The Hidden Costs of Using Capitalized Java Variables
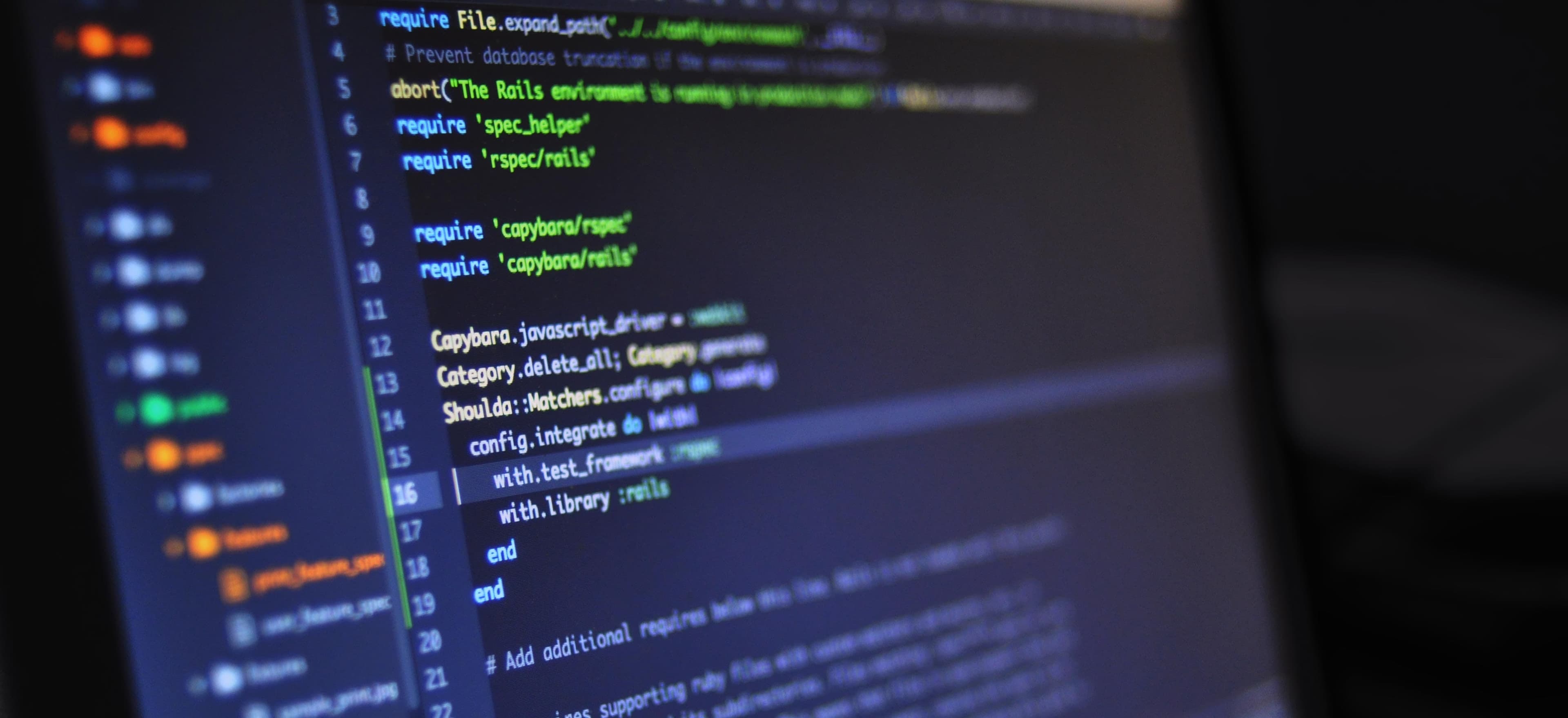
- Published on
The Hidden Costs of Using Capitalized Java Variables
In the Java programming world, naming conventions play a pivotal role in ensuring code clarity and maintainability. Among the various conventions, the usage of capitalized variables—specifically uppercase variables—can lead to both understandable naming choices and subtle yet significant pitfalls. In this blog post, we will explore the hidden costs associated with using capitalized Java variables, understand why these conventions are critical, and eventually guide you toward best practices in Java variable naming.
Understanding Capitalization in Java
In Java, certain naming conventions must be adhered to for consistency and clarity. The Java Language Specification suggests:
- Class Names: Should use PascalCase (e.g.,
MyClass
). - Instance Variables and Method Names: Should use camelCase (e.g.,
myInstanceVariable
,myMethod
). - Constants: Should be written in ALL_CAPS with underscores separating words (e.g.,
MAX_VALUE
).
While these conventions help developers understand the type and purpose of the variable at a glance, straying from these norms—particularly through the overuse of capitalized variables—can lead to unintended consequences.
The Hidden Costs of Using Capitalized Java Variables
1. Miscommunication Among Developers
When using capitalized variable names indiscriminately, your intention can become muddled. For example, take the following scenario:
public class Configuration {
public static final int MAX_RETRIES = 5;
public static final int MAX_RETRIES = 10; // duplicate declaration
}
In this case, the horizontal scalability of using capitalized constants might inadvertently allow duplicate declarations. The use of constant names should clearly denote their purpose.
Why is this important?
Using clear naming conventions helps all team members understand the role of a variable quickly, reducing the chances of errors and increasing the clarity of the codebase.
2. Overwriting Constants
Developers may accidentally overwrite constants due to a lack of clarity or misunderstanding of variable scope, particularly if they use all-caps for variables that aren't constants.
Consider the following snippet:
public class UserSettings {
public static final String USERNAME = "default_user";
public static String USERNAME = "admin_user"; // redefinition
}
By using capitalized variables for non-constants, it becomes seamless to confuse them with true constants. This can result in accidental overwrites, leading to bugs that are difficult to trace.
3. Lower Readability
Overuse of uppercase variables within a code body can lead to reduced readability, making it strenuous for developers to parse through code. Compare these two variable naming conventions:
public void updateSettings() {
final int MAX_ATTEMPTS = 3;
// Logic
}
public void updateSettings() {
final int maxAttempts = 3;
// Logic
}
The second example enhances clarity. Following consistent conventions, especially with case sensitivity, leads to easier readability and understanding across the board.
4. Enhanced Code Complexity
When dealing with large Java applications, complexity inevitably increases. Using capitalized variables excessively can lead to misunderstandings in the hierarchy and structure of variables, thus complicating an otherwise straightforward flow of logic:
public class Order {
public static final String PAGE_TITLE = "Order";
public static String page_title = "Home"; // conflicting naming conventions
}
Using inconsistent naming conventions like this can confuse team members and deliver a complicated codebase where maintaining and modifying code is tedious.
Best Practices for Java Variable Naming
1. Stick to Established Conventions
Adhering to Java naming conventions is vital for maintaining code quality. For constants, use ALL_CAPS format, whereas instance and method variables should be in camelCase. This ensures clarity for anyone reading the code.
2. Utilize Contextual Naming
Variable names should be descriptive. Instead of using vague names like MAX
or VALUE
, specify what it represents.
public static final int MAX_CONNECTION_RETRIES = 5;
This helps indicate what 'MAX' refers to.
3. Limit Scope Awareness
Understanding the scope of variables aids in maintaining the intended purpose. Constants should be static final and recognize it cannot be changed elsewhere or redeclared.
public static final String API_ENDPOINT = "https://api.example.com/v1/";
This clearly indicates its constant nature.
4. Refactor and Review
In large projects, regularly refactoring your code can help maintain quality. Engage in code reviews focusing on naming conventions to curb improper usage of capitalized variables.
5. Collaborative Communication
Encourage a culture of communication within the team about naming conventions. Share insights on possible pitfalls while using capitalized variables can save time, effort, and potential bugs later on.
Wrapping Up
Java capitalization conventions are not merely arbitrary. They are rooted in the pursuit of making code more understandable and maintainable. Misusing capitalized variables can lead to miscommunication, constant redefinitions, reduced readability, and unnecessary complexity. Always remember to apply best practices consistently—capitalize only when appropriate, provide clear descriptions, and keep the codebase readable.
For additional reading on Java naming conventions, take a look at Google's Java Style Guide and explore the Java Language Specification.
By adhering to naming conventions that prioritize clarity and intent, your codebase will become not only more robust but also easier to collaborate on, leading to a harmonious and effective programming environment.