Unlocking Column Toggler Issues in PrimeFaces 5.0 DataTable
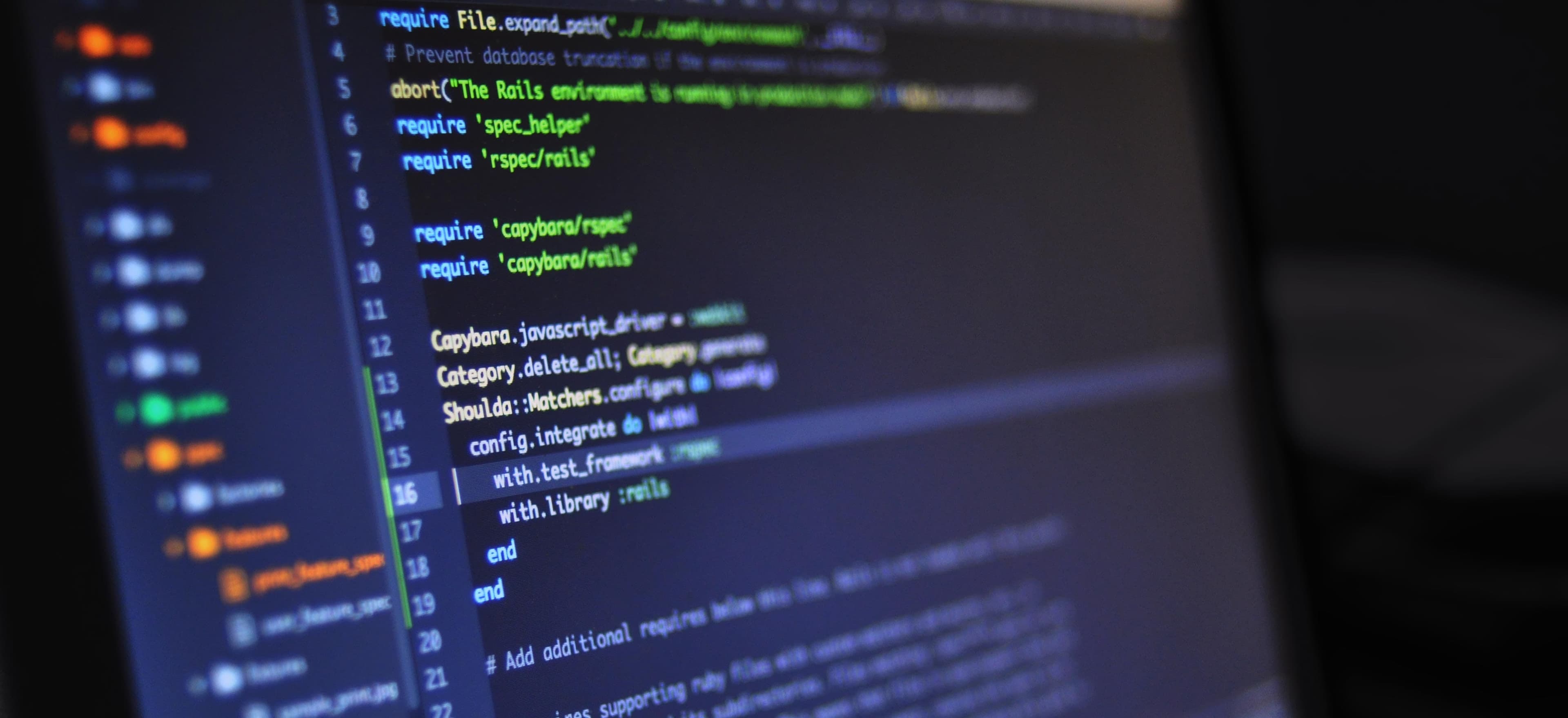
- Published on
Unlocking Column Toggler Issues in PrimeFaces 5.0 DataTable
PrimeFaces is a powerful Java framework that allows developers to enrich their applications with graphically appealing components. One of the standout features of PrimeFaces is the DataTable
, a flexible component used for displaying and manipulating data. However, like any framework, it comes with its own set of challenges. In this post, we will explore common issues with the column toggler feature in PrimeFaces 5.0 DataTable and how you can resolve them efficiently.
Understanding PrimeFaces DataTable
The DataTable
component serves as a grid for displaying data in a tabular format, making it easily readable and interactable. One of the essential features is the column toggler, which allows users to show or hide specific columns based on their preferences. This dynamic view can significantly enhance user experience, especially when dealing with vast amounts of data.
Before we dive into the issues, let’s start with a basic implementation of the DataTable with column toggler.
Basic Implementation
Here is a basic example of how to implement the column toggler in a PrimeFaces DataTable.
<h:form>
<p:dataTable id="dataTable" var="product" value="#{productBean.products}" widgetVar="dt">
<p:column>
<f:facet name="header">
Product Name
</f:facet>
#{product.name}
</p:column>
<p:column>
<f:facet name="header">
Price
</f:facet>
#{product.price}
</p:column>
<p:column>
<f:facet name="header">
Quantity
</f:facet>
#{product.quantity}
</p:column>
</p:dataTable>
<p:columnToggler for="dataTable" />
</h:form>
Breakdown of Code
- h:form: This is a JavaServer Faces (JSF) form used to encapsulate the DataTable component.
- p:dataTable: This is the PrimeFaces DataTable component. It uses the
value
attribute to bind to a list ofProduct
objects available in the managed bean. - p:column: Each column of the DataTable is defined within
<p:column>
, allowing you to specify headers and display data. - p:columnToggler: This line adds the column toggler feature to the DataTable.
Common Issues with Column Toggler
Having laid out a basic implementation, it's time to address some frequent issues developers face with the column toggler in PrimeFaces 5.0.
Issue 1: Column Toggler Not Displaying
One frequently encountered issue is that the column toggler does not appear. This often occurs due to the following reasons:
- Incorrect ID Reference: Ensure that the
for
attribute in<p:columnToggler>
matches the ID of your DataTable component.
Fixing the Issue
Double-check the IDs to ensure consistency.
<p:columnToggler for="dataTable" />
Issue 2: Header Not Being Toggled
You might find that when toggling columns, the headers do not disappear or show as expected.
Possible Causes
- JavaScript Errors: Check for any console errors in your browser that might be preventing the toggler from functioning.
- Component Visibility: Confirm that you're not using CSS that hides the headers inadvertently.
Fixing the Issue
Inspect your browser’s console for any JavaScript errors. To ensure the toggler works, the following step can be implemented.
<p:ajax event="columnToggle" listener="#{productBean.onColumnToggle}" />
Issue 3: Backward Compatibility
If you have upgraded from a previous version, other compatibility issues might arise due to changes in API methods.
Recommended Action
Check the PrimeFaces migration guide for any adjustments to your existing code.
Advanced Column Toggling with Dynamic Columns
For applications which require dynamic columns, you can leverage PrimeFaces functionality to generate columns at runtime.
Example of Dynamic Columns
<h:form>
<p:dataTable id="dynamicTable" value="#{dynamicView.filteredProducts}" var="product">
<p:ajax event="rowSelect" listener="#{dynamicView.onRowSelect}" />
<p:columns value="#{dynamicView.columns}" var="column">
<f:facet name="header">
#{column.header}
</f:facet>
#{product[column.prop]}
</p:columns>
</p:dataTable>
<p:columnToggler for="dynamicTable" />
</h:form>
Explanation of Dynamic Columns
- p:columns: This component allows you to define columns dynamically. You can have a collection of descriptors (headers and properties) to configure the DataTable.
#{column.header}
and#{product[column.prop]}
: These expressions bind the header and the value of each column dynamically based on data.
Closing Remarks
The column toggler is a beneficial feature in PrimeFaces DataTable, allowing users the flexibility to manage their view of the displayed data. By implementing the toggler correctly, addressing common issues proactively, and leveraging dynamic columns, developers can provide an enhanced user experience.
Should you wish to delve deeper into other PrimeFaces components, feel free to utilize resources available at PrimeFaces Showcase or consult the official documentation.
Remember, errors can be a part of the development process. Embracing them with curiosity and resolving them will not only refine your coding skills but also lead to better applications. Happy coding!
Checkout our other articles