Top Access Control Mistakes That Compromise App Security
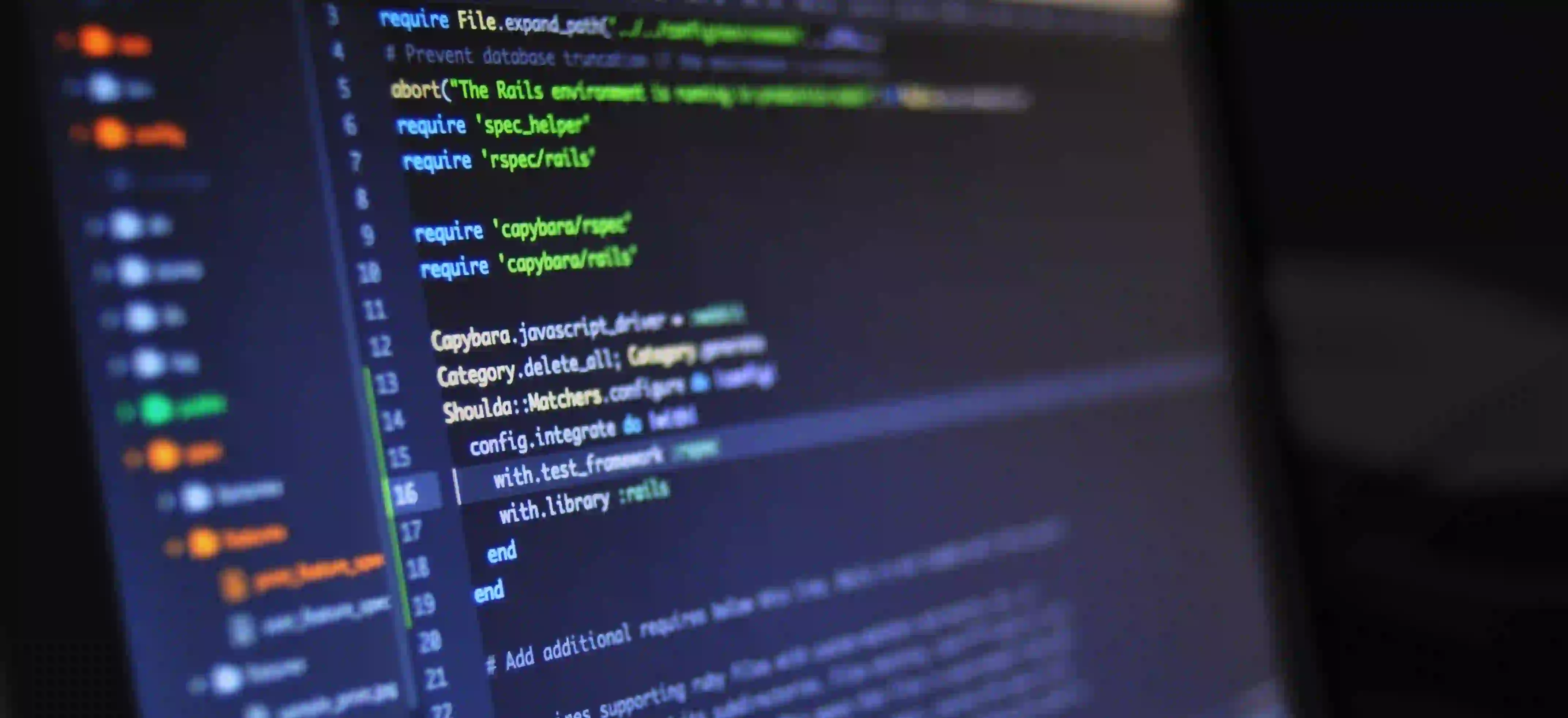
Top Access Control Mistakes That Compromise App Security
Access control is a fundamental aspect of application security. It's the gatekeeper mechanism that ensures users can only interact with resources they are authorized to access. Despite its importance, many developers and organizations make critical mistakes in implementing access controls. In this post, we will discuss common mistakes, backed by effective strategies and best practices to secure your applications.
Understanding Access Control
Before diving into the mistakes, let's clarify what access control entails. Access control mechanisms are techniques that restrict access to resources based on user permissions. They ensure that users cannot perform actions or access data beyond their authorized limits.
Access control can be broken down into several models, including:
- Discretionary Access Control (DAC) - Access is granted at the discretion of the owner.
- Mandatory Access Control (MAC) - Access is granted based on fixed policies.
- Role-Based Access Control (RBAC) - Access is determined by the user’s role within the organization.
Why Access Control Matters
Failing to implement strong access controls can lead to data breaches, unauthorized access, and exposure of sensitive information. This can result in significant financial losses, reputational damage, and legal ramifications.
Common Access Control Mistakes
- Insufficient User Authentication
One of the most prevalent mistakes is weak or insufficient user authentication methods. When applications fail to validate user identities rigorously, they compromise the entire access control model.
Best Practice:
- Implement multi-factor authentication (MFA) to add an additional layer of security.
Example:
// Using Spring Security for MFA
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin()
.and()
.sessionManagement()
.sessionFixation().migrateSession()
.and()
.csrf().disable(); // Not recommended in production, for illustration only
// MFA logic implementation would go here, possibly integrating with SMS or email verification
}
}
Commentary: The code snippet illustrates how to secure endpoints, ensuring that all requests are authenticated.
- Overly Permissive Access Controls
Developers often assign excessive permissions to users, thereby broadening the attack surface. The principle of least privilege states that users should only have access necessary to perform their jobs.
Best Practice:
Conduct an access review regularly to ensure permissions are appropriately assigned.
Example:
@PreAuthorize("hasRole('ADMIN')")
public void deleteUser(Long userId) {
// Method logic to delete user goes here
}
Commentary: In this example, only users with the ADMIN role can execute the deleteUser method, showcasing the principle of least privilege in action.
- Hardcoded Credentials
Embedding credentials or API keys directly in the source code is a critical misstep. This practice exposes sensitive information if the codebase is compromised or shared via public repositories.
Best Practice:
Use environment variables or secure vaults to manage sensitive configurations.
Example:
// Using environment variables for sensitive data
public class Config {
public String getDbUser() {
return System.getenv("DB_USER");
}
public String getDbPassword() {
return System.getenv("DB_PASSWORD");
}
}
Commentary: By reading credentials from environment variables, you enhance security by not exposing sensitive data within the code.
- Ignoring API Security
With the increasing use of APIs, many developers overlook securing these interfaces. APIs lacking robust access control can lead to severe vulnerabilities, exposing back-end systems.
Best Practice:
Always authenticate and authorize API requests.
Example:
@GetMapping("/api/user/{id}")
@PreAuthorize("hasRole('USER') or hasRole('ADMIN')")
public User getUserById(@PathVariable Long id) {
// Logic to retrieve user by ID
}
Commentary: In this example, the API endpoint is restricted to specific roles, ensuring that only authorized users can access sensitive information.
- Relying Solely on Client-side Security
Some developers mistakenly assume that client-side security controls are sufficient. This can lead to code being manipulated in the browser, allowing unauthorized access.
Best Practice:
Always enforce access controls on the server side.
Example:
@PostMapping("/api/protected/resource")
public ResponseEntity<?> accessProtectedResource(@AuthenticationPrincipal UserDetails userDetails) {
// Server-side validation for access
if (!userDetails.hasAccessToResource()) {
throw new AccessDeniedException("You do not have access to this resource");
}
// Resource logic here...
return ResponseEntity.ok().build();
}
Commentary: This method ensures all access control checks are validated on the server side, independent of the client environment.
- Neglecting Regular Security Audits
Many organizations fail to conduct regular audits of their access control mechanisms and user permissions. This leads to outdated permissions being retained, which poses security risks.
Best Practice:
Schedule periodic security audits and access reviews.
Final Considerations
Security is an ongoing process, and addressing access control mistakes is crucial to safeguarding your applications and data. By implementing strong authentication, enforcing the principle of least privilege, securing your APIs, and conducting regular audits, you can significantly reduce risks.
For more resources on access control and application security, consider exploring the following links:
Remember, the goal is to effectively secure your applications without compromising user experience. Security and usability can go hand in hand when the right practices are implemented.
By staying vigilant and adopting best practices for access control, you’re proactively creating a safer environment for both your users and your organization.