Mastering Single Responsibility: Avoiding Code Chaos
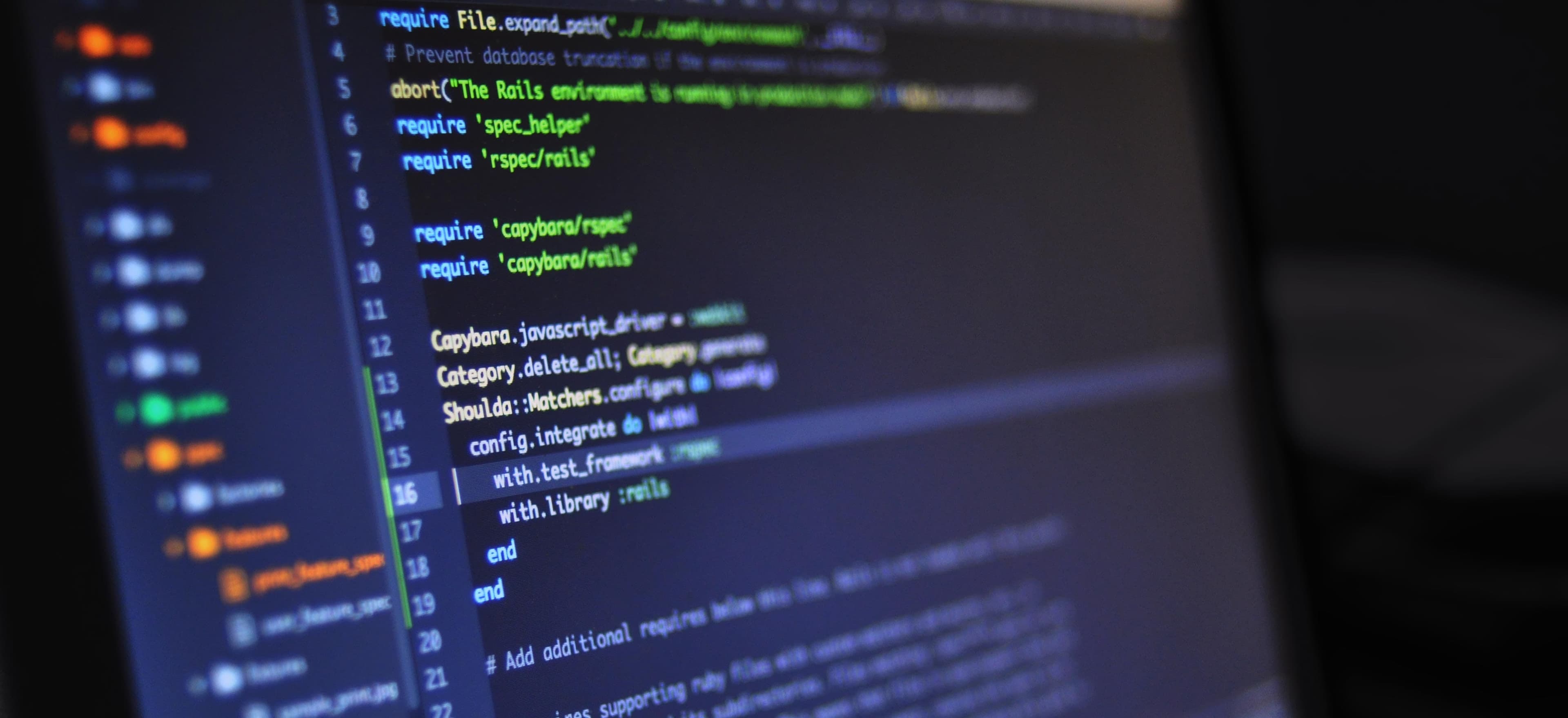
- Published on
Mastering Single Responsibility: Avoiding Code Chaos
In today’s fast-paced world of software development, maintaining clean, manageable, and scalable code is imperative. One of the most effective ways to achieve this is by adhering to the Single Responsibility Principle (SRP), one of the five SOLID principles of object-oriented programming. Understanding and implementing SRP can help you avoid code chaos and promote maintainability.
In this blog post, we will explore what the Single Responsibility Principle is, its significance, practical code examples, and ways to apply it effectively in your Java projects.
What is the Single Responsibility Principle?
The Single Responsibility Principle states that a class should have only one reason to change. In other words, each class should encapsulate a single concept or functionality. When this principle is applied correctly, it leads to a clearer structure, easier testing, and improved maintainability of the code.
Why Does SRP Matter?
- Easier Testing: With fewer responsibilities, a class becomes easier to test, since you can isolate its functionality without interference from other features.
- Improved Readability: Classes with a single responsibility are typically smaller and more focused, making them easier for developers to read and understand.
- Enhanced Maintainability: Changes in requirements often lead to code modifications. When a class is well-defined, modifications are less likely to introduce bugs in unrelated functionalities.
- Reusability: Classes focused on one task can be reused in different contexts, leading to less duplication and reduced risk of errors.
Applying SRP in Java: Code Examples
Let’s take a practical look into how to implement the Single Responsibility Principle in Java.
Bad Example: Violating SRP
Consider a simple class that handles user management along with sending emails when a user registers.
public class UserManager {
public void createUser(String username, String email) {
// Code to create user
System.out.println("User created: " + username);
sendEmail(email);
}
public void sendEmail(String email) {
// Code to send email
System.out.println("Email sent to: " + email);
}
}
In the above code, UserManager
has two responsibilities: managing user data and sending emails. If a requirement changes—say, the method for sending emails changes—the UserManager
class needs to be modified. This can lead to potential bugs or unintentional side effects.
Good Example: Complying with SRP
Instead, we can separate these functionalities into different classes following the SRP.
public class User {
private String username;
private String email;
public User(String username, String email) {
this.username = username;
this.email = email;
}
// Getters and other methods related to User
}
public class UserManager {
public void createUser(String username, String email) {
// Code to create user
User user = new User(username, email);
System.out.println("User created: " + username);
}
}
public class EmailService {
public void sendEmail(String email) {
// Code to send email
System.out.println("Email sent to: " + email);
}
}
Commentary on the Good Example
User
Class: Responsible solely for user data. It is encapsulated, which makes it reusable and maintainable.UserManager
Class: Handles user creation, but does not concern itself with the details of sending an email.EmailService
Class: Focuses entirely on email functionalities.
By structuring our code this way, we can easily modify or replace the EmailService
without impacting the user management functionality. This encapsulation fosters maintainability.
Real World Application: Dependency Injection
To further enhance the implementation of SRP, consider using Dependency Injection (DI) to decouple your services and enhance testability.
public class UserManager {
private final EmailService emailService;
public UserManager(EmailService emailService) {
this.emailService = emailService;
}
public void createUser(String username, String email) {
User user = new User(username, email);
System.out.println("User created: " + username);
emailService.sendEmail(email);
}
}
Why Use Dependency Injection?
- Decoupling: The
UserManager
class does not need to know how to send an email. It simply receives an instance ofEmailService
, promoting separation of concerns. - Flexible Testing: You can easily create a mock of
EmailService
during unit tests to verifyUserManager
functionality without needing to verify email sending logic.
Refactoring Existing Code for SRP
If you find yourself working with legacy code that violates SRP, here are steps you can take to refactor:
- Identify Responsibilities: Assess classes and methods to determine if they encompass multiple responsibilities.
- Decouple: Create new classes for distinct concerns and segregate responsibilities accordingly.
- Test: Ensure that proper unit tests are in place to maintain functionality during the transition.
- Iterate: Refactor in small, manageable increments rather than attempting to change everything at once.
This iterative process encourages sustainable development without overwhelming the development team.
The Bottom Line: The Road to Clean Code
Adhering to the Single Responsibility Principle is not merely a programming guideline; it is a necessary approach to write maintainable, scalable, and robust Java code. By ensuring that each class has a singular focus, developers can create a more organized codebase.
Are you ready to apply SRP in your Java projects? Start by examining your current code for violations and implement the changes outlined here. Remember, in the world of software development, simplicity is key to productivity, and SRP is your trusted guide.
For further reading on SOLID principles and best coding practices in Java, you may find the following links helpful:
- The SOLID Principles in Java
- Refactoring Techniques for Java Programs
Embrace SRP, and unlock a new level of code quality in your projects!