Common Pitfalls When Checking Palindromes in Java
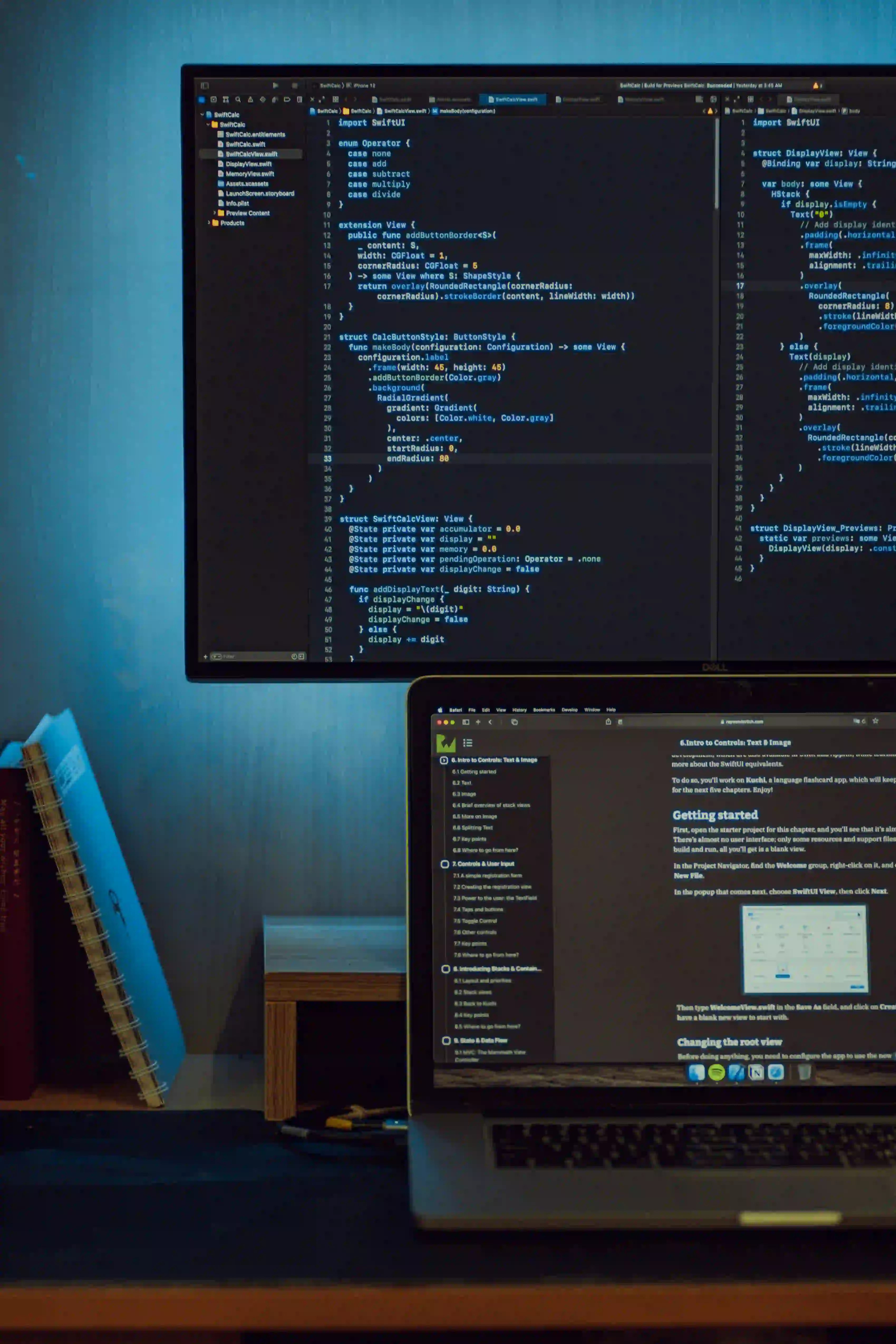
Common Pitfalls When Checking Palindromes in Java
When programming, few tasks are as intriguing and deceptively simple as checking if a word or phrase is a palindrome. A palindrome is a word, phrase, number, or sequence that reads the same backward as forward. Classic examples include "radar" and "level." In this post, we'll explore common pitfalls developers encounter when implementing palindrome checks in Java, along with effective solutions and best practices.
What is a Palindrome?
Before diving into the pitfalls, let's clarify what constitutes a palindrome. A basic definition is straightforward: a string is a palindrome if it remains unchanged when reversed. However, palindromes can get complex when you factor in spaces, punctuation, and case sensitivity.
Examples of Palindromes:
- Words: "madam", "racecar"
- Phrases: "A man, a plan, a canal, Panama!"
- Numbers: 121, 12321
Common Pitfalls in Palindrome Checks
1. Ignoring Case Sensitivity
A common mistake is overlooking the importance of case when checking for palindromes. Specifically, "Madam" and "madam" should be treated the same. To handle this, we should convert the string to either all upper case or all lower case.
public boolean isPalindrome(String str) {
String cleanString = str.toLowerCase(); // Normalize case
String reversedString = new StringBuilder(cleanString).reverse().toString();
return cleanString.equals(reversedString);
}
In the above code, converting the input string to lower case allows us to compare it without worrying about mismatched casing.
2. Ignoring Spaces and Punctuation
When checking palindromes, we often assume that only alphanumeric characters matter. However, spaces and punctuation can interfere with our results. To solve this, we can use regex to filter out non-alphanumeric characters.
public boolean isPalindrome(String str) {
String cleanString = str.replaceAll("[^a-zA-Z0-9]", "").toLowerCase(); // Clean input
String reversedString = new StringBuilder(cleanString).reverse().toString();
return cleanString.equals(reversedString);
}
In this code, we use replaceAll
with a regex pattern to keep only alphanumeric characters, enhancing the function's robustness.
3. Inefficiency in String Manipulation
String manipulation in Java can be costly. The above examples create new strings, which can impact performance for long inputs. Instead of creating multiple strings, we can use two-pointer technique, checking characters from both ends of the cleaned string.
public boolean isPalindrome(String str) {
String cleanString = str.replaceAll("[^a-zA-Z0-9]", "").toLowerCase();
int left = 0;
int right = cleanString.length() - 1;
while (left < right) {
if (cleanString.charAt(left) != cleanString.charAt(right)) {
return false; // Found a mismatch
}
left++;
right--;
}
return true; // It's a palindrome
}
Here, we improve efficiency by eliminating excess string creation and directly comparing characters. This significantly reduces memory overhead.
4. Neglecting Edge Cases
Many developers forget to handle edge cases, such as empty strings or single-character strings. Both are technically palindromes. Incorporating these checks can help ensure the integrity of our function.
public boolean isPalindrome(String str) {
if (str == null || str.isEmpty()) return true; // Handle empty string & null
String cleanString = str.replaceAll("[^a-zA-Z0-9]", "").toLowerCase();
int left = 0;
int right = cleanString.length() - 1;
while (left < right) {
if (cleanString.charAt(left) != cleanString.charAt(right)) {
return false; // Found a mismatch
}
left++;
right--;
}
return true; // It's a palindrome
}
By checking for null or empty strings, we create a more robust and user-friendly function.
5. Overcomplicating the Solution
Sometimes, developers tend to overthink the problem, introducing unnecessary complexity. A straightforward approach is typically the best. The two-pointer technique described earlier strikes a balance between clarity and efficiency.
Example: Full Palindrome Checker
Let's combine all the solutions discussed. Below is a full implementation of a palindrome checker that is robust, efficient, and straightforward.
public class PalindromeChecker {
public boolean isPalindrome(String str) {
if (str == null || str.isEmpty()) return true; // Handle empty or null input
String cleanString = str.replaceAll("[^a-zA-Z0-9]", "").toLowerCase(); // Clean input
int left = 0;
int right = cleanString.length() - 1;
while (left < right) {
if (cleanString.charAt(left) != cleanString.charAt(right)) {
return false; // Mismatch found
}
left++;
right--;
}
return true; // It's a palindrome
}
public static void main(String[] args) {
PalindromeChecker checker = new PalindromeChecker();
String[] testStrings = {"A man, a plan, a canal, Panama!", "No lemon, no melon", "Madam", "Hello"};
for (String test : testStrings) {
System.out.println(test + " -> " + checker.isPalindrome(test));
}
}
}
Explanation of the Code
- Input Handling: The function checks for null and empty strings.
- String Cleaning: Non-alphanumeric characters are removed, and the string is converted to lower case.
- Two-Pointer Approach: It compares characters from both ends, moving towards the center.
- Main Method: The
main
method demonstrates usage with various test cases.
Final Considerations
Checking for palindromes in Java may seem trivial at first, but it involves pitfalls that can lead to incorrect results or inefficient performance. By taking care of case sensitivity, cleaning inputs, and avoiding unnecessary complexity, we can create an efficient algorithm that handles various edge cases gracefully.
For more information on strings in Java, check out the Oracle documentation.
Now, go ahead and try implementing your own palindrome checker while avoiding these common pitfalls! Happy coding!