Avoiding Confusion: Mixing Abstractions in Clean Code
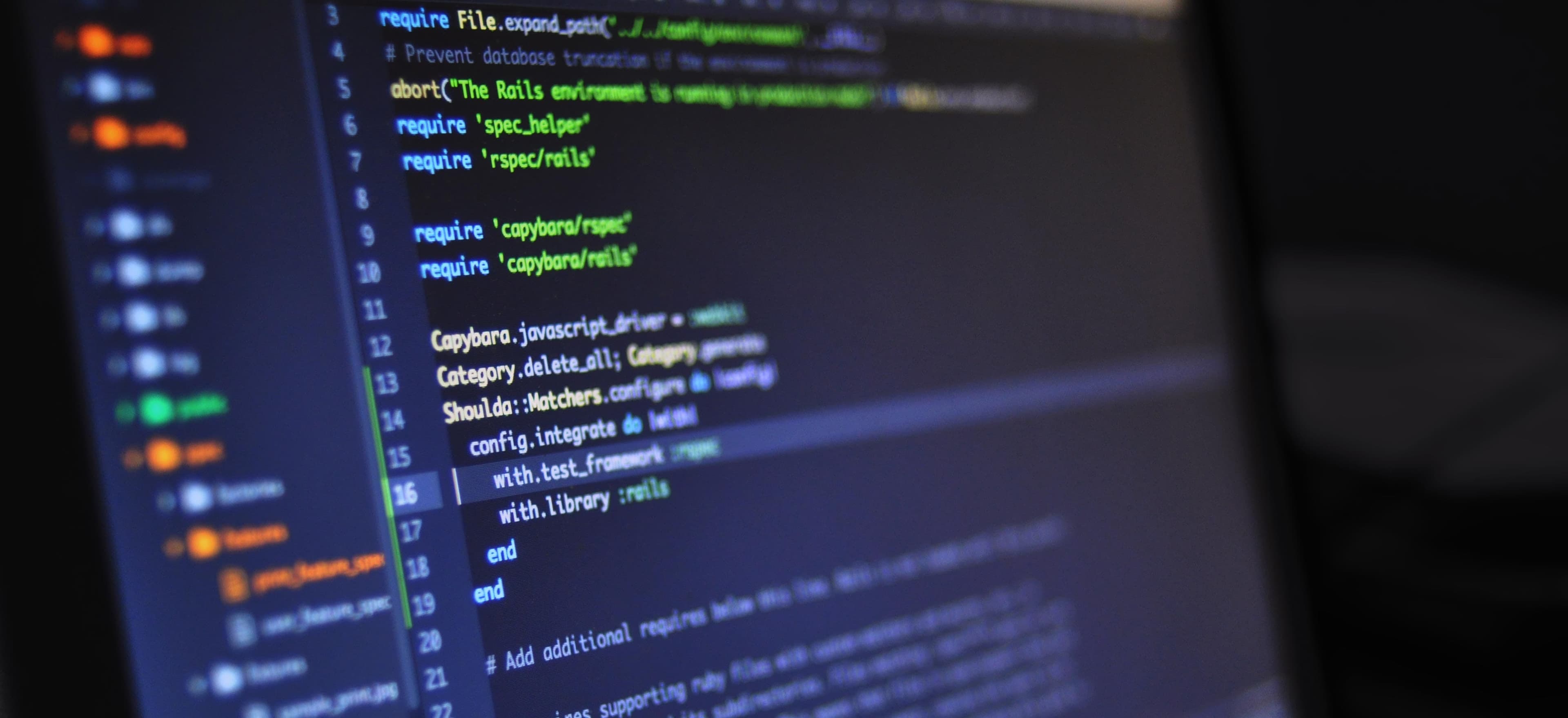
- Published on
Avoiding Confusion: Mixing Abstractions in Clean Code
In the world of software development, the concept of "clean code" is paramount. Understanding how to navigate through the myriad of abstractions available in programming languages like Java is essential for developers looking to write maintainable, readable, and efficient code. The idea of mixing abstractions can often lead to confusion, making it crucial to grasp the principles of clean coding practices.
In this blog post, we will explore what mixing abstractions means, why it can lead to issues in coding, and how to avoid these pitfalls while leveraging abstractions effectively in Java.
What Are Abstractions?
Abstraction is a fundamental principle in computer science that involves hiding the complex reality while exposing only the necessary parts. In Java, abstractions can be implemented through interfaces, abstract classes, and even through high-level constructs like APIs.
To illustrate, consider the following snippet that uses an interface to achieve abstraction:
public interface Animal {
void sound();
}
In this example, the Animal
interface defines a method sound
. This method doesn't specify what sound is made; it simply states that animals will have this behavior.
Why Is Mixing Abstractions a Problem?
Mixing abstractions can create code that is difficult to read, understand, and maintain. Here are a few reasons why:
-
Complexity: When developers use multiple layers of abstractions without clear separations, it often leads to complex systems that are tough to decipher.
-
Coupling: Tight coupling can occur when different abstractions depend on one another. Changes in one part of the code can lead to unexpected behaviors elsewhere.
-
Unpredictable Behavior: Mixing different types of abstractions can lead to assumptions in the code that might not hold true. For instance, expecting a certain behavior from an interface that wasn't designed for that purpose can introduce bugs.
-
Diminished Readability: When you mix abstractions, the readability of the code diminishes. Developers may spend time trying to understand what parts of the code belong where.
To further emphasize these themes, let's illustrate a scenario involving mixed abstractions.
A Scenario: Mixing Interfaces and Abstract Classes
Let’s consider a Java project where we have an abstract class Vehicle
and an interface Flyable
.
public abstract class Vehicle {
abstract void start();
abstract void stop();
}
public interface Flyable {
void fly();
}
Now, imagine we create a subclass of Vehicle
called Car
, and we erroneously implement the Flyable
interface:
public class Car extends Vehicle implements Flyable {
@Override
void start() {
System.out.println("Car is starting.");
}
@Override
void stop() {
System.out.println("Car is stopping.");
}
@Override
public void fly() {
// This method doesn’t make sense for a Car
throw new UnsupportedOperationException("Cars cannot fly!");
}
}
What Went Wrong?
In this example, mixing an abstract class with a functionality it does not support leads to confusion. Here are a few points to consider:
-
Unintended Behavior: By implementing the
Flyable
interface inCar
, we introduce an expectation that a car can fly, which is fundamentally untrue. This can lead to bugs if someone tries to call thefly()
method on a Car instance. -
Responsibility: The
fly()
method should be associated with vehicles that are actually capable of flying, such asPlane
orBird
classes. TheCar
class dilutes its responsibility by trying to adopt a behavior that does not apply.
Avoiding Mixing Abstractions
To avoid the confusion that comes from mixing abstractions, follow these guidelines:
-
Single Responsibility Principle: Each class or interface should have one reason to change. Ensure that your abstractions truly reflect single roles in the system.
-
Proper Hierarchy: Establish a clear hierarchy. If a class is inheriting from an abstract class or implementing an interface, ensure that it logically fits that abstraction.
-
Consistent Naming: Use clear and descriptive names that reflect the behavior and responsibilities of the class. This helps readers understand the purpose of each abstraction without digging through the code.
-
Explicit Contracts: Define interfaces with clear contracts that dictate their behavior. Any implementation should comply strictly with these contracts to avoid confusion.
-
Refactoring: Don’t hesitate to refactor your code. If you find that a class is taking on too many roles or that an abstraction is becoming ambiguous, it is essential to refactor for clarity.
Example: Properly Extending Functionality
Consider a better approach using clear responsibilities:
public class Plane extends Vehicle implements Flyable {
@Override
void start() {
System.out.println("Plane is starting.");
}
@Override
void stop() {
System.out.println("Plane is landing.");
}
@Override
public void fly() {
System.out.println("Plane is flying.");
}
}
In this example, the Plane
class properly inherits from the Vehicle
class and also knows how to fly. Each abstraction fits perfectly, following clear responsibilities without overlap.
In Conclusion, Here is What Matters
Incorporating clean coding practices while avoiding the confusion that arises from mixing abstractions can dramatically improve the readability, maintainability, and robustness of your Java applications. As developers, we need to remain conscious of how abstractions interact and avoid convoluted designs.
Emphasizing clarity, promoting single responsibility, and ensuring proper application of interfaces and abstract classes will lead to cleaner, more effective code bases.
For further reading on clean coding practices, consider checking out Uncle Bob's Clean Code and Martin Fowler on Refactoring.
Let’s move forward with a commitment to crafting clean, understandable, and efficient code in our Java projects!