Common Java Mistakes Beginners Make and How to Avoid Them
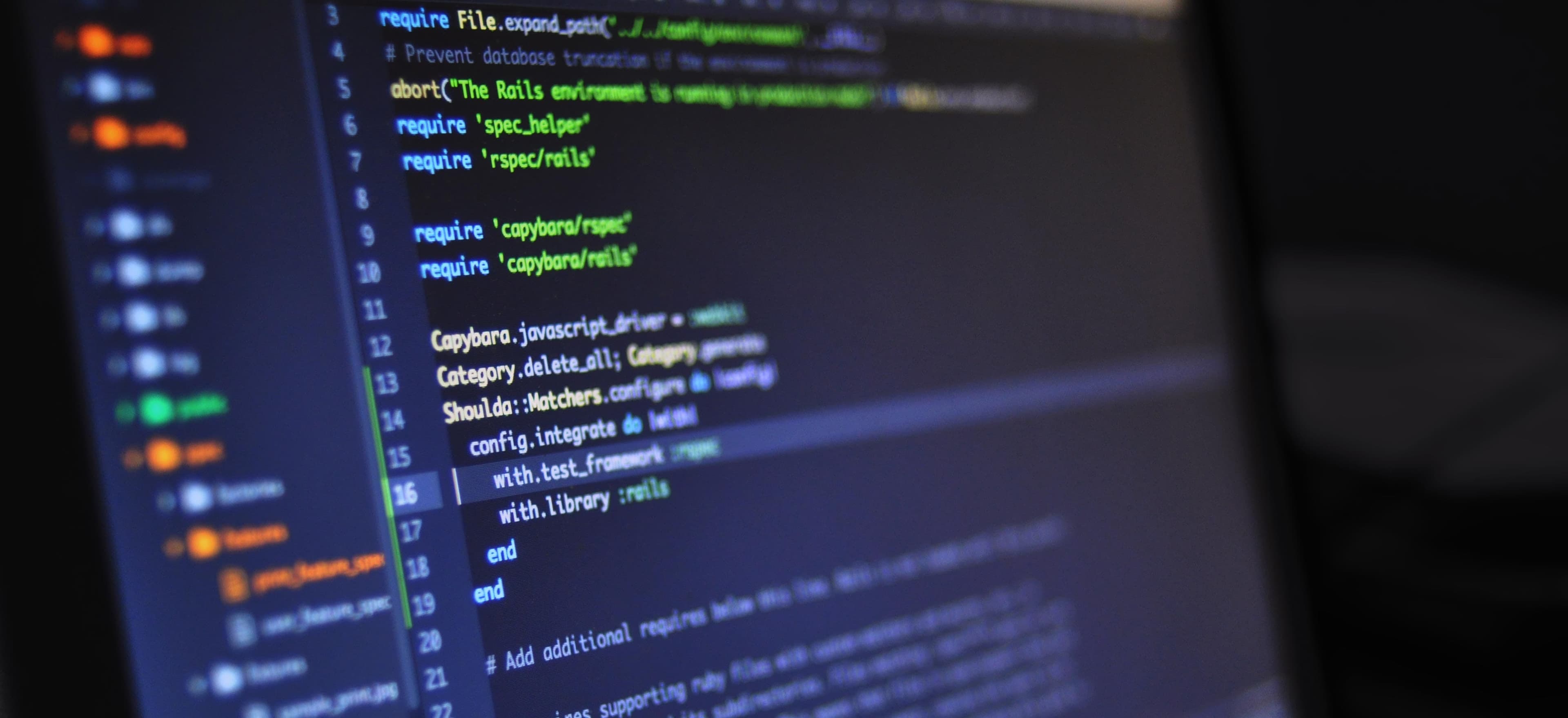
- Published on
Common Java Mistakes Beginners Make and How to Avoid Them
Java, a versatile and powerful programming language, has become a staple for both novice and seasoned developers. However, beginners often stumble on certain issues that can lead to confusion and frustration. In this post, we will explore common mistakes made by Java beginners and provide guidance on how to avoid them.
1. Ignoring Variable Initialization
One of the most common mistakes is failing to initialize variables. In Java, local variables must be explicitly initialized before they can be used, while instance and class variables have default values.
Example 1: Uninitialized Local Variable
public class Main {
public static void main(String[] args) {
int number; // Local variable
System.out.println(number); // Compile-time error
}
}
Why This Happens
Java does not provide a default value for local variables, which can lead to compilation errors. Always ensure local variables are initialized before use.
How to Avoid
int number = 10; // Initialized variable
System.out.println(number); // Output: 10
Always declare and initialize your variables in the same line to prevent errors.
2. Misunderstanding Object References
Beginners often misinterpret how Java handles objects and references. Unlike some languages, Java uses reference types rather than direct variable copies.
Example 2: Confusing Object Reference
class Dog {
String name;
Dog(String name) {
this.name = name;
}
}
public class Main {
public static void main(String[] args) {
Dog dog1 = new Dog("Buddy");
Dog dog2 = dog1; // dog2 references the same object as dog1
dog2.name = "Max"; // Changes name for both dog1 and dog2
System.out.println(dog1.name); // Output: Max
}
}
Why This Happens
Beginners mistakenly believe dog1 and dog2 are independent objects. However, they point to the same memory location.
How to Avoid
To create a distinct object, use a copy constructor or a cloning method (assuming the class implements Cloneable
).
class Dog {
String name;
Dog(String name) {
this.name = name;
}
// Copy constructor
Dog(Dog other) {
this.name = other.name;
}
}
public class Main {
public static void main(String[] args) {
Dog dog1 = new Dog("Buddy");
Dog dog2 = new Dog(dog1); // Creates a new object
dog2.name = "Max";
System.out.println(dog1.name); // Output: Buddy
System.out.println(dog2.name); // Output: Max
}
}
3. Forgetting to Handle Exceptions
Java places a strong emphasis on error handling through exceptions. Beginners often overlook this, leading to application crashes when unexpected events occur.
Example 3: Not Catching Exceptions
public class Main {
public static void main(String[] args) {
String str = null;
System.out.println(str.length()); // Throws NullPointerException
}
}
Why This Happens
Not anticipating potential errors can cause runtime exceptions.
How to Avoid
Use try-catch blocks to handle exceptions gracefully.
public class Main {
public static void main(String[] args) {
String str = null;
try {
System.out.println(str.length());
} catch (NullPointerException e) {
System.out.println("String is null, can't perform length operation.");
}
}
}
By handling exceptions, you allow your program to recover gracefully instead of crashing unexpectedly.
4. Not Utilizing Java Collections Properly
Java provides a rich set of Collection classes, but beginners may misuse or misunderstand when to use them.
Example 4: Using Arrays Instead of Lists
public class Main {
public static void main(String[] args) {
int[] numbers = new int[5];
// Wrong approach: Fixed size, difficult to resize
}
}
Why This Happens
Arrays have a fixed size and can complicate dynamic additions of elements.
How to Avoid
Make use of ArrayList
, which is resizable and more flexible:
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<Integer> numbers = new ArrayList<>(); // Dynamically resizable
numbers.add(1);
numbers.add(2);
System.out.println(numbers); // Output: [1, 2]
}
}
Utilize Java's Collections Framework for greater ease in managing dynamic datasets.
5. Neglecting Object-Oriented Principles
One of the foundational principles of Java is Object-Oriented Programming (OOP). Beginners sometimes ignore key OOP concepts like encapsulation, inheritance, and polymorphism.
Example 5: Lack of Encapsulation
class Person {
public String name; // Not encapsulated
}
Why This Happens
Without encapsulating data, you risk exposing your data to unintended modifications.
How to Avoid
Utilize access modifiers and provide getter and setter methods:
class Person {
private String name; // Encapsulated
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
This enhances the integrity of your objects and maintains clear access control.
6. Unfamiliarity with Array Indexing
Java arrays are zero-indexed, meaning the first item in an array is at index 0. Beginners may struggle with this concept, leading to off-by-one errors.
Example 6: Off-by-One Error
public class Main {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5};
// Potential off-by-one error
System.out.println(numbers[5]); // ArrayIndexOutOfBoundsException
}
}
Why This Happens
Miscounting the number of elements in an array can lead to accessing invalid indexes.
How to Avoid
Always remember arrays start at index 0:
System.out.println(numbers[4]); // Correct usage: Output: 5
7. Poor Naming Conventions
Java places a significant emphasis on code readability. Beginners sometimes use unclear or inconsistent naming conventions.
Example 7: Ambiguous Variable Names
public class Main {
public static void main(String[] args) {
int a = 10; // Poor variable name
String b = "Hello";
}
}
Why This Happens
Unclear names can significantly hinder the comprehension of your code.
How to Avoid
Use meaningful names that provide context:
public class Main {
public static void main(String[] args) {
int userAge = 10; // Clear variable name
String greetingMessage = "Hello";
}
}
Final Considerations
Avoiding these common mistakes can greatly elevate your Java programming skills. From variable initialization and object references to error handling and embracing OOP principles, each section serves as a stepping stone to becoming a proficient Java developer.
For more resources on Java programming, check out Oracle’s Java Documentation. Understanding Java's foundational principles will bolster your growth as a developer.
Stay curious and keep coding!
Checkout our other articles