Common Pitfalls in Spring MVC Controller Unit Testing
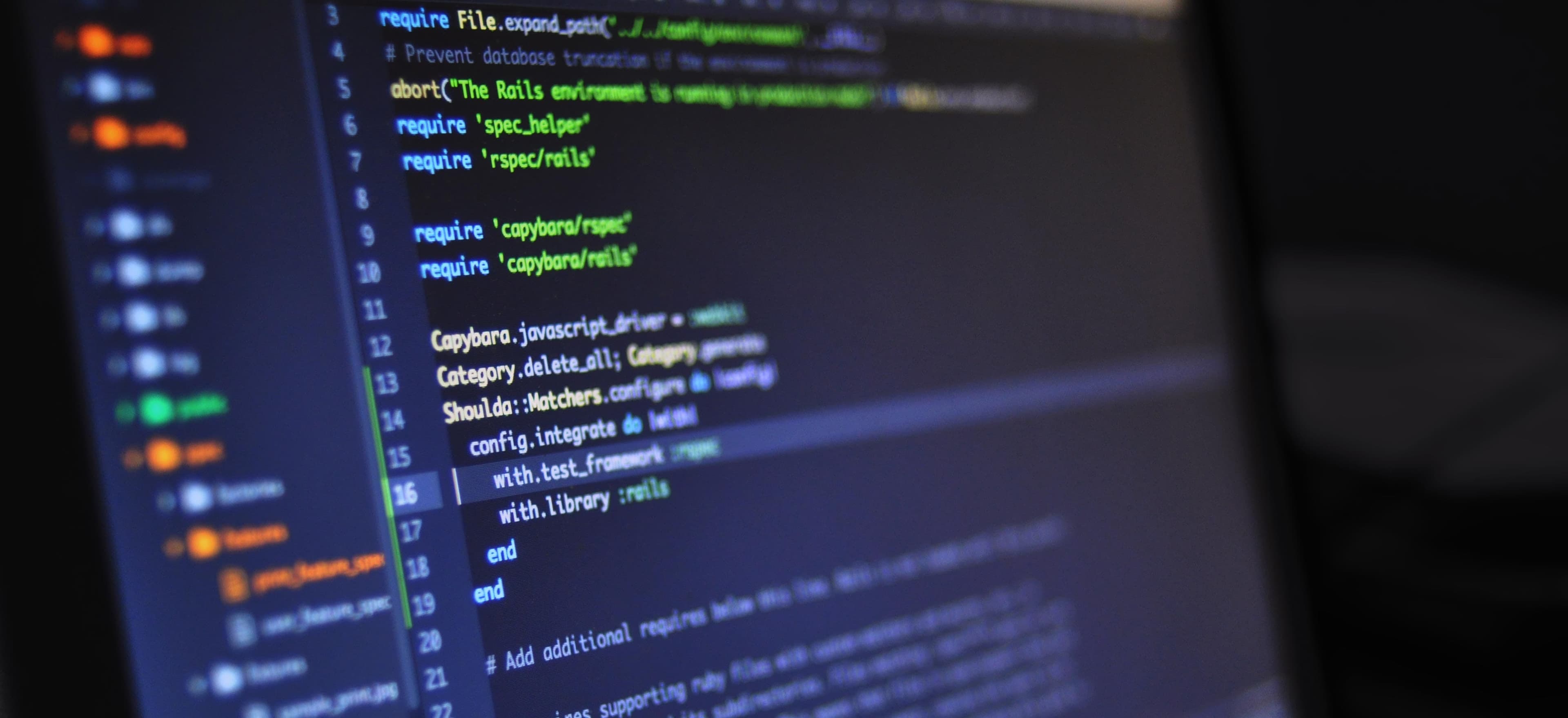
- Published on
Common Pitfalls in Spring MVC Controller Unit Testing
Unit testing is an essential aspect of software development that ensures the reliability of your application. However, when it comes to testing Spring MVC controllers, developers often encounter a series of common pitfalls. In this blog post, we'll delve into these pitfalls and provide actionable insights on how to avoid them.
Understanding Spring MVC Controllers
Before diving into testing pitfalls, let’s briefly discuss what Spring MVC controllers are. Spring MVC is a part of the Spring Framework, primarily used to develop web applications. A controller in Spring MVC serves as an intermediary between user input and the application’s logic.
The Importance of Unit Testing
Unit testing helps you:
- Detect bugs early: By isolating components and testing them individually.
- Facilitate changes: Refactoring code becomes easier, as you can run tests to ensure functionality remains intact.
- Improve design: Writing tests often leads to more modular code and better separation of concerns.
Now, let's look at some common pitfalls and how we can navigate them.
1. Not Isolating Dependencies
One of the most significant pitfalls in unit testing controllers is not isolating dependencies. A controller might depend on several services (like a UserService or ProductService). If you instantiate these services in your tests, you are not actually testing the controller in isolation.
Solution: Use mocking frameworks like Mockito to create mock objects for these dependencies. This allows you to focus purely on the controller's behavior.
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTest;
import org.springframework.test.web.servlet.MockMvc;
@WebMvcTest(UserController.class)
public class UserControllerTest {
@Autowired
private MockMvc mockMvc;
@MockBean
private UserService userService;
@Test
public void testGetUser() throws Exception {
when(userService.findUserById(1)).thenReturn(new User(1, "John Doe"));
mockMvc.perform(get("/users/1"))
.andExpect(status().isOk())
.andExpect(jsonPath("$.name").value("John Doe"));
}
}
In the above example, we use @MockBean
to create a mocked version of UserService
. This means we can control its behavior without involving the real implementation.
2. Overlooking Exception Handling
Another common oversight is neglecting to test how your controller handles exceptions. Exception handling is crucial in web applications for providing meaningful responses to users.
Solution: Utilize @ControllerAdvice
to handle exceptions globally and write unit tests that verify exception scenarios.
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(UserNotFoundException.class)
public ResponseEntity<String> handleUserNotFound(UserNotFoundException ex) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body(ex.getMessage());
}
}
To test how our controller reacts to exceptions, write a dedicated test case:
@Test
public void testGetUserNotFound() throws Exception {
when(userService.findUserById(1)).thenThrow(new UserNotFoundException("User not found"));
mockMvc.perform(get("/users/1"))
.andExpect(status().isNotFound())
.andExpect(content().string("User not found"));
}
3. Ignoring Response Validation
It is not enough to check just the HTTP status code. Many tests stop at verifying that an endpoint returns a successful response, but this is a significant oversight.
Solution: Always validate the response body and headers.
@Test
public void testCreateUser() throws Exception {
User newUser = new User(2, "Jane Doe");
when(userService.createUser(any())).thenReturn(newUser);
mockMvc.perform(post("/users")
.contentType(MediaType.APPLICATION_JSON)
.content("{\"name\":\"Jane Doe\"}"))
.andExpect(status().isCreated())
.andExpect(jsonPath("$.id").value(2))
.andExpect(header().string("Location", containsString("/users/2")));
}
4. Failing to Test All HTTP Methods
Many developers focus solely on GET requests and overlook other HTTP methods like POST, PUT, and DELETE. Each has its unique behavior and should be tested accordingly.
Solution: Structure your test class to include various HTTP methods for comprehensive coverage.
@Test
public void testUpdateUser() throws Exception {
User updatedUser = new User(1, "Updated Doe");
when(userService.updateUser(anyInt(), any())).thenReturn(updatedUser);
mockMvc.perform(put("/users/1")
.contentType(MediaType.APPLICATION_JSON)
.content("{\"name\":\"Updated Doe\"}"))
.andExpect(status().isOk())
.andExpect(jsonPath("$.name").value("Updated Doe"));
}
5. Not Testing Edge Cases
Neglecting edge cases can lead to unexpected behavior in production. While it’s essential to cover typical use cases, edge cases often reveal flaws in your logic.
Solution: Always think of corner cases when writing your tests. Consider scenarios with null inputs, empty strings, or massive data sizes.
@Test
public void testCreateUserWithEmptyName() throws Exception {
mockMvc.perform(post("/users")
.contentType(MediaType.APPLICATION_JSON)
.content("{\"name\":\"\"}"))
.andExpect(status().isBadRequest());
}
6. Non-Independence of Tests
Sometimes, testing code leads to interdependencies between test cases where one test influences another. This can create confusion and bugs that are tough to track down.
Solution: Ensure that each test method is independent. Use @BeforeEach
and @AfterEach
annotations to set up and tear down the testing context and data.
@BeforeEach
public void setUp() {
// Setup code here
}
@AfterEach
public void tearDown() {
// Cleanup code here
}
7. Not Utilizing Assertions Effectively
Lack of thorough assertions can lead to tests that pass without really verifying the expected behavior. Sometimes developers use only simple assertions, missing deeper components to validate.
Solution: Use dedicated assertion libraries like AssertJ or Hamcrest for more expressive assertions that yield clearer insights into failures.
import static org.assertj.core.api.Assertions.assertThat;
@Test
public void testGetUserResponse() throws Exception {
// Previously defined mock
MvcResult result = mockMvc.perform(get("/users/1"))
.andReturn();
String content = result.getResponse().getContentAsString();
assertThat(content).contains("John Doe");
}
Final Considerations
By recognizing these common pitfalls in Spring MVC controller unit testing, developers can significantly improve their code quality. Unit tests should provide confidence in your application’s functionality, ensuring that it behaves correctly under various conditions.
For more insights into Spring and Java development, feel free to check out Spring MVC Documentation and JUnit 5 User Guide for additional resources.
With these guidelines, you should be well-equipped to write effective and efficient unit tests for your Spring MVC controllers. Happy coding!