Mastering Optimal String Alignment in Java: Common Pitfalls
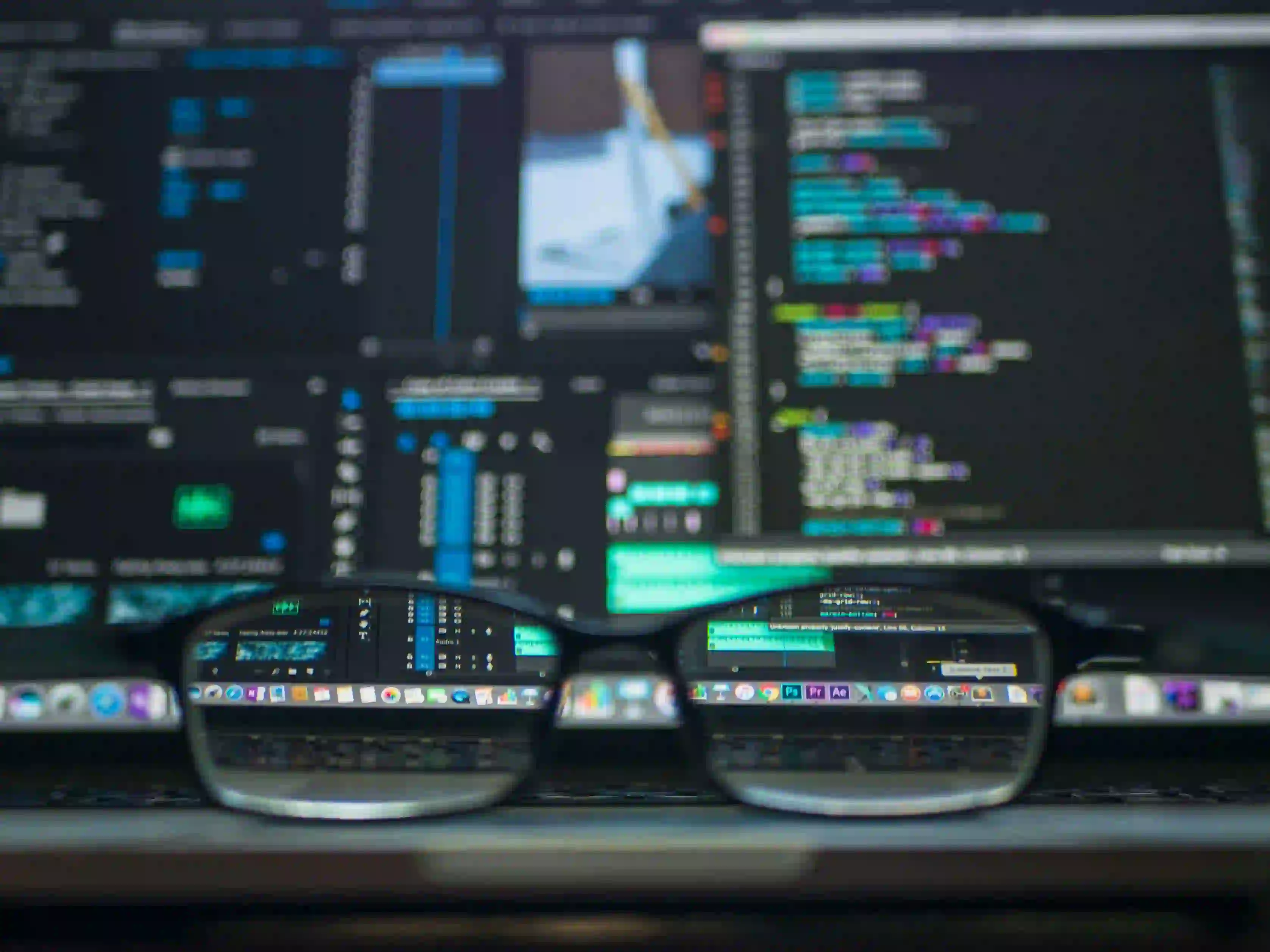
Mastering Optimal String Alignment in Java: Common Pitfalls
When dealing with strings, we often find ourselves faced with the challenge of aligning them optimally. This concept holds great significance in applications like computational biology, spell-checking, and natural language processing. In this blog, we'll dive deep into the common pitfalls associated with string alignment in Java and how to effectively manage them. We’ll also explore crucial methods and provide practical code snippets along the way.
Understanding String Alignment
String alignment essentially refers to the process of arranging two strings in a way that maximizes similarity, often involving dynamic programming techniques. There are various string alignment algorithms, such as Needleman-Wunsch and Smith-Waterman—both critical in bioinformatics.
Typical string alignment outcomes aim to minimize edit distance, which comprises insertions, deletions, and substitutions. Implementing these efficiently in Java is paramount yet can introduce common traps that developers need to avoid.
Common Pitfalls
1. Ignoring Edge Cases
When developing string alignment algorithms, failing to consider edge cases can lead to runtime errors or inaccurate results. Edge cases might include aligning empty strings or contrasting lengths of strings.
Example: Let's analyze how to handle string pairs where one or both are empty.
public static int alignStrings(String s1, String s2) {
// Handle empty strings
if (s1.isEmpty()) return s2.length();
if (s2.isEmpty()) return s1.length();
// Proceed with alignment logic
// ...
}
Why: Here, prior checks prevent unnecessary computations by addressing direct outcomes for empty strings.
2. Inefficient Memory Use
Dynamic programming typically requires a 2D array to store the alignment scores. With longer strings, this can significantly increase memory usage. It’s crucial to optimize this representation.
Example: Instead of a full DP table, use two arrays to save space.
public static int optimalAlignment(String s1, String s2) {
int[] previous = new int[s2.length() + 1];
int[] current = new int[s2.length() + 1];
// Initialization
for (int j = 0; j <= s2.length(); j++) {
previous[j] = j; // Cost of aligning with empty s1
}
for (int i = 1; i <= s1.length(); i++) {
current[0] = i; // Cost of aligning with empty s2
for (int j = 1; j <= s2.length(); j++) {
int cost = (s1.charAt(i-1) == s2.charAt(j-1)) ? 0 : 1;
current[j] = Math.min(Math.min(current[j-1] + 1, previous[j] + 1), previous[j-1] + cost);
}
int[] temp = previous;
previous = current;
current = temp;
}
return previous[s2.length()];
}
Why: Utilizing two one-dimensional arrays instead of a full 2D array saves memory without sacrificing the algorithm's efficiency.
3. Failing to Normalize Input Strings
Strings may have different cases or even contain unwanted characters that can affect alignment results. Normalizing the input ensures more reliable comparisons.
Example: Use a method to preprocess strings.
public static String normalizeString(String s) {
// Convert to lower case and remove non-alphanumeric characters
return s.toLowerCase().replaceAll("[^a-z0-9]", "");
}
Why: This approach ensures that the strings being aligned focus purely on their alphanumeric content, improving accuracy.
4. Not Using Early Termination
Dynamic programming can be resource-intensive. If a subproblem already provides optimal results, terminate earlier. This concept, often referred to as memoization, can save time and computational power.
Example: Add conditions to terminate when optimal solutions are already found.
public static int memoizedAlignment(String s1, String s2) {
// Implement memoization logic
Map<String, Integer> memo = new HashMap<>();
return alignWithMemoization(s1, s2, memo);
}
private static int alignWithMemoization(String s1, String s2, Map<String, Integer> memo) {
String key = s1 + "|" + s2;
if (memo.containsKey(key)) return memo.get(key);
// Alignment logic (similar to previous example)
// ...
int result = // computed value;
memo.put(key, result);
return result;
}
Why: Using a memoization technique prevents repeated calculations, vastly improving performance.
Best Practices for Optimal String Alignment
-
Preprocessing of Input Strings: Always clean and normalize the strings before processing. Removing special characters or unifying case will provide a consistent basis for alignment.
-
Choosing the Right Algorithm: Depending on the context, select from algorithms like Needleman-Wunsch for global alignment and Smith-Waterman for local alignment.
- If you need further insights into these algorithms, check this detailed comparison.
-
Optimize for Space and Time: As illustrated, using techniques like space-saving arrays is essential for larger inputs.
-
Use Libraries When Feasible: Sometimes, relying on well-optimized libraries, such as Apache Commons Lang, can save time on development while ensuring reliability.
The Bottom Line
Mastering optimal string alignment in Java is not merely about implementing the right algorithms; it's also about navigating common pitfalls that can compromise your solution's effectiveness. By paying attention to potential issues such as edge cases, memory inefficiency, string normalization, and proper algorithm selection, you can enhance both performance and correctness in your applications.
As you embark on your journey through string alignment, leveraging these practices will allow you to build robust applications capable of handling complex text processing tasks. For more in-depth coverage on string manipulation techniques, feel free to explore Java String Handling Techniques.
Happy coding!