Common Pitfalls in Software Team Architecture Decisions
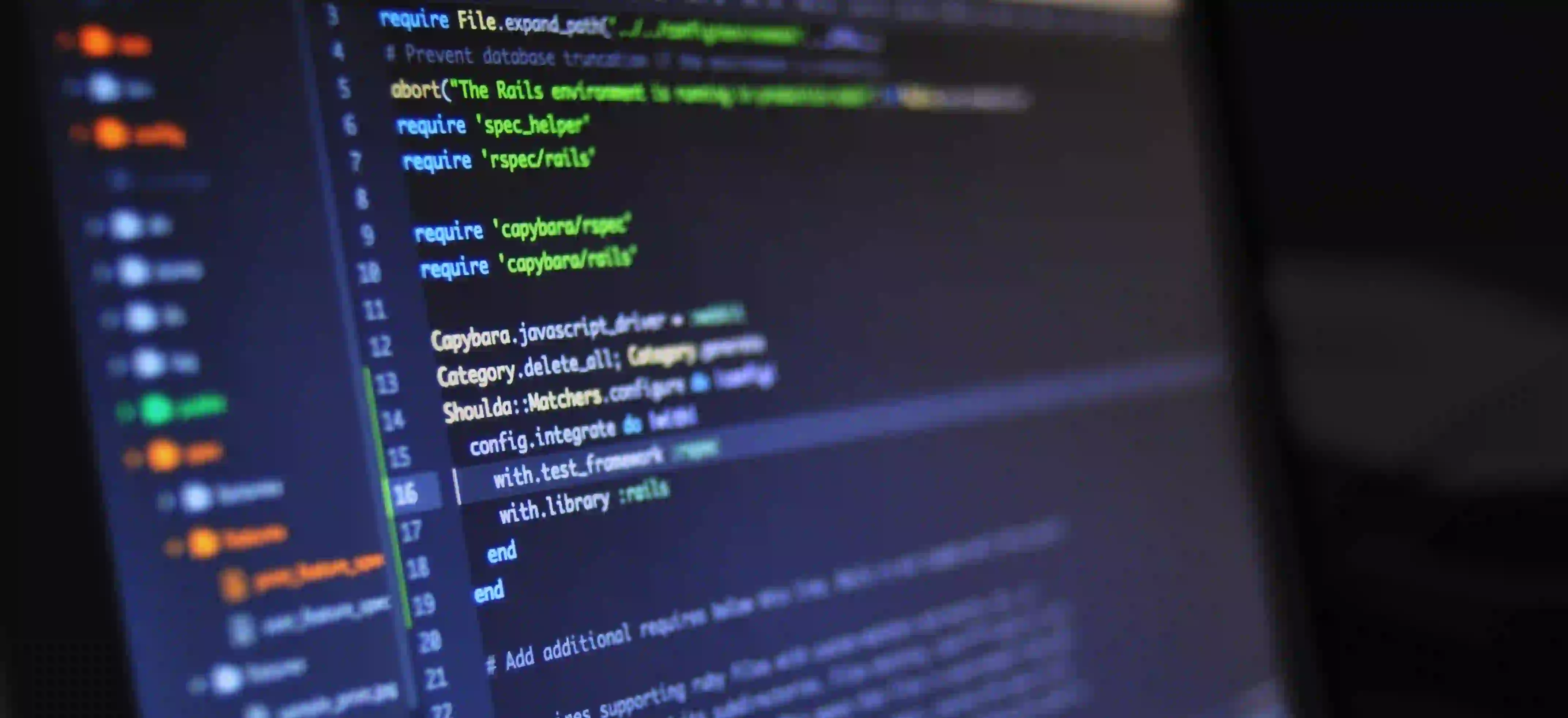
Common Pitfalls in Software Team Architecture Decisions
In the realm of software development, a strong architectural foundation is essential for building scalable, maintainable, and robust applications. However, architectural decisions can often lead teams astray if not approached judiciously. Let’s explore common pitfalls that software teams experience when making architectural decisions and how to avoid them.
1. Neglecting Requirements Analysis
One of the most significant pitfalls in architectural decisions is the failure to thoroughly analyze requirements. Rushing into a design without a comprehensive understanding of project needs can lead to misalignment between the architecture and the actual expectations of stakeholders.
Why It's Important
Failing to gather and analyze requirements can result in costly rework later. A clear understanding of functional and non-functional requirements ensures architects can make informed decisions.
Tips for Mitigating This Pitfall
- Engage stakeholders: Regular communication with stakeholders helps clarify expectations.
- Iterative feedback: Use an iterative approach to gather feedback and adapt the architecture accordingly.
2. Overengineering Solutions
Overengineering refers to designing a system that is more complex than necessary. While it might seem appealing to create a solution that can handle every possible future scenario, this often leads to unnecessary complexity and increases development time.
Example of Overengineering
Consider a simple user management system. If a team decides to build a microservices architecture to manage user profiles, user settings, and notifications without confirming the actual requirements, it can lead to excess overhead.
// Example of an overengineered approach with microservices for a simple task
// User Profile Service
public class UserProfileService {
public User getUserProfile(String userId) {
// retrieves user profile from database
}
}
// Notification Service
public class NotificationService {
public void sendNotification(String userId, String message) {
// sends notification to the user
}
}
// User Settings Service
public class UserSettingsService {
public UserSettings getUserSettings(String userId) {
// retrieves user settings from database
}
}
Simplified Alternative
Instead, a monolithic approach could be effective:
// Simplified user management using a single class
public class UserManager {
public User getUser(String userId) {
// retrieves user data, settings, and sends notifications as needed
}
}
Why This Matters
A simpler solution not only reduces development time but also eases maintenance and enhances team productivity. Always opt for the simplest architecture that meets the requirements without unnecessary complexity.
3. Ignoring Scalability and Performance
As applications grow, scalability becomes a crucial concern. Teams often overlook it in the early stages, leading to significant performance bottlenecks later on.
The Pitfall of Ignoring Scalability
Building an application with no performance considerations can result in a slow user experience as data volume grows. For instance, an application that is currently performing well can struggle when user counts double or triple.
How to Ensure Scalability
- Plan for growth: Anticipate how the application might need to scale and design accordingly.
- Load testing: Regularly conduct performance tests to identify bottlenecks.
4. Failing to Document Architecture Decisions
Clear documentation of architectural decisions is essential for current and future team members. Neglecting this can lead to confusion, miscommunication, and errors down the line.
Importance of Documentation
Documenting decisions keeps everyone on the same page. It explains the rationale behind certain choices and can be invaluable for onboarding new team members.
Good Practices
- Use diagrams: Visual representations can enhance understanding. Tools like Lucidchart or draw.io can aid in visualizing architecture.
- Maintain a changelog: Document changes to architectural decisions to provide context for future iterations.
5. Avoiding Technical Debt
Technical debt is often perceived as a necessary evil in software development. However, allowing technical debt to accumulate without appropriate refactoring can cripple projects in the long run.
Understanding Technical Debt
It's essential to strike a balance between delivering features quickly and maintaining code quality. Ignoring the need for refactoring leads to poor code maintainability and inflated costs.
Strategies to Manage Technical Debt
- Code reviews: Regular code reviews can help identify areas of technical debt.
- Scheduled refactoring: Include refactoring in your sprint planning to ensure ongoing code quality.
6. Not Involving the Entire Team
Architectural decisions should not be made in isolation. Neglecting to involve the entire team, especially those who will work directly with the code, can lead to incorrect assumptions and inadequate solutions.
Why Team Involvement is Crucial
A collaborative approach not only brings diverse perspectives but also ensures the solution is feasible for all team members.
Implementing Team Collaboration
- Regular architectural discussions: Hold regular meetings to discuss architectural concerns.
- Pair programming: Foster collaboration through pair programming to share knowledge and expertise.
A Final Look
Architectural decisions in software development can profoundly impact the success of a project. By avoiding these common pitfalls—neglecting requirements analysis, overengineering solutions, ignoring scalability, failing to document decisions, slacking on technical debt management, and excluding team involvement—software teams can create a more robust and adaptable system.
For further reading on software architecture principles, consider exploring Martin Fowler's "Patterns of Enterprise Application Architecture" or Nine Common Pitfalls in Software Architecture.
Make informed decisions, engage your team, and build an architecture that stands the test of time.