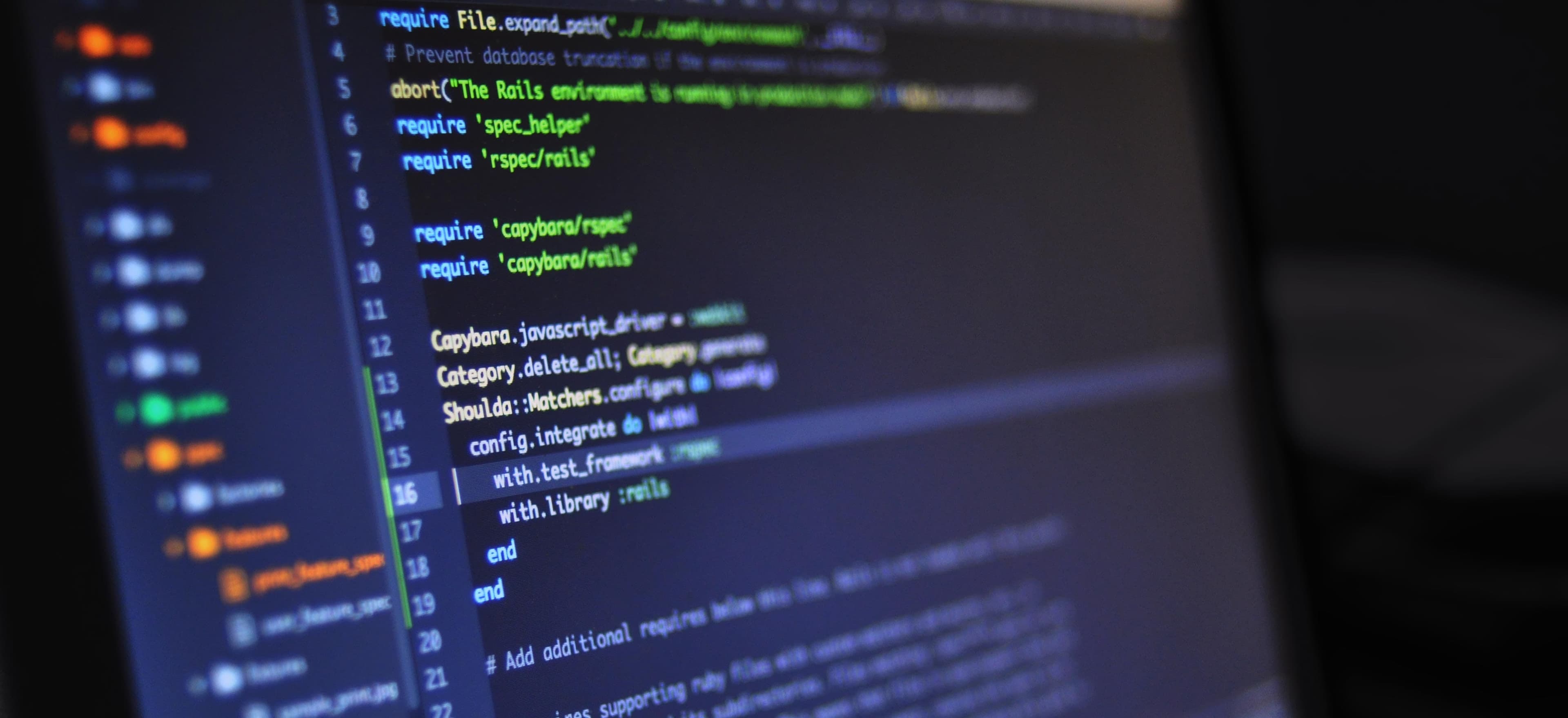
- Published on
Why Blocking Calls Can Break Your Reactor-Based App
In the world of concurrent programming, blocking calls can wreak havoc, especially in reactor-based applications. The reactor pattern is designed to handle multiple tasks effectively by processing events in a non-blocking manner. Understanding the implications of blocking calls is crucial for developers who want to maintain the responsiveness and performance of their applications.
In this blog post, we will delve into:
- What reactor-based applications are
- The characteristics of blocking calls
- How blocking calls disrupt event loops
- Best practices to avoid blocking calls
- Practical examples
Understanding Reactor-Based Applications
Reactor-based applications focus on asynchronous processing where events are handled in an event loop. This architecture allows the application to handle numerous requests simultaneously without dedicating a thread for each one.
For instance, popular frameworks like Node.js and Vert.x rely on this event-driven model to manage input/output operations. A single-threaded event loop waits for events and dispatches them to the associated handlers, enabling efficient resource usage.
Here's a simplified illustration of how reactor-based architecture works:
// Pseudocode for Reactor-based Application
EventLoop eventLoop = new EventLoop();
eventLoop.on("dataReceived", data -> {
processData(data);
});
// Starting the event loop
eventLoop.start();
Why Sympathetically Asynchronous?
Reactor-based applications thrive on non-blocking methods. The primary benefit of this model is throughput; it can manage thousands of connections without the overhead of traditional threading. With non-blocking I/O, the application can perform background tasks without blocking the main thread, allowing it to remain responsive to incoming events.
The Problem with Blocking Calls
What Are Blocking Calls?
Blocking calls are operations that halt the progress of a thread until a particular condition is met or a resource becomes available. Common examples include:
- Network I/O operations, such as waiting for data to be fetched from a remote server
- Long-running computations
- File I/O operations
When a blocking operation is invoked, it prevents other tasks in the event loop from executing. In a reactor-based application, this can lead to delayed responses, degraded user experience, and in severe cases, application failure.
The Event Loop Disruption
Consider the potential disruption within an event loop when a blocking call occurs. While processing an incoming event, if a blocking operation is invoked, the event loop is halted until that operation completes. This not only affects the current user's request but also all other requests queued in the system.
Here’s a rough example of blocking versus non-blocking calls in a Java-like pseudo code:
// Blocking call example
void fetchDataBlocking() {
// This call blocks the entire thread until data is fetched
String data = fetchFromServiceBlocking();
processData(data);
}
// Non-blocking call example
void fetchDataNonBlocking() {
fetchFromServiceNonBlocking(data -> {
processData(data);
});
}
In the blocking example, if fetchFromServiceBlocking
takes a long time, the whole application would freeze, which is unacceptable in modern responsive design.
Best Practices to Avoid Blocking Calls
-
Use Asynchronous APIs: Always prefer non-blocking versions of libraries/APIs. In Java, frameworks like Spring WebFlux and RxJava provide excellent support for asynchronous programming.
-
Leverage Multi-threading with Care: If you need to perform a blocking operation, consider moving it to a separate thread pool, keeping it isolated from the main event loop.
CompletableFuture.runAsync(() -> { String data = blockingOperation(); processData(data); });
-
Use Reactive Programming: Embrace reactive programming paradigms that emphasize a non-blocking flow of data. Libraries such as Project Reactor or RxJava provide rich tools for building reactive applications.
-
Monitor Performance: Implement logging and monitoring tools to track the responsiveness of your application and watch for any signs of blocking.
-
Implement Backpressure: When dealing with a high influx of requests, backpressure allows your application to manage load effectively without overwhelming the system.
Real-World Example
Let’s consider a simplified asynchronous web server using Java and Spring WebFlux.
@RestController
public class ReactiveController {
@GetMapping("/data")
public Mono<ResponseEntity<String>> getData() {
return fetchDataNonBlocking()
.map(data -> ResponseEntity.ok(data))
.defaultIfEmpty(ResponseEntity.notFound().build());
}
private Mono<String> fetchDataNonBlocking() {
return Mono.fromCallable(() -> {
// This simulates a blocking call (do not do this!)
return blockingService.call();
}).subscribeOn(Schedulers.boundedElastic());
}
}
In the example above, the fetchDataNonBlocking
method mimics a blocking operation but does so within a reactive context using Schedulers.boundedElastic()
. This allows the call to occur in its own thread, avoiding a freeze in the event loop.
To Wrap Things Up
Blocking calls have the potential to harm the very essence of reactor-based applications. By keeping operations non-blocking, you maintain system responsiveness, enabling your applications to scale seamlessly under demanding loads. As developers, it's our responsibility to adhere to best practices, ensuring that our applications are not only functional but also performant.
For further reading on asynchronous programming concepts, you might find Java Concurrency in Practice helpful. And for a deeper dive on reactive programming, check out the official Spring WebFlux documentation.
Adopting a non-blocking mindset will not only improve the performance of your applications but will also enhance user experience, making your systems robust and effective. Happy coding!