Common Pitfalls When Running Java Class in a Subprocess
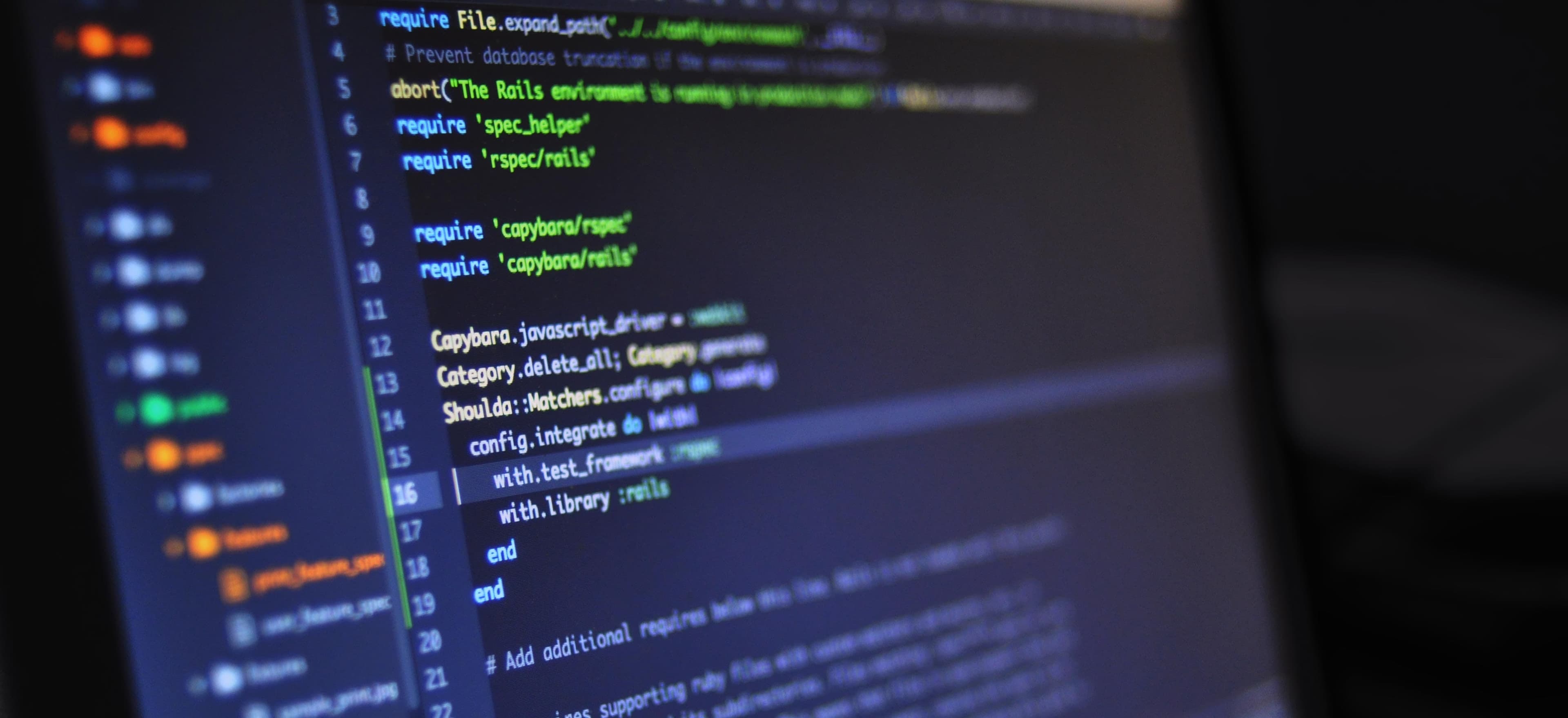
- Published on
Common Pitfalls When Running Java Class in a Subprocess
Java is a powerful programming language that allows developers to build and run applications across different platforms seamlessly. However, when working with subprocesses, developers sometimes encounter challenges that can lead to unexpected behaviors. In this blog post, we will explore some common pitfalls when running a Java class in a subprocess and how to avoid them.
Understanding Subprocess Management in Java
A subprocess is a separate process created by a parent process. In Java, we can manage subprocesses using the ProcessBuilder
and Runtime
classes. Running a subprocess can help us execute external commands, scripts, or even other Java applications.
Consider this snippet to create a subprocess in Java:
import java.io.IOException;
public class SubprocessExample {
public static void main(String[] args) {
try {
ProcessBuilder processBuilder = new ProcessBuilder("java", "-version");
Process process = processBuilder.start();
process.waitFor();
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
}
In this example, we create a subprocess to run the Java version command. The ProcessBuilder
class allows us to configure and run external commands easily. However, running a Java class as a subprocess presents its unique set of challenges.
1. Handling I/O Streams
One common pitfall is not correctly handling the input and output streams of the subprocess, which can lead to blocked processes or other unexpected behavior. Every subprocess has three standard streams:
- Standard Input (stdin)
- Standard Output (stdout)
- Standard Error (stderr)
If you fail to read from these streams properly, it can result in a deadlock, as the buffer will fill up, causing your subprocess to hang.
Example Solution
To properly handle the streams, ensure you create separate threads to read output and error streams:
public class ProcessOutputHandler {
private static void handleProcessOutput(Process process) {
new Thread(() -> {
try (BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println("OUTPUT: " + line);
}
} catch (IOException e) {
e.printStackTrace();
}
}).start();
new Thread(() -> {
try (BufferedReader reader = new BufferedReader(new InputStreamReader(process.getErrorStream()))) {
String line;
while ((line = reader.readLine()) != null) {
System.err.println("ERROR: " + line);
}
} catch (IOException e) {
e.printStackTrace();
}
}).start();
}
}
Why This Matters
By utilizing separate threads, we ensure that the application remains responsive and can handle large outputs without blocking.
2. Process Termination
Another common pitfall is not properly terminating subprocesses. Failing to terminate a process can lead to resource leaks. If your subprocess creates background threads or performs extensive operations, you must ensure that it exits correctly.
Example of Handling Termination
You can signal termination using either destroy()
or destroyForcibly()
methods from the Process
class:
public class ProcessTermination {
public static void main(String[] args) {
try {
Process process = new ProcessBuilder("longRunningProcess").start();
// Wait for a certain time or condition
Thread.sleep(5000);
process.destroy(); // Gracefully stop the process
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
}
The Importance of Cleanup
Being diligent about cleaning up subprocesses prevents memory leaks and ensures that system resources are efficiently utilized. As a best practice, always check if your subprocess has ended using isAlive()
or by analyzing the exit value with waitFor()
.
3. Environment Variables
Subprocesses inherit their environment variables from the parent process. Not accounting for this can lead to problems, such as the subprocess not being able to find necessary paths or configurations.
Setting Environment Variables
You can customize the environment using the environment()
method in ProcessBuilder
:
ProcessBuilder processBuilder = new ProcessBuilder("yourJavaClass");
Map<String, String> env = processBuilder.environment();
env.put("MY_VARIABLE", "someValue");
Why Environment Matters
By explicitly managing environment variables, you can ensure that your subprocess has the necessary context to run successfully.
4. Handling Exit Codes
It's critical to check the exit code of a subprocess to determine if it succeeded or failed. The exit code can provide insights into what went wrong if the subprocess encounters issues.
Checking Exit Codes
After finishing the subprocess execution, you can retrieve its exit value:
int exitCode = process.waitFor();
if (exitCode != 0) {
System.err.println("Process failed with exit code " + exitCode);
} else {
System.out.println("Process completed successfully");
}
Understanding Exit Codes
By analyzing exit codes, you can implement error-handling logic and make your applications more robust. A zero exit code typically indicates success, while any non-zero value suggests an error occurred.
5. Thread Safety
When dealing with subprocesses, make sure you consider thread safety. Many utility classes are not thread-safe by default, which can lead to unpredictable behavior when accessed by multiple threads.
Using Thread-Safe Classes
You might consider using thread-safe data structures, such as ConcurrentLinkedQueue
, to manage data between threads safely.
import java.util.concurrent.ConcurrentLinkedQueue;
public class ThreadSafeExample {
private static ConcurrentLinkedQueue<String> outputQueue = new ConcurrentLinkedQueue<>();
public static void main(String[] args) {
// Use threads to populate the queue with process output as shown earlier
}
}
Why Thread Safety Is Crucial
Thread safety avoids potential race conditions, ensuring that data integrity is maintained and reducing the likelihood of hard-to-diagnose bugs.
Wrapping Up
Running a Java class in a subprocess can be fraught with common pitfalls, including I/O stream management, process termination, and environment variable handling. By adhering to best practices, such as those discussed above, you can avoid these issues and create robust applications.
For deeper insights into process management in Java, you can refer to the official Java documentation or explore Java concurrency best practices for more information.
By learning from these pitfalls and solutions, developers can leverage Java subprocesses more effectively, leading to greater productivity and fewer headaches in application development. Happy coding!
Checkout our other articles