Overcoming Common Pitfalls in System Testing Rules
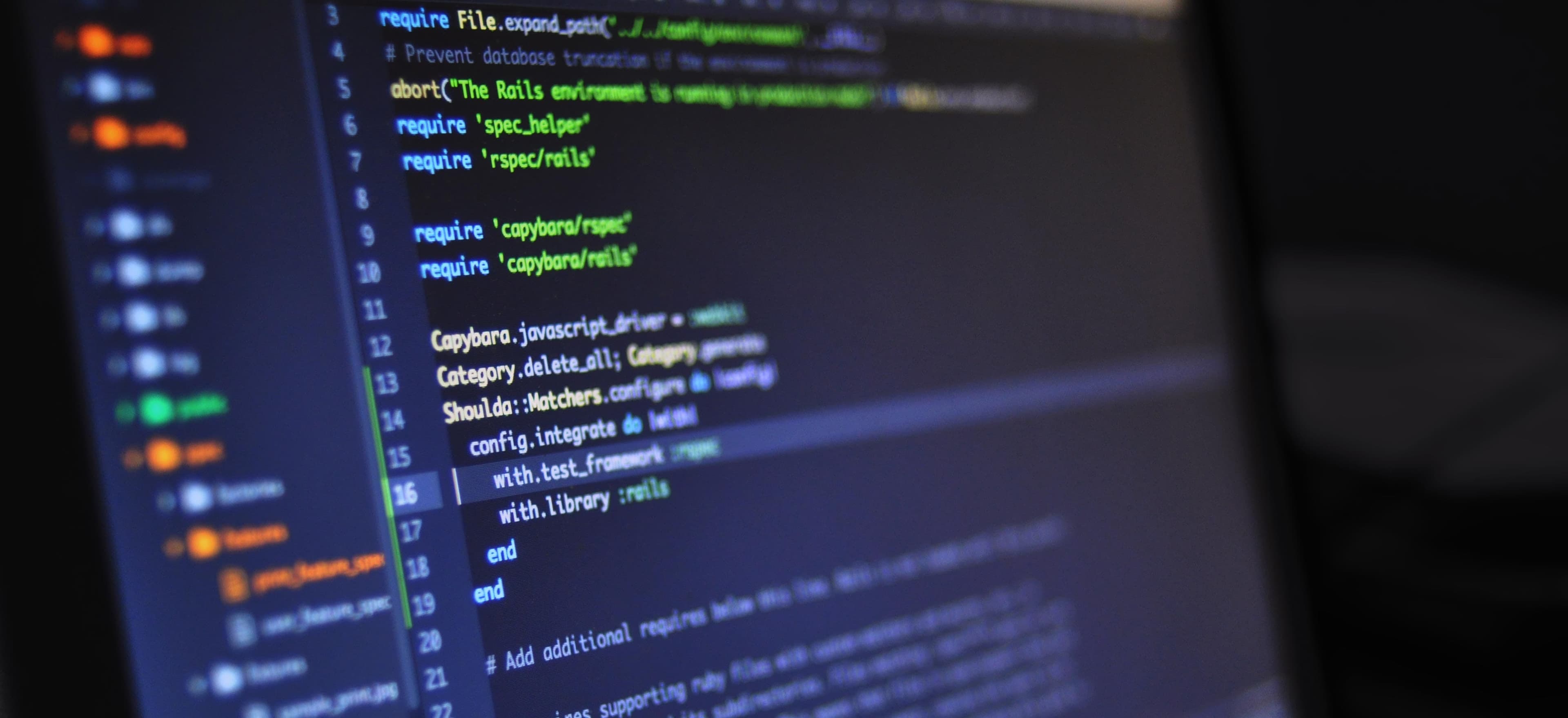
- Published on
Overcoming Common Pitfalls in System Testing Rules
System testing is a critical phase in the software development lifecycle. It ensures that the entire system works as intended before it’s deployed to the production environment. However, many teams struggle with common pitfalls when implementing system testing rules. In this blog post, we will explore these pitfalls and, more importantly, how to overcome them. Let’s dive into systematic testing best practices, essential strategies, and valuable code snippets to enforce your testing rules effectively.
Understanding System Testing
Before delving into pitfalls, let’s clarify what system testing entails. System testing evaluates the complete and fully integrated software product to verify its compliance with specified requirements. It is one of the last phases of testing before the product goes to the users.
The critical objectives of system testing include:
- Validating functionality against requirements.
- Identifying any discrepancies before deployment.
- Assessing the end-to-end system specifications and the overall performance.
Common Pitfalls in System Testing
-
Lack of Clear Requirements
System testing begins with clearly defined requirements. Without them, teams often struggle to create meaningful test cases. This can lead to incomplete testing and missed defects.
Solution: Ensure a collaborative approach between stakeholders and the testing team to define clear, documented requirements. Use use cases or user stories to clarify the expected system behavior.
-
Ignoring Non-Functional Requirements
A failure to consider non-functional requirements such as performance, usability, and security can result in a system that functions well but is not user-friendly or secure.
Solution: Include test cases that focus on non-functional aspects. For instance, performance testing and security testing should be integrated into the system testing process.
-
Inadequate Test Data Management
Using inadequate or incorrect test data can lead to misleading test results. Teams might receive false positives or miss critical issues.
Solution: Establish a robust test data management strategy. Use a combination of real and synthetic data mimicking real-world scenarios.
Here is a small example in Java demonstrating how you might create mock data for testing purposes:
import java.util.ArrayList; import java.util.List; public class TestDataGenerator { public static List<User> generateMockUsers(int numberOfUsers) { List<User> users = new ArrayList<>(); for (int i = 0; i < numberOfUsers; i++) { users.add(new User("User" + i, "user" + i + "@example.com")); } return users; } public static void main(String[] args) { // Generate and display mock users List<User> users = generateMockUsers(5); users.forEach(System.out::println); } } class User { private String name; private String email; public User(String name, String email) { this.name = name; this.email = email; } @Override public String toString() { return "Name: " + name + ", Email: " + email; } }
This example demonstrates the importance of good test data. It quickly creates multiple user instances to validate scenarios effectively.
-
Over-Reliance on Automated Testing
While automation is vital in system testing, over-reliance on it can cause teams to ignore manual testing's contextual insight and exploration.
Solution: Balance automated and manual testing. Use automation for repetitive tasks and regression testing, while reserving manual tests for explorative testing and scenarios that require human judgment.
-
Unclear Testing Scope
Without a well-defined scope, tests can become scattered, leading to important areas being overlooked or overtested.
Solution: Clearly outline the scope of your testing based on the identified requirements. Develop detailed test plans that include defined objectives, milestones, and metrics.
Best Practices for Effective System Testing
-
Develop a Comprehensive Test Plan
A well-structured test plan serves as a roadmap for the testing process. It should include:
- Testing objectives.
- Scope of testing.
- Resources required.
- Schedules and timelines.
-
Prioritize Test Cases
Given the limited time and resources, it is essential to prioritize test cases based on risk and requirements’ importance.
Why it matters: Prioritized testing ensures that high-risk scenarios are tested first, helping to uncover critical issues early in the testing phase.
-
Utilize the Right Testing Tools
Selecting the right tools is crucial for efficient test management, execution, and reporting. Tools such as Selenium for web applications, LoadRunner for performance testing, and JMeter for load testing help in achieving quality assurance goals.
-
Embrace Continuous Integration/Continuous Deployment (CI/CD)
Integrating testing into a CI/CD pipeline helps detect defects early and encourages a shift-left approach.
Code Snippet:
Here is a basic example using a Jenkins pipeline script for CI/CD to run tests:
pipeline { agent any stages { stage('Build') { steps { // Build the project sh 'mvn clean install' } } stage('Test') { steps { // Run tests sh 'mvn test' } } } post { always { junit '**/target/surefire-reports/*.xml' } } }
This pipeline script automates the build and testing stages, minimizing human error and improving efficiency.
-
Conduct Regular Retrospectives
After testing phases, hold retrospectives to identify what worked well and what needs improvement. This fosters a culture of continuous learning and adaptation among team members.
My Closing Thoughts on the Matter
System testing is crucial for ensuring that software meets its intended requirements and expectations. By avoiding common pitfalls and following best practices, teams can enhance their testing strategies, deliver quality products, and increase users' satisfaction.
For more in-depth discussions on system and software testing, consider exploring IEEE Testing Standards or Software Testing Fundamentals.
Embrace these strategies, and transform your approach to system testing, paving the way for successful software deployment.
Checkout our other articles