Troubleshooting Common Hazelcast Publish-Subscribe Issues
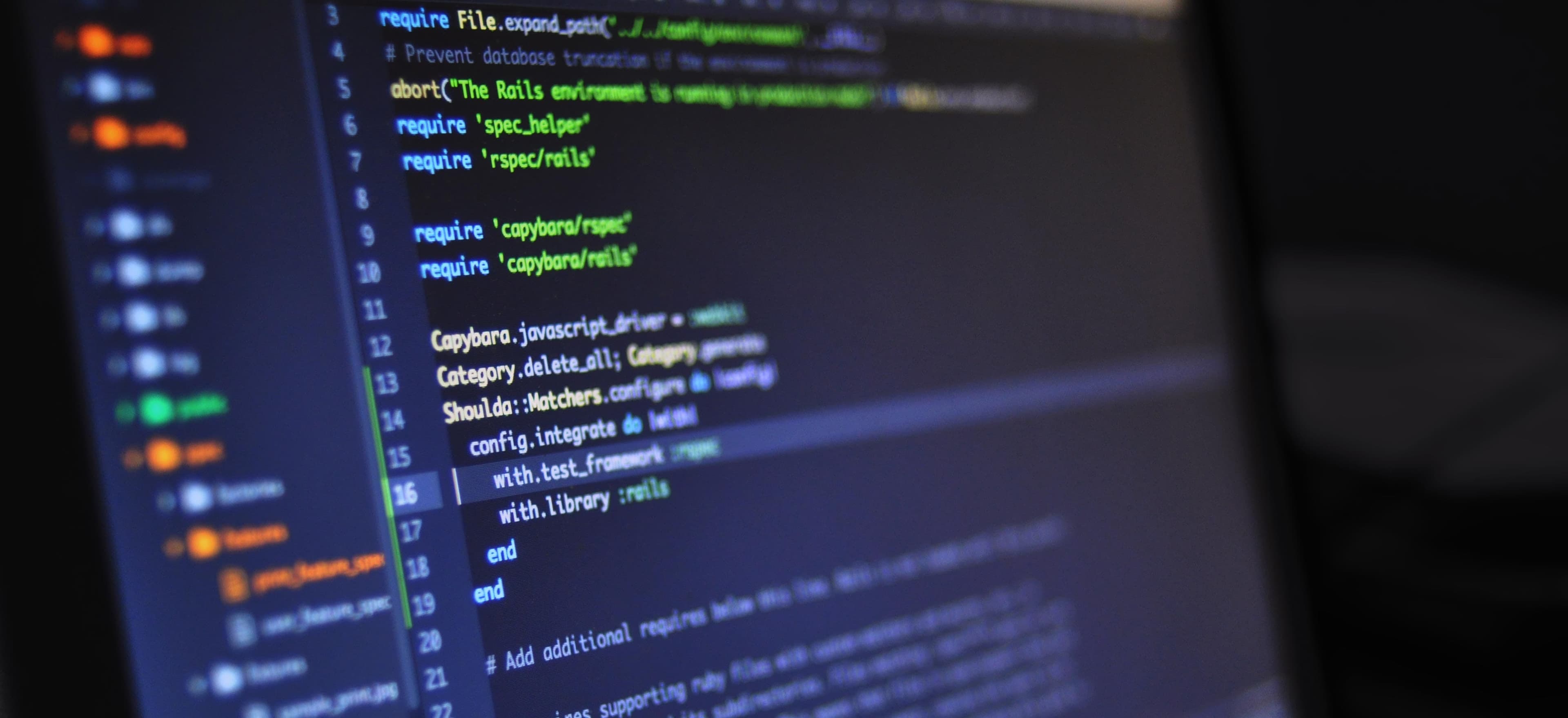
- Published on
Troubleshooting Common Hazelcast Publish-Subscribe Issues
Hazelcast is a powerful in-memory data grid that offers a distributed computing platform for Java applications. One of its essential features is the publish-subscribe model, which facilitates communication between multiple services or components in a system. However, as with any system, it can encounter issues that can disrupt functionality. In this post, we will examine common problems related to Hazelcast's publish-subscribe mechanism and provide solutions to troubleshoot them effectively.
Understanding the Publish-Subscribe Model
Before diving into troubleshooting, it's important to understand the publish-subscribe model itself. In this model, publishers send messages to a topic without needing to know who the subscribers are. Subscribers receive these messages published to the topics they are interested in. This decouples the sender and receiver, enhancing scalability and reducing dependencies.
Despite its advantages, users can face challenges due to configuration errors, network issues, or misuse of the API. Let's explore the common issues and what you can do to fix them.
Common Issues in Hazelcast Publish-Subscribe
1. Missing Messages in Subscription
One frequent issue that developers encounter is that subscribers do not receive messages that were published. This can occur for several reasons:
- Subscription Timing: If a subscriber subscribes to a topic after a message has been published, it will miss that message.
To prevent this, ensure that subscribers are connected and subscribed before any message is sent. Here is an example of proper message handling:
HazelcastInstance hazelcastInstance = Hazelcast.newHazelcastInstance();
ITopic<String> topic = hazelcastInstance.getTopic("exampleTopic");
// Subscriber
topic.addMessageListener(message -> {
System.out.println("Received message: " + message.getMessageObject());
});
// Publisher
topic.publish("Hello Subscribers!");
2. Network Issues
Often, messages may not be delivered due to network-related issues. Ensure that:
- All nodes in the Hazelcast cluster are reachable.
- There aren’t any firewalls or network policies blocking the communication.
You can verify the health of your cluster:
HazelcastInstance hazelcastInstance = Hazelcast.newHazelcastInstance();
Cluster cluster = hazelcastInstance.getCluster();
for (Member member : cluster.getMembers()) {
System.out.println("Member: " + member.getAddress());
}
This snippet provides insight into the nodes in your cluster and helps diagnose network issues.
3. Message Serialization Problems
Messages being published should be serializable. If the message type does not implement Serializable
, subscribers will not be able to deserialize the message, resulting in an error.
Ensure that the objects you publish implement java.io.Serializable
:
public class MyMessage implements Serializable {
private String content;
public MyMessage(String content) {
this.content = content;
}
public String getContent() {
return content;
}
}
When you publish, do so as follows:
MyMessage message = new MyMessage("Hello Serialization!");
topic.publish(message);
4. Listeners Not Triggering
Sometimes, subscribers might not receive any notifications despite being subscribed correctly. This can often happen due to incorrect listener configuration.
Make sure that your listener implementation is done properly. Ensure that it extends MessageListener<T>
:
class MyMessageListener implements MessageListener<MyMessage> {
@Override
public void onMessage(Message<MyMessage> message) {
System.out.println("Received: " + message.getMessageObject().getContent());
}
}
And then add it to the topic:
topic.addMessageListener(new MyMessageListener());
5. High Throughput and Message Loss
When dealing with a high volume of messages, you may experience message loss. The publish-subscribe model does not guarantee message delivery, so in cases of high throughput, it's possible for messages to be dropped.
To mitigate this issue, consider the following strategies:
- Increase Timeouts: Ensure your subscribers have sufficient time to process messages.
- Implement Acknowledgment: You can implement an acknowledgment system where subscribers send confirmation back to the publisher.
6. Configuration Misalignment
When using Hazelcast in a distributed system, misconfigurations between nodes can lead to unpredictable behavior. Ensure that configurations, particularly around networking, are consistent across your Hazelcast instances.
For example, check the hazelcast.xml
configuration file for correct settings:
<network>
<join>
<multicast enabled="false" />
<tcp-ip enabled="true">
<member>192.168.1.1:5701</member>
<member>192.168.1.2:5701</member>
</tcp-ip>
</join>
</network>
Monitoring and Debugging Hazelcast
In addition to resolving specific issues, it can be beneficial to monitor your Hazelcast instance and set up proper logging.
-
Enable Logging: Use SLF4J or another logging framework to keep track of events and errors. Hazelcast logging can provide insights into what's happening under the hood.
-
Management Center: Utilize the Hazelcast Management Center to visualize your cluster health, network stats, and message statistics.
-
Profiling: Use Java profilers (like VisualVM) to identify any bottlenecks in your publish-subscribe operations.
Further Reading
As you dive deeper into Hazelcast and its functionalities, consider exploring the following resources:
- Hazelcast Documentation
- Publish-Subscribe Model Insights
Final Considerations
Troubleshooting make-or-break functionalities like publish-subscribe in Hazelcast requires a clear understanding of both the framework and potential pitfalls. By leveraging the insights provided here, you should be well-equipped to handle common issues, ensuring seamless communication in your distributed applications.
Remember, proper configuration, monitoring, and staying updated on Hazelcast best practices are vital to maintaining a healthy application ecosystem. Happy coding!
Checkout our other articles