Common API Design Mistakes That Hurt Performance
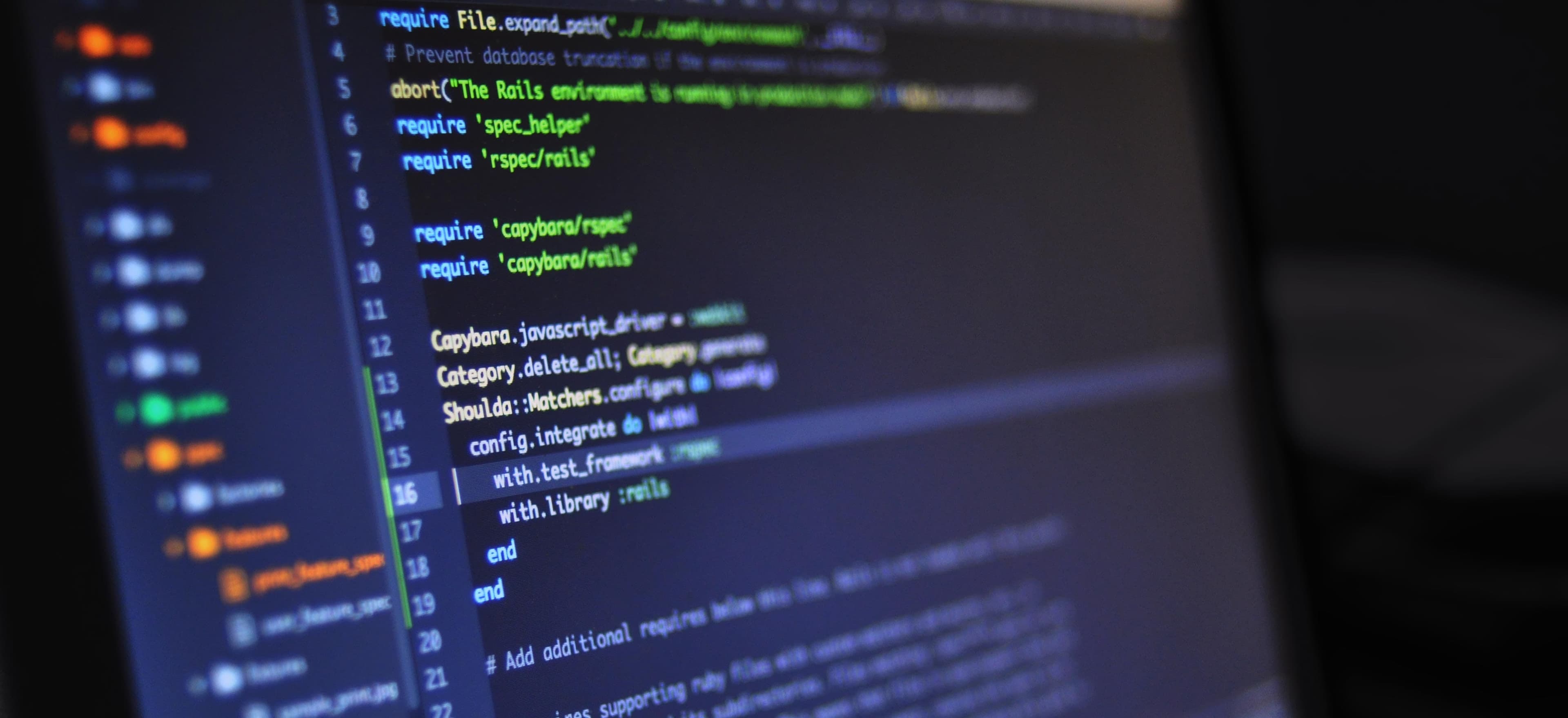
- Published on
Common API Design Mistakes That Hurt Performance
In today's digital landscape, APIs (Application Programming Interfaces) play a pivotal role in connecting services, applications, and data. However, poorly designed APIs can hinder performance and lead to a bad user experience. Below, we delve into common pitfalls in API design that can directly impact performance. We also explore best practices to avoid these traps and create efficient API solutions.
Table of Contents
- Not Versioning Your API
- Over-fetching and Under-fetching Data
- Ignoring Caching Mechanisms
- Using Synchronous Calls
- Neglecting Error Handling
- Conclusion
Not Versioning Your API
The Importance of Versioning
Versioning is crucial for maintaining the integrity of your API as it evolves. Without proper versioning, changes may inadvertently break existing integrations.
How to Version:
Typically, APIs are versioned through the URL. For instance:
GET /api/v1/users
This indicates that you are referring to version 1 of the users' endpoint. When a breaking change is necessary, you can deploy a v2:
GET /api/v2/users
Why It Matters:
By using versioning, you safeguard the existing functionality for users who still rely on your previous version. It also allows for gradual shifts, giving developers time to adapt their applications to new changes.
More information about API versioning can be found in this article.
Over-fetching and Under-fetching Data
Understanding Fetching
APIs should return only the data required for a particular request. Over-fetching occurs when the API sends more data than needed, while under-fetching happens when data is insufficient, leading to multiple requests.
Example of Over-fetching:
Consider retrieving user data where the client only needs the user's name:
{
"id": "123",
"name": "John Doe",
"email": "john@example.com",
"address": "123 Street Name, City, Country"
}
In this case, returning the address and email is over-fetching. It can be minimized to:
{
"name": "John Doe"
}
Solution Using Query Parameters:
You can accomplish this by allowing clients to specify fields they need:
GET /api/users?fields=name
This ensures that clients get only the necessary data, reducing bandwidth and processing costs.
Ignoring Caching Mechanisms
Why Caching is Essential
APIs are often repeatedly called for the same data. Ignoring caching can result in unnecessary database hits, significantly impacting performance.
Implementing Cache Strategies:
You can use HTTP caching headers to instruct clients and intermediate caches to save the results of expensive calls. For instance:
Cache-Control: public, max-age=3600
This header indicates that a response can be cached and is valid for one hour.
Why It Matters:
By employing caching, you reduce latency and server load, which enhances the overall user experience.
For deeper insights on caching strategies in APIs, check out this guide.
Using Synchronous Calls
Asynchronous vs. Synchronous
Synchronous API calls force the client to wait for a response before proceeding. This can lead to poor performance, especially if the API requires multiple service calls.
Better Approach:
Using asynchronous calls enables non-blocking requests, allowing the application to continue processing. In Java, you can utilize CompletableFuture for this.
Example:
import java.util.concurrent.CompletableFuture;
public CompletableFuture<User> fetchUser(int userId) {
return CompletableFuture.supplyAsync(() -> {
// Simulate API call
return apiClient.getUser(userId);
});
}
Why Use Asynchronous Calls:
Asynchronous API calls help to improve responsiveness and can dramatically enhance user experience, especially in front-end applications where delays can be noticeable.
Neglecting Error Handling
The Importance of Error Handling
Errors are inevitable in programming. An API without proper error handling can leave clients in the dark when something goes wrong.
How to Handle Errors:
Define clear error responses that include useful information. Here's an example:
{
"error": {
"code": "404",
"message": "User not found"
}
}
Why It Matters:
Clear, informative error messages help developers diagnose issues quickly. This leads to faster resolutions and improves the overall reliability of your API.
The Last Word
Designing a well-performing API is essential for any modern application. Avoiding common pitfalls such as not versioning, under-fetching or over-fetching, ignoring caching, using synchronous calls, and neglecting error handling can significantly enhance your API’s performance. By taking a proactive approach in these areas, you provide your users with a fast, efficient, and reliable service.
Further Reading
For those interested in deepening their understanding of API design best practices, consider exploring:
- API Design Best Practices: A Complete Guide
- RESTful API Design: A Beginner’s Guide
By employing these strategies and understanding the rationale behind them, you can create APIs that not only meet business requirements but also provide optimal performance for end-users.