Overcoming Event Ordering Challenges in MongoDB Streams
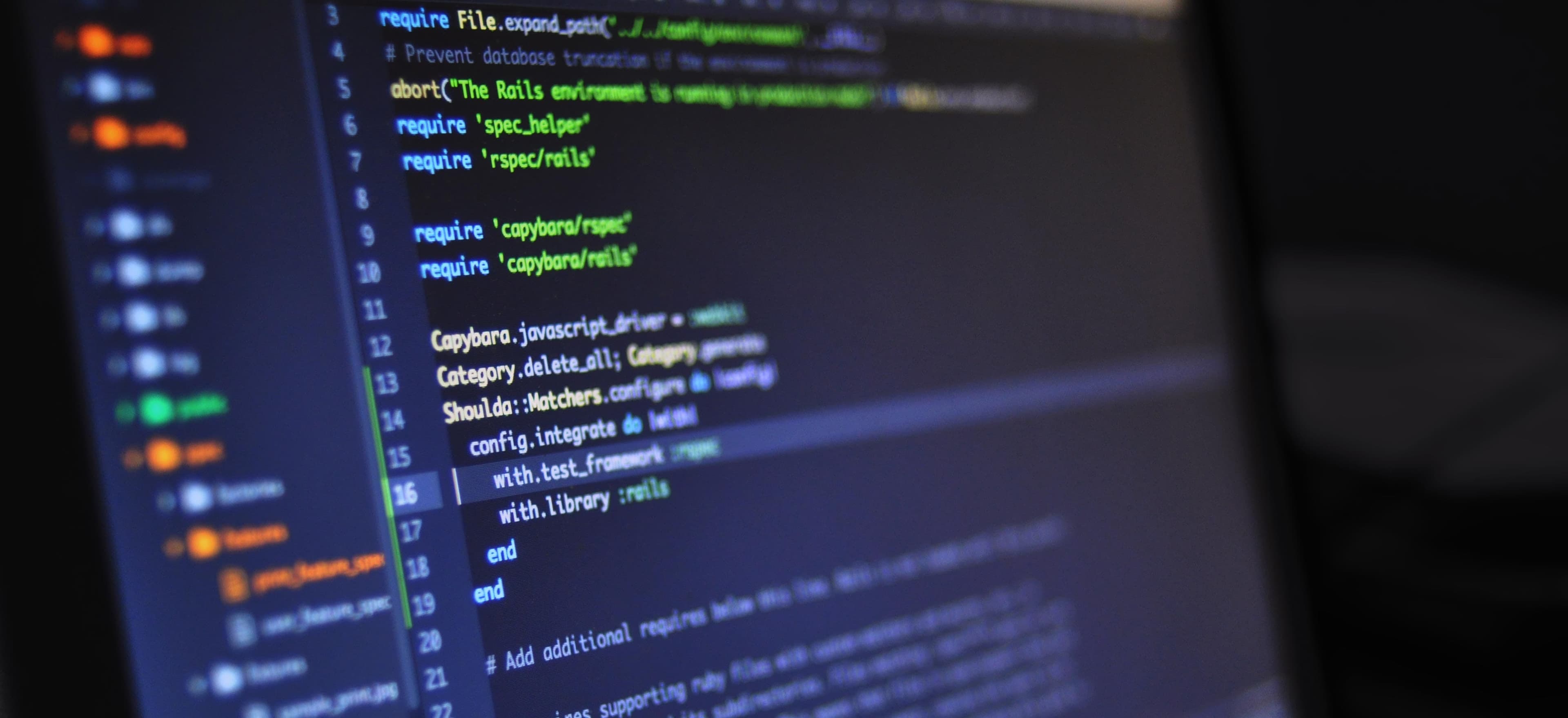
- Published on
Overcoming Event Ordering Challenges in MongoDB Streams
In today's world of real-time data processing, event ordering is a critical aspect. When dealing with streams of data, ensuring that events are processed in the correct sequence can significantly impact the integrity and accuracy of the information. MongoDB Streams presents unique challenges when it comes to maintaining order, especially in distributed systems. In this blog post, we'll explore the nature of these challenges and discuss effective strategies to overcome them.
Understanding MongoDB Streams
Before diving into the ordering challenges, let’s briefly understand what MongoDB Streams is. MongoDB Change Streams allow applications to access real-time data changes in your MongoDB collections. This is achieved without needing additional polling or complex workloads.
This capability is immensely powerful for applications requiring live updates, notifications about database changes, or real-time analytics. However, as enticing as this feature is, managing the order of events can sometimes be a hurdle.
The Importance of Event Ordering
When processing events, maintaining the correct order is crucial for several reasons:
- Data Consistency: Incorrectly ordered events can lead to inconsistent states in the application.
- Transactional Integrity: Events that influence each other need to be processed in sequence to prevent data corruption.
- User Experience: In user-facing applications, presenting events in the right order improves usability and trust.
Challenges with Event Ordering in MongoDB Streams
1. Concurrent Updates
In a highly concurrent environment, multiple updates to the same document can lead to race conditions. For instance, if two updates occur simultaneously, the results will depend on which update gets processed last.
Code Snippet: Handling Concurrent Updates
Here’s a MongoDB update operation using a Java driver that showcases how to handle concurrent updates using transaction behaviors.
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoDatabase;
import com.mongodb.client.MongoCollection;
import org.bson.Document;
import static com.mongodb.client.model.Filters.eq;
public class UpdateWithTransaction {
public static void main(String[] args) {
MongoClient mongoClient = MongoClients.create("mongodb://localhost:27017");
MongoDatabase database = mongoClient.getDatabase("test");
MongoCollection<Document> collection = database.getCollection("events");
try (var session = mongoClient.startSession()) {
session.startTransaction();
try {
collection.updateOne(session, eq("eventId", 1), new Document("$set", new Document("status", "processed")));
session.commitTransaction();
} catch (Exception e) {
session.abortTransaction();
System.out.println("Transaction aborted: " + e.getMessage());
}
}
}
}
Why This Matters: This code snippet ensures that the operation is atomic. If one operation fails, the changes are rolled back, preserving the integrity of the database. Thus, even in concurrent scenarios, you mitigate the risk of inconsistent states.
2. Out-of-Order Events from Microservices
When events are generated in a microservices architecture, it’s common for events to reach the MongoDB database out of order due to network latency or processing delays. One service may produce an event that is processed faster than another, leading to chaos in event handling.
3. Using Timestamps
One common approach to tackle event ordering issues is to use timestamps. By timestamping each event, you can sort the events based on their arrival times.
Code Snippet: Sorting Events Using Timestamps
Here’s a code example that retrieves events from the collection and sorts them using the timestamp.
import java.util.List;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoDatabase;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoCursor;
import org.bson.Document;
public class SortEventsByTimestamp {
public static void main(String[] args) {
MongoClient mongoClient = MongoClients.create("mongodb://localhost:27017");
MongoDatabase database = mongoClient.getDatabase("test");
MongoCollection<Document> collection = database.getCollection("events");
List<Document> events = collection.find()
.sort(new Document("timestamp", 1))
.into(new ArrayList<>());
for (Document event : events) {
System.out.println(event.toJson());
}
}
}
Why This Matters: By sorting events upon retrieval, this method ensures they are processed accurately based on when they occurred. However, this approach requires that the timestamps are correctly captured and consistent across services.
4. Using Sequence Numbers
Another effective method for ensuring order is to use sequence numbers. Each event can carry a sequence number, incremented with each new event. This number acts as a determinant for processing.
Code Snippet: Using Sequence Numbers
Here’s an example of how you might implement this.
public class Event {
private int sequenceNumber;
private String data;
public Event(int sequenceNumber, String data) {
this.sequenceNumber = sequenceNumber;
this.data = data;
}
public int getSequenceNumber() {
return sequenceNumber;
}
public String getData() {
return data;
}
}
Why This Matters: Each event created now carries information on its sequence. When processing events, you can easily ensure the correct order utilizing these numbers.
Strategies for Enhancing Ordering
1. Use a Buffer or Queue
For systems with heavy load or unpredictable event flows, consider implementing a buffer or queue to hold events temporarily. This will allow for processing events in a controlled manner.
2. Acknowledge Processed Events
Implementing an acknowledgment system ensures that once an event is processed, it is marked appropriately. This helps in tracking which events have been successfully handled.
3. Implement Event Sourcing
In event-sourced systems, the state of the application is determined by a series of events. By maintaining these events in a correct sequence, developers can rebuild the application state accurately.
Final Thoughts
The challenge of event ordering in MongoDB Streams is not insurmountable. By leveraging concurrent updates management, timestamps, sequence numbers, or even implementing buffering mechanisms, you can ensure your system processes events accurately and reliably.
For further information on MongoDB Change Streams and event processing strategies, check out these helpful resources.
Ultimately, as we build systems to handle real-time data, our approach towards event ordering will significantly define the systems' robustness and reliability. By tackling these challenges head-on, developers can ensure better performance and a smoother experience for end-users.