Common Pitfalls When Uploading Multiple Files in Spring MVC
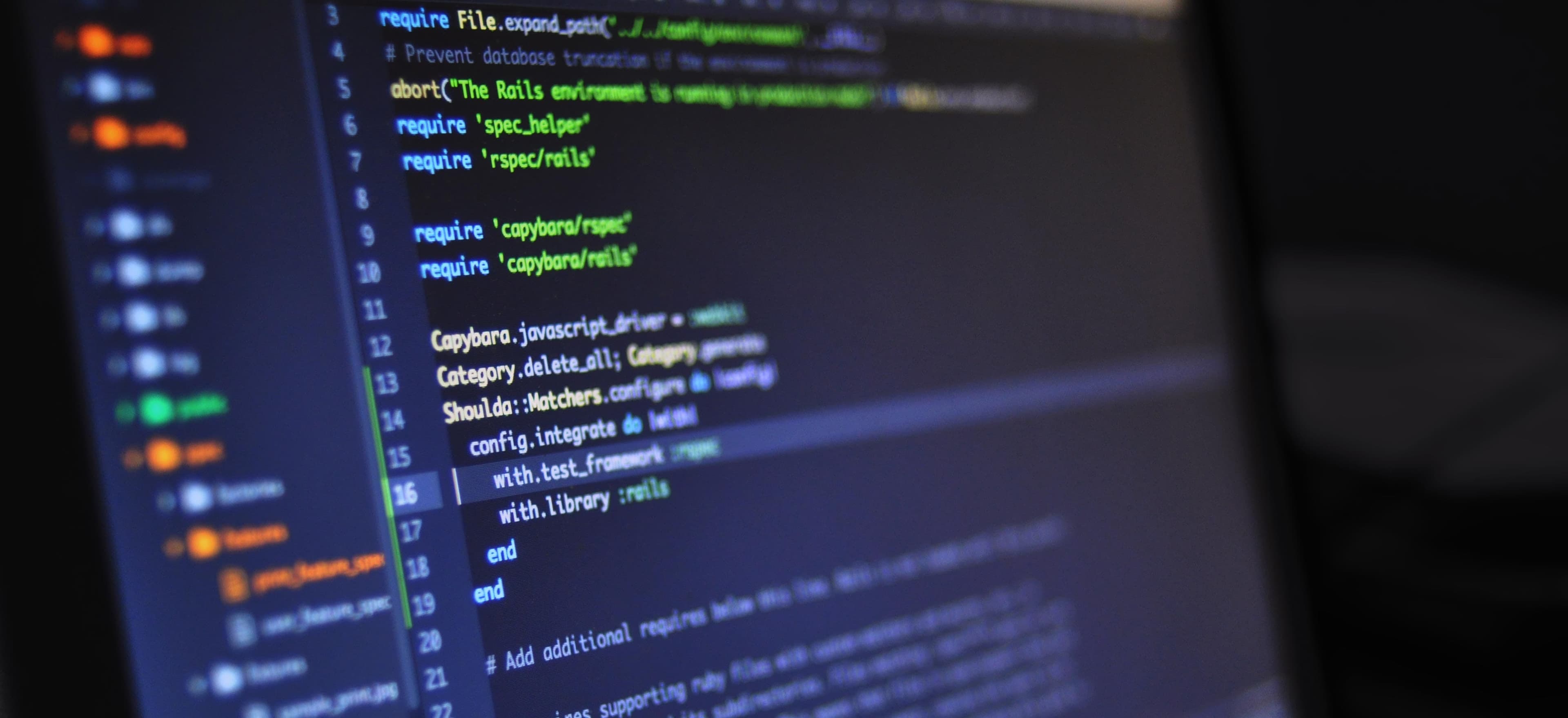
- Published on
Common Pitfalls When Uploading Multiple Files in Spring MVC
Uploading files is a common requirement in modern web applications. However, when dealing with multiple file uploads, developers may encounter various pitfalls that can lead to less than optimal outcomes. In this blog post, we will explore the common pitfalls associated with uploading multiple files in Spring MVC, how to avoid them, and sample code snippets to illustrate best practices.
Understanding Spring MVC File Uploads
Spring MVC is a popular framework that simplifies web application development in Java. File uploads can be done using MultipartFile
, which represents an uploaded file in Spring's web context. The framework provides seamless integration with file upload capabilities through the Apache Commons FileUpload library or Servlet 3.0's @MultipartConfig
.
Pitfall 1: Forgetting to Configure Multipart Resolver
One of the first things you might overlook when handling file uploads is configuring a MultipartResolver
. By default, Spring MVC doesn't know how to handle multipart requests unless you tell it to.
Solution
To handle multiple file uploads efficiently, you need to define a CommonsMultipartResolver
or StandardServletMultipartResolver
in your Spring configuration. Here’s how you do it in XML configuration:
<bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<!-- Set maximum file size -->
<property name="maxUploadSize" value="5242880"/> <!-- 5 MB -->
</bean>
And here’s how to configure it using Java configuration:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.multipart.commons.CommonsMultipartResolver;
@Configuration
public class AppConfig {
@Bean
public CommonsMultipartResolver multipartResolver() {
CommonsMultipartResolver resolver = new CommonsMultipartResolver();
resolver.setMaxUploadSize(5242880); // 5 MB
return resolver;
}
}
Pitfall 2: Not Handling the List<MultipartFile>
Correctly
When users upload multiple files, you will typically receive a List<MultipartFile>
as part of your controller method parameters. Failing to iterate through these files appropriately can lead to errors.
Solution
Make sure to iterate through the list and handle potential errors for each file individually. Here is an example of how you can process multiple file uploads:
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import java.io.File;
import java.io.IOException;
@RestController
@RequestMapping("/api")
public class FileUploadController {
@PostMapping("/upload")
public String handleFileUpload(@RequestParam("files") MultipartFile[] files) {
StringBuilder response = new StringBuilder();
for (MultipartFile file : files) {
if (file.isEmpty()) {
continue; // Skip empty files
}
try {
File destinationFile = new File("/uploads/" + file.getOriginalFilename());
file.transferTo(destinationFile);
response.append("Uploaded: ").append(file.getOriginalFilename()).append("<br/>");
} catch (IOException e) {
response.append("Failed to upload: ").append(file.getOriginalFilename()).append("<br/>");
}
}
return response.toString();
}
}
In this code, we ensure that each file is checked for emptiness and handled appropriately, reducing the risk of processing errors.
Pitfall 3: Not Validating the File Format
Another common mistake is failing to validate the uploaded file types. Users may upload malicious files or unwanted formats that could compromise your application.
Solution
Implement file type validation to ensure that only specific file formats are allowed. Here’s how you can do that:
import org.springframework.web.multipart.MultipartFile;
import java.util.Arrays;
import java.util.List;
private void validateFile(MultipartFile file) {
List<String> allowedContentTypes = Arrays.asList("image/jpeg", "image/png", "application/pdf");
if (!allowedContentTypes.contains(file.getContentType())) {
throw new IllegalArgumentException("Invalid file type: " + file.getContentType());
}
}
This function can be called within the upload loop in the controller to validate each file before processing.
Pitfall 4: Ignoring File Size Limits
Uploading large files can cause performance issues and lead to out-of-memory errors if not properly managed.
Solution
As mentioned in the configuration part, always set a maximum file size limit in your MultipartResolver
. You can also implement additional checks in the controller:
if (file.getSize() > maxFileSize) {
throw new IllegalArgumentException("File is too large: " + file.getOriginalFilename());
}
Pitfall 5: Overwriting Existing Files
When multiple files with the same name are uploaded, the last uploaded file may overwrite previous ones. This can lead to loss of data.
Solution
To prevent this, create unique file names by appending timestamps or unique IDs. Here’s an example:
import java.util.UUID;
public String getUniqueFileName(String originalFilename) {
String fileExtension = originalFilename.substring(originalFilename.lastIndexOf("."));
return UUID.randomUUID().toString() + fileExtension; // use UUID for unique file names
}
// Usage
File destinationFile = new File("/uploads/" + getUniqueFileName(file.getOriginalFilename()));
file.transferTo(destinationFile);
Pitfall 6: Inadequate Error Handling
Lack of proper error handling can lead to a poor user experience. Users should be informed if their upload fails for any reason.
Solution
Ensure to catch exceptions comprehensively and provide relevant feedback. Here's an improved error handling version of the upload method:
@PostMapping("/upload")
public ResponseEntity<String> handleFileUpload(@RequestParam("files") MultipartFile[] files) {
StringBuilder response = new StringBuilder();
for (MultipartFile file : files) {
if (file.isEmpty()) {
response.append("Skipped empty file.<br/>");
continue;
}
try {
validateFile(file); // validate format
File destinationFile = new File("/uploads/" + getUniqueFileName(file.getOriginalFilename()));
file.transferTo(destinationFile);
response.append("Uploaded: ").append(file.getOriginalFilename()).append("<br/>");
} catch (IllegalArgumentException e) {
response.append("Error: ").append(e.getMessage()).append("<br/>");
} catch (IOException e) {
response.append("Failed to upload: ").append(file.getOriginalFilename()).append("<br/>");
}
}
return ResponseEntity.ok(response.toString());
}
This method provides the user with concise error messages that can help in diagnosing issues.
Lessons Learned
Uploading multiple files in Spring MVC can present various challenges. However, by being aware of common pitfalls and implementing best practices, you can create a robust file upload feature within your application. Always remember to validate file types, handle errors gracefully, and ensure that you have correctly configured your multipart resolver.
For more in-depth learning, consider visiting the Spring MVC documentation. Here, you can find further information on handling file uploads and the configurations that come into play.
Happy coding!
Checkout our other articles