Solving JSON Schema Generation Issues in Jersey Applications
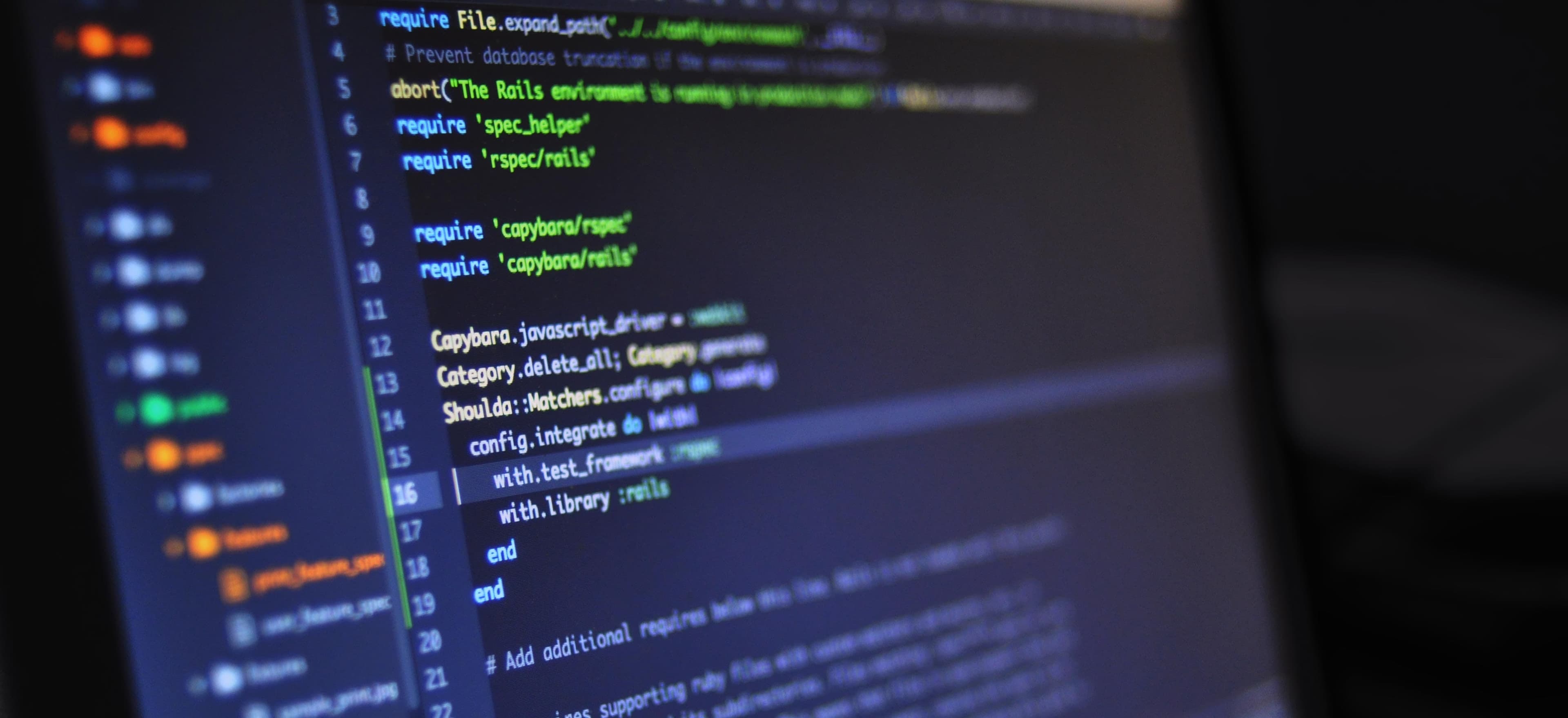
- Published on
Solving JSON Schema Generation Issues in Jersey Applications
When developing RESTful web services using Jersey, one common task is generating JSON Schemas from your Java model classes. This allows you to validate your API's request and response payloads. However, issues can often arise during this process. In this blog post, we will explore how to effectively generate JSON Schema in Jersey applications, troubleshoot common problems, and ensure that your API adheres to a defined structure.
Understanding JSON Schema
Before delving into the troubleshooting of JSON schema generation, let's clarify what JSON Schema is. According to json-schema.org, JSON Schema provides a vocabulary that allows you to annotate and validate JSON documents. It can greatly enhance the clarity and structure of APIs.
Where does Jersey fit in? Jersey is an open-source framework that simplifies the development of RESTful services in Java. While it offers great features for building REST APIs, generating a valid JSON Schema from Java model classes can sometimes present challenges.
Getting Started with Jersey and JSON Schema
To illustrate how to tackle JSON schema generation in Jersey applications, let's begin by setting up a simple Jersey application.
Setting Up a Jersey Application
-
POM Dependency: Make sure you have the necessary dependency for Jersey in your
pom.xml
file.<dependency> <groupId>org.glassfish.jersey.containers</groupId> <artifactId>jersey-container-servlet-core</artifactId> <version>2.35</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.12.0</version> </dependency> <dependency> <groupId>com.github.fge</groupId> <artifactId>json-schema-validator</artifactId> <version>2.2.6</version> </dependency>
-
Create a Resource Class:
Create a simple resource class to handle HTTP requests.
import javax.ws.rs.*; import javax.ws.rs.core.MediaType; import java.util.HashMap; import java.util.Map; @Path("/users") public class UserResource { private static Map<Integer, User> userStore = new HashMap<>(); @POST @Consumes(MediaType.APPLICATION_JSON) @Produces(MediaType.APPLICATION_JSON) public User createUser(User user) { // Assuming the user has an ID field generated elsewhere userStore.put(user.getId(), user); return user; } }
-
Define the Model Class:
Next, you will need a model class. Here's a simple model for our User entity.
public class User { private int id; private String name; private String email; // Getters and Setters public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } }
Generating JSON Schema
The main issue that developers face with Jersey applications is generating a proper JSON Schema from their Java objects. Let's generate a schema for our User
class.
Using Jackson's Annotations
One simple way to generate JSON Schemas is to leverage Jackson annotations. This requires the jackson-module-jsonSchema
module. Update your dependencies:
<dependency>
<groupId>com.fasterxml.jackson.module</groupId>
<artifactId>jackson-module-jsonSchema</artifactId>
<version>2.12.0</version>
</dependency>
Generating JSON Schema Programmatically
Here’s how to create the JSON schema for the User
class programmatically:
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.module.jsonSchema.JsonSchema;
import com.fasterxml.jackson.module.jsonSchema.factories.SchemaFactoryWrapper;
public class JsonSchemaGenerator {
public String generateSchema() throws Exception {
ObjectMapper mapper = new ObjectMapper();
SchemaFactoryWrapper visitor = new SchemaFactoryWrapper();
mapper.acceptJsonFormatVisitor(User.class, visitor);
JsonSchema jsonSchema = visitor.finalSchema();
return mapper.writeValueAsString(jsonSchema);
}
}
Explanation of the Code
-
ObjectMapper
: This is the primary class of Jackson library that enables conversion between Java objects and JSON. -
SchemaFactoryWrapper
: This is used to capture the JSON schema representation as you visit the Java object. -
acceptJsonFormatVisitor
: This method processes the schema of the specified class. -
finalSchema
: This returns the final schema representation.
This utility function can be invoked in your application to generate and return the JSON schema for any model class.
Troubleshooting Common Issues
Typically, developers face several issues while generating JSON Schemas with Jersey. Here are a few common situations:
Problem 1: Missing Fields in Schema
Issue: Your generated schema does not include certain fields from your Java class.
Solution: Ensure that your fields have the correct get/set methods. Jackson relies on these to access the field values. Annotations such as @JsonProperty
can also be used to explicitly define how the fields should be named in JSON.
import com.fasterxml.jackson.annotation.JsonProperty;
public class User {
@JsonProperty("user_id")
private int id;
private String name;
private String email;
// Getters and Setters...
}
Problem 2: Incorrect Data Types or Formats
Issue: The schema generated contains incorrect data types.
Solution: Double-check the data types of your fields. Sometimes, using List<String>
instead of String[]
or vice versa may lead to confusion in schema output. Properly annotating your data types can ensure accurate representation.
Problem 3: Schema Generation Errors
Issue: You receive exceptions when attempting Schema generation.
Solution: Ensure all your dependencies are compatible and updated to the latest version. You should also catch exceptions during schema generation and log them appropriately.
try {
String schema = new JsonSchemaGenerator().generateSchema();
System.out.println(schema);
} catch (Exception e) {
e.printStackTrace(); // Log the error for further investigation
}
Additional Enhancements and Considerations
-
Validation: Implement validation checks using the JSON Schema Validator for incoming requests to ensure they comply with the schema. This can prevent backend failures and improve the robustness of your API.
-
Documentation: Use tools like Swagger to generate documentation and visualize your API endpoints and models, which includes the JSON schema.
-
Testing: Write unit tests to ensure that your schema generation works as expected. This can help catch issues before they affect your application.
Final Considerations
Generating JSON Schemas in Jersey applications might initially seem daunting, but with the right approach and tools, it can be a breeze. By following best practices with annotations, proper error handling, and validation mechanisms, you can ensure that your API remains consistent and reliable.
Next time faced with challenges in generating JSON Schemas, revisit the techniques discussed in this blog post to resolve issues proactively. For more information on JSON Schema, feel free to explore the JSON Schema website for comprehensive resources.
This approach not only improves the quality of your API but also enhances the overall development experience in your Jersey applications. Happy coding!
Checkout our other articles