Overcoming Common Spring Boot Actuator Performance Issues
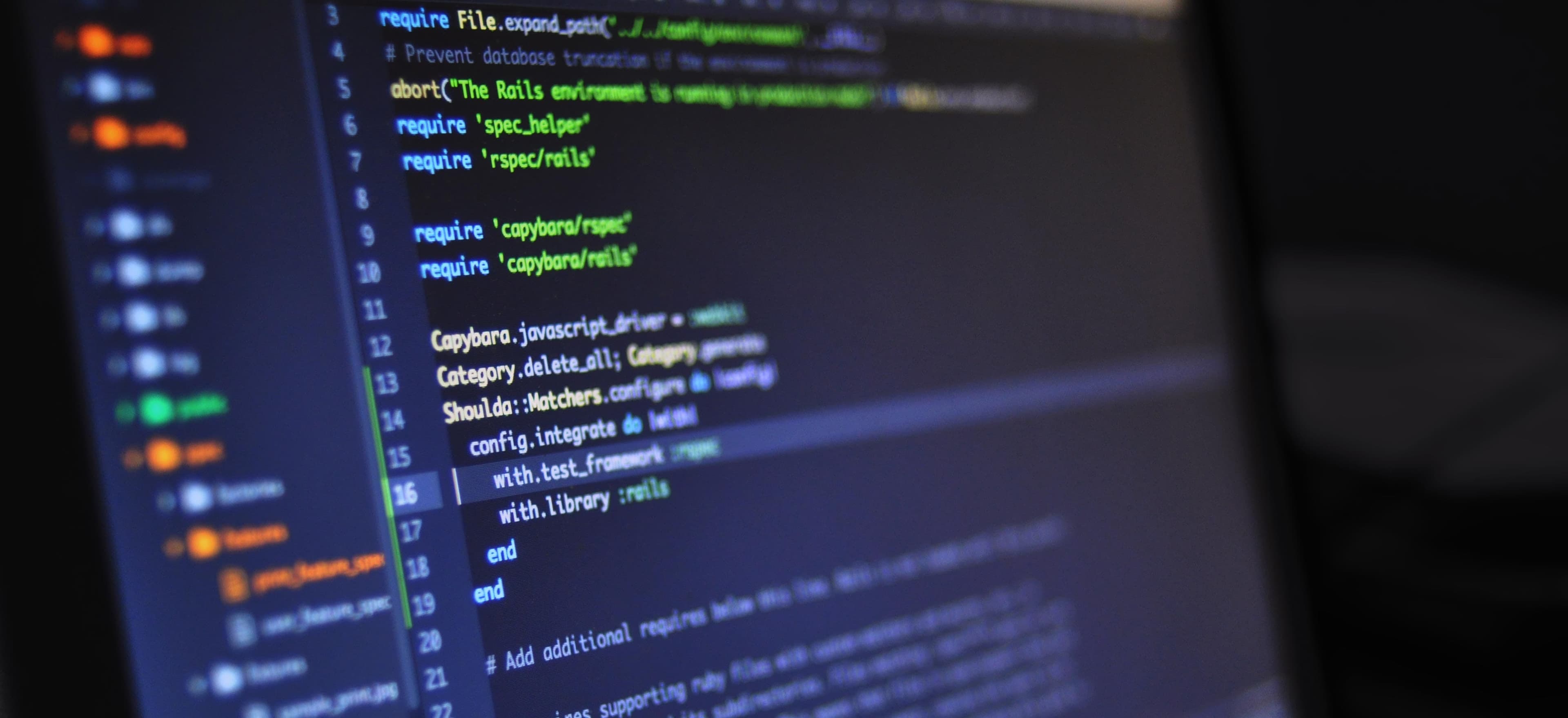
- Published on
Overcoming Common Spring Boot Actuator Performance Issues
Spring Boot Actuator is an essential toolkit designed to help you monitor and manage your Spring Boot applications. As applications scale, performance becomes a critical factor. Understanding how to manage the performance of Spring Boot Actuator can significantly enhance your application's efficiency. In this blog post, we will explore common performance issues associated with Spring Boot Actuator and how to efficiently overcome them.
Understanding Spring Boot Actuator
Spring Boot Actuator provides a variety of production-ready features that help developers monitor and manage their applications. With features like health checks, metrics gathering, and application information endpoints, Actuator simplifies operations. However, if not appropriately configured, it can create performance bottlenecks.
Key Features of Spring Boot Actuator
- Health Checks: Automatically reports the application's health status.
- Metrics Gathering: Collects and exposes metrics about your application.
- Endpoint Exposure: Allows you to expose various endpoints to control and monitor your application.
- Environment Info: Gives insights into the configuration and environment.
Performance Issues in Spring Boot Actuator
While Actuator is immensely useful, improper configuration can lead to performance issues. Here are a few common problems you might encounter:
1. Resource-Heavy Metrics Collection
One prevalent issue is the overhead of collecting and processing metrics, especially when using the default settings. For applications with many active connections or requests, the metrics can become a significant performance burden.
Solution
To mitigate this, consider reducing the frequency of the metrics collection. Customize the metrics collection interval to suit your application’s needs. You can adjust this in your application.properties
:
management.metrics.export.simple.enabled=true
management.metrics.export.simple.step=30s
In this example, we configure the metrics collection to be less frequent, thus reducing the performance overhead.
2. Unnecessary Endpoint Exposure
By default, Spring Boot Actuator exposes many endpoints. For larger applications, this can expose sensitive information and lead to performance degradation.
Solution
You can limit the endpoints that are exposed in your configuration:
management.endpoints.web.exposure.include=health,info
Here, we are only exposing the health
and info
endpoints. This reduces overhead and mitigates risk.
3. Health Checks Impacting Performance
Heavy health checks can delay response times or cause failures, especially when they check external resources like databases or messaging services.
Solution
Optimize your health checks by specifying what to include:
management.health.db.enabled=false
In this scenario, the database check is disabled, reducing the load on your system. You should only check what's necessary for your application to run efficiently.
4. Thread Pool and Connection Pool Implications
Spring Boot applications often utilize connection pools for their database interactions. Improper configurations can slow down the performance when Actuator's metrics try to gather and report on pools that are under heavy load.
Solution
Monitor thread pools, and ensure that they are configured correctly. You can adjust the maximum and minimum pool sizes:
spring.datasource.hikari.maximumPoolSize=15
spring.datasource.hikari.minimumIdle=5
This code snippet sets the maximum number of connections, balancing performance and resource utilization.
5. Logging Overhead
Actuator can generate an extensive amount of logs, especially when monitoring all endpoints. This results in significant logging overhead.
Solution
Configure the logging level appropriately. You can selectively reduce logging for Actuator:
logging.level.org.springframework.boot.actuate=ERROR
This limits the logging to only error messages from Actuator, thereby improving performance.
Real-World Example: Optimizing Actuator in a Microservices Environment
Imagine deploying several microservices using Spring Boot, each exhibiting Actuator's capabilities. Here's how you can optimize performance in such an environment.
-
Evaluate Metrics: Focus on critical metrics relevant to application performance, like response time and error rates.
-
Fine-tune Health Indicators: Disable unnecessary health checks and pre-emptively test interactions with external services to minimize the impact on performance.
-
Use Prometheus for Metrics: Consider integrating Prometheus and Grafana for a more comprehensive metrics collection rather than relying solely on built-in Actuator metrics. This reduces load while enabling powerful visualization capabilities.
management.metrics.export.prometheus.enabled=true
The above configuration enables Prometheus integration, allowing the collection and analysis of metrics without impacting application performance significantly.
The Closing Argument
Spring Boot Actuator provides a robust solution for monitoring and managing applications. However, it's essential to recognize that its default configurations are not always optimal for high-performance scenarios. By understanding the common pitfalls and adopting best practices, you can effectively overcome these challenges.
For more detailed coverage, check the official Spring Boot Actuator documentation. Keep experimenting with the configurations mentioned above until you find the perfect balance between performance and observability for your applications.
Additional Resources
- Spring Boot Official Documentation
- Monitoring Spring Boot Applications
- Prometheus for Monitoring Java Applications
Optimizing Spring Boot Actuator requires a strategic approach. With the discussed techniques, you can enhance your application's performance while retaining powerful monitoring capabilities. Happy coding!
Checkout our other articles