Ditching Utility Classes: Embracing OOP for Clean Code
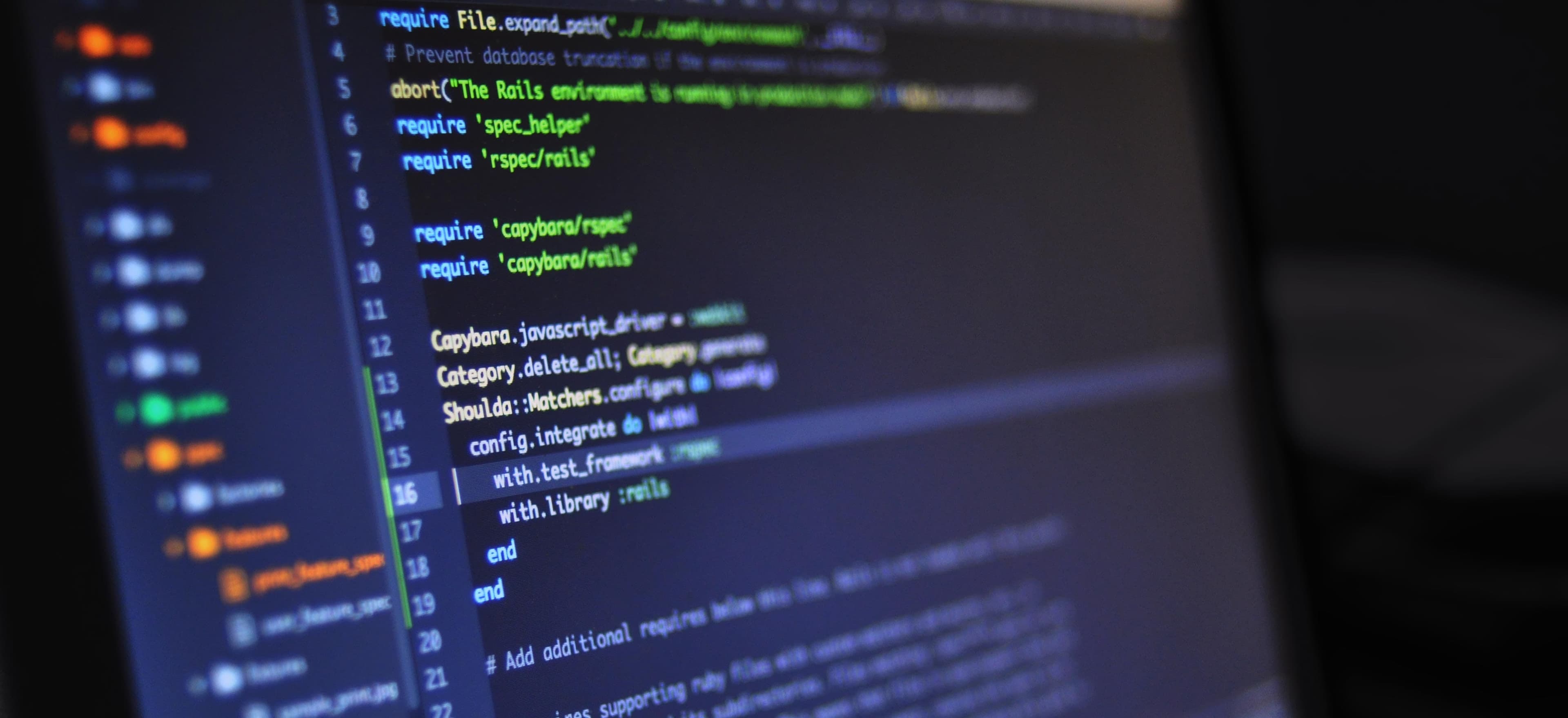
- Published on
Ditching Utility Classes: Embracing OOP for Clean Code
In the world of software development, maintaining clean and manageable code is paramount. As developers, we often come across utility classes—those catch-all containers for static methods that simplify certain tasks. However, relying on these utility classes can lead to messy code and hinder the principles of Object-Oriented Programming (OOP). In this blog post, we will explore the drawbacks of utility classes and how embracing OOP can help achieve cleaner, more maintainable code.
Understanding Utility Classes
Utility classes are collections of static methods used to perform common tasks. A typical example might include methods for string manipulation, date formatting, or file handling. While utility classes can provide convenience, they often lead to several issues:
- Global State: Utility classes often operate on global states which can introduce hidden dependencies in your code.
- Hard to Test: Testing the static methods of a utility class requires running the entire class rather than testing individual behaviors.
- Less Flexibility: They provide no opportunity for extension or modification through subclassing.
- Violation of OOP Principles: Utility classes generally undermine the four pillars of OOP—abstraction, encapsulation, inheritance, and polymorphism.
An Example of a Utility Class
Here’s a simple example of a utility class that handles common string operations:
public class StringUtil {
public static boolean isBlank(String str) {
return str == null || str.trim().isEmpty();
}
public static String toUpperCase(String str) {
return str == null ? null : str.toUpperCase();
}
}
This class provides static methods for checking if a string is blank and converting a string to uppercase. However, it also comes with the drawbacks discussed above.
The OOP Approach
To enhance code quality, we can adopt an OOP approach and replace utility classes with more elegant solutions like services, models, and exceptions.
Encapsulation with a Service Class
Instead of a utility class, we can create a service class that encapsulates string operations. This version can be easily tested and extended without altering existing code.
public class StringService {
public boolean isBlank(String str) {
return str == null || str.trim().isEmpty();
}
public String toUpperCase(String str) {
return str == null ? null : str.toUpperCase();
}
}
In this approach, we can inject the StringService
into components that require string operations, allowing for more organized dependency management.
Advantages of OOP Over Utility Classes
- Improved Testability: OOP methods can be tested independently, facilitating unit tests.
- Reduced Coupling: The usage of dependency injection allows for looser coupling and cleaner code architecture.
- Increased Reusability: OOP promotes code reuse through inheritance and interfaces, leading to efficient code maintenance.
- Clarity and Organization: With the encapsulation of functionalities in classes, code organization improves significantly, reducing complexity.
Leveraging Polymorphism
By using interfaces and polymorphism, we can further enhance flexibility. Let’s define an interface for string operations:
public interface StringOperations {
boolean isBlank(String str);
String toUpperCase(String str);
}
Now we can implement this interface in our string service class:
public class StringService implements StringOperations {
@Override
public boolean isBlank(String str) {
return str == null || str.trim().isEmpty();
}
@Override
public String toUpperCase(String str) {
return str == null ? null : str.toUpperCase();
}
}
This leads to a flexible codebase where future modifications can be made with minimal impact on existing code.
Composition over Inheritance
While polymorphism brings advantages, we can take it one step further by embracing the composition over inheritance principle. By composing our classes with required functionalities, we can avoid issues often associated with deep inheritance trees.
An Example of Composition
Here's how you would structure a class that utilizes the string service via composition:
public class UserNotification {
private final StringService stringService;
public UserNotification(StringService stringService) {
this.stringService = stringService;
}
public void notifyUser(String message) {
if (stringService.isBlank(message)) {
System.out.println("Notification: Message cannot be empty!");
} else {
System.out.println("Notification: " + stringService.toUpperCase(message));
}
}
}
This UserNotification
class now uses the StringService
to perform its functionality. This structure allows you to change the StringService
implementation without altering the UserNotification
class.
To Wrap Things Up
Ditching utility classes in favor of OOP-centric approaches offers clear benefits for code maintainability, testability, and overall cleanliness. This transition aligns with the core principles of object orientation, allowing us to create flexible, reusable, and manageable codebases.
Moving to OOP doesn’t have to be an overnight process. Gradually refactor your code, replacing utility classes with service-oriented designs, and watch your code transform into a more organized and scalable solution.
For more insights on OOP principles, consider checking out This Resource on OOP Basics or James Gosling's perspective on Java and OOP.
Embrace OOP for clean code, and you will find your coding life becomes more enjoyable and productive.
By acknowledging the need to modernize our approach and focusing on the right principles, we can significantly enhance the quality of our software projects in the long run. Happy coding!