How to Securely Sandbox Java Code for Safe Execution
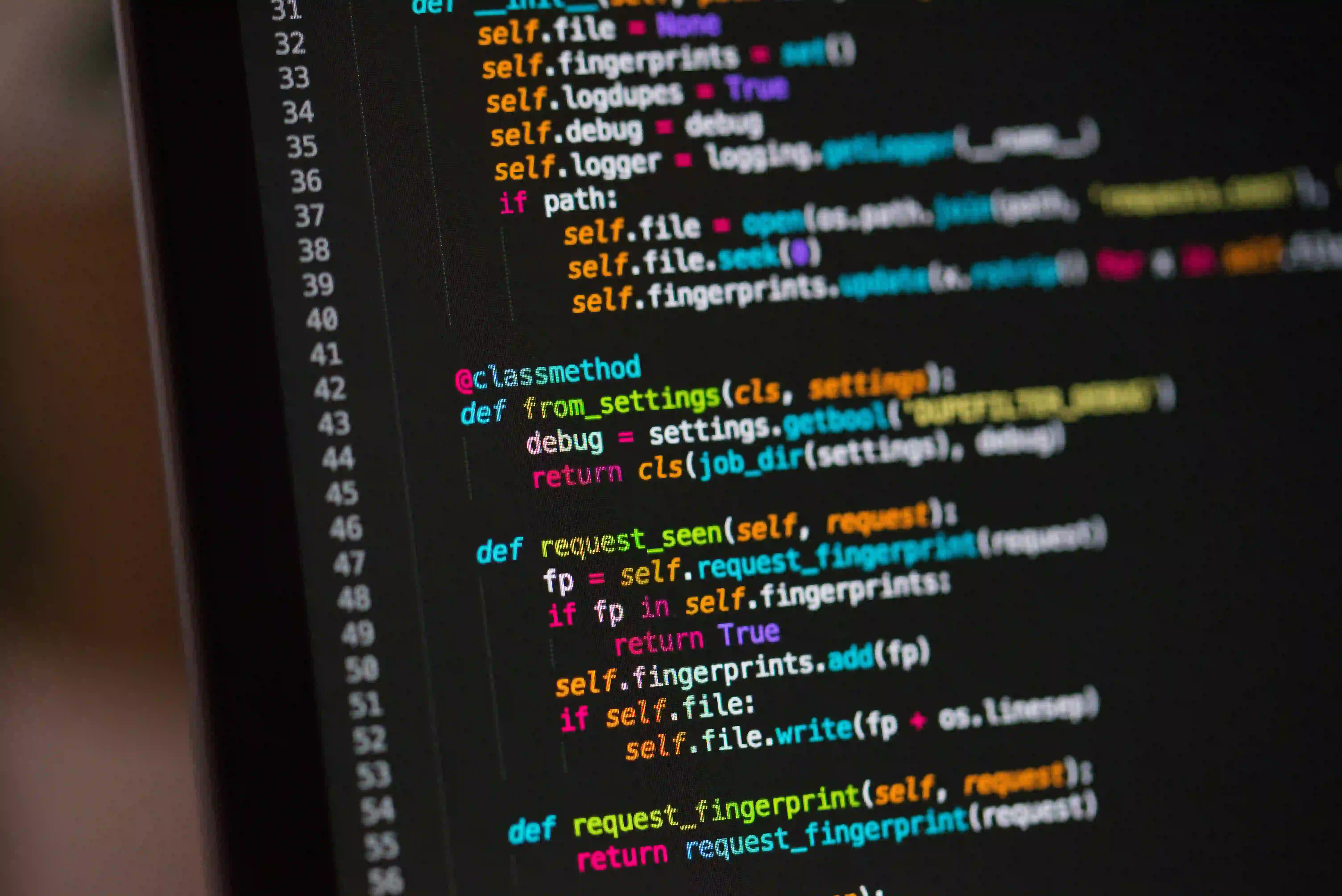
How to Securely Sandbox Java Code for Safe Execution
Java is widely popular for its versatility, performance, and extensive libraries. However, executing untrusted code can pose significant security risks. Therefore, properly sandboxing Java code becomes essential. This blog post will explore how to create a secure sandbox environment for running Java applications without compromising system integrity.
What is Code Sandbox?
A code sandbox is an isolated environment where code can be executed independently from the host system. This is particularly crucial when dealing with untrusted or third-party Java applications. The goal is to ensure that even if the running code is malicious or behaves unpredictably, it cannot affect the stability or security of the host system.
Why Sandbox Java Code?
- Security: Prevents unauthorized access to sensitive data and system resources.
- Stability: Isolates potentially buggy code, preventing crashes or errors in the host application.
- Testing: Allows you to test new libraries or frameworks without the risk of system corruption.
Now, let’s discuss effective strategies for securely sandboxing Java code.
1. Use the Java Security Manager
The Java Security Manager is a built-in feature designed to enforce security policies in Java applications. You can specify which permissions the code can access, and it does so by leveraging a security policy file.
Setting Up the Security Manager
To use the Security Manager, you need to define a security policy that outlines permitted actions. Below is an example of how to set up a simple security policy file.
- Create a file named
sandbox.policy
:
grant {
// Allow read access to the user's home directory
permission java.io.FilePermission "${user.home}/-", "read";
// Allow execution of limited system commands
permission java.lang.RuntimePermission "exec.*";
// Allow basic network access
permission java.net.SocketPermission "*", "connect,resolve";
// Deny all else
permission java.lang.RuntimePermission "exitVM.0";
permission java.lang.RuntimePermission "shutdownHooks";
};
Enabling the Security Manager
To enforce the policy, you can enable the Security Manager with the following command:
java -Djava.security.manager -Djava.security.policy=sandbox.policy YourApplication
Why Use the Security Manager?
Using the Security Manager allows fine-grained control over what the Java code can do, providing an extra layer of security. By defining what operations are safe, you significantly minimize the risks associated with executing untrusted code.
2. Leverage Java’s ClassLoader
The ClassLoader in Java helps you load classes at runtime and can be an effective way to isolate the executed code further. By creating a custom ClassLoader, you can ensure that the untrusted code does not have access to sensitive libraries or classes.
Creating a Custom ClassLoader
public class SandboxClassLoader extends ClassLoader {
@Override
protected Class<?> findClass(String name) throws ClassNotFoundException {
// Restrict class loading here
// Only allow loading classes from your predefined directory
// Example of loading a class
String filePath = "path/to/classes/" + name.replace('.', '/') + ".class";
byte[] classData = loadClassData(filePath);
return defineClass(name, classData, 0, classData.length);
}
private byte[] loadClassData(String filePath) {
// Implement your own logic to read the class file as bytes
// Use FileInputStream, BufferedInputStream here
}
}
Why Implement a Custom ClassLoader?
Using a custom ClassLoader ensures that untrusted code cannot load any classes that could potentially compromise the hosting environment. This control over the class loading mechanism is paramount for maintaining security.
3. Use Isolated Process with Restricted Resources
Running untrusted Java code in a separate process can effectively isolate it from the main application. This approach allows you to impose resource limits and manage the lifecycle securely.
Using Java ProcessBuilder
Java's ProcessBuilder API allows you to create and manage external processes easily.
ProcessBuilder processBuilder = new ProcessBuilder("java", "-Djava.security.manager", "YourUntrustedApp");
processBuilder.redirectErrorStream(true);
processBuilder.directory(new File("path/to/untrusted/code"));
try {
Process process = processBuilder.start();
// Monitor and impose limits to process resources
int exitCode = process.waitFor();
System.out.println("Exited with code: " + exitCode);
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
Why Use Isolated Processes?
Isolating untrusted code in a separate process not only enhances security but also simplifies resource management. You can set explicit limits on CPU and memory usage, ensuring that a rogue process cannot overwhelm system resources.
4. Utilize Docker Containers
Another effective method for sandboxing Java applications is by using Docker containers. Docker provides lightweight, portable, and secure environments for running applications.
Setting Up a Docker Container
Here's a simple Dockerfile to create a Java sandbox environment.
# Use an official Java runtime as a parent image
FROM openjdk:11-jre-slim
# Set the working directory
WORKDIR /app
# Copy application JAR files
COPY target/your-application.jar .
# Run the application
CMD ["java", "-Djava.security.manager", "-Djava.security.policy=/path/to/sandbox.policy", "your.package.MainClass"]
Running the Docker Container
To build and run the container:
docker build -t java-sandbox .
docker run java-sandbox
Why Use Docker Containers?
Using Docker allows for effective application isolation while providing portability. Docker containers can be easily deployed, replicate environments, and clean up after themselves when not needed.
Closing the Chapter
Securing and sandboxing Java code is an essential skill for developers, particularly in today's environment of growing cyber threats. By leveraging the Java Security Manager, custom ClassLoaders, isolated processes, and Docker, you can execute untrusted code safely and reliably.
For further information on secure Java programming, you may check out the official Java documentation at Oracle's Java Security or review best practices for secure coding at OWASP.
By utilizing some or all of these methods, you can provide a robust defense against potential security threats while maintaining the flexibility Java offers. The balance of code execution safety and maintaining application performance is achievable with careful implementation of these techniques.