Common Pitfalls When Starting with Serverless Java Functions
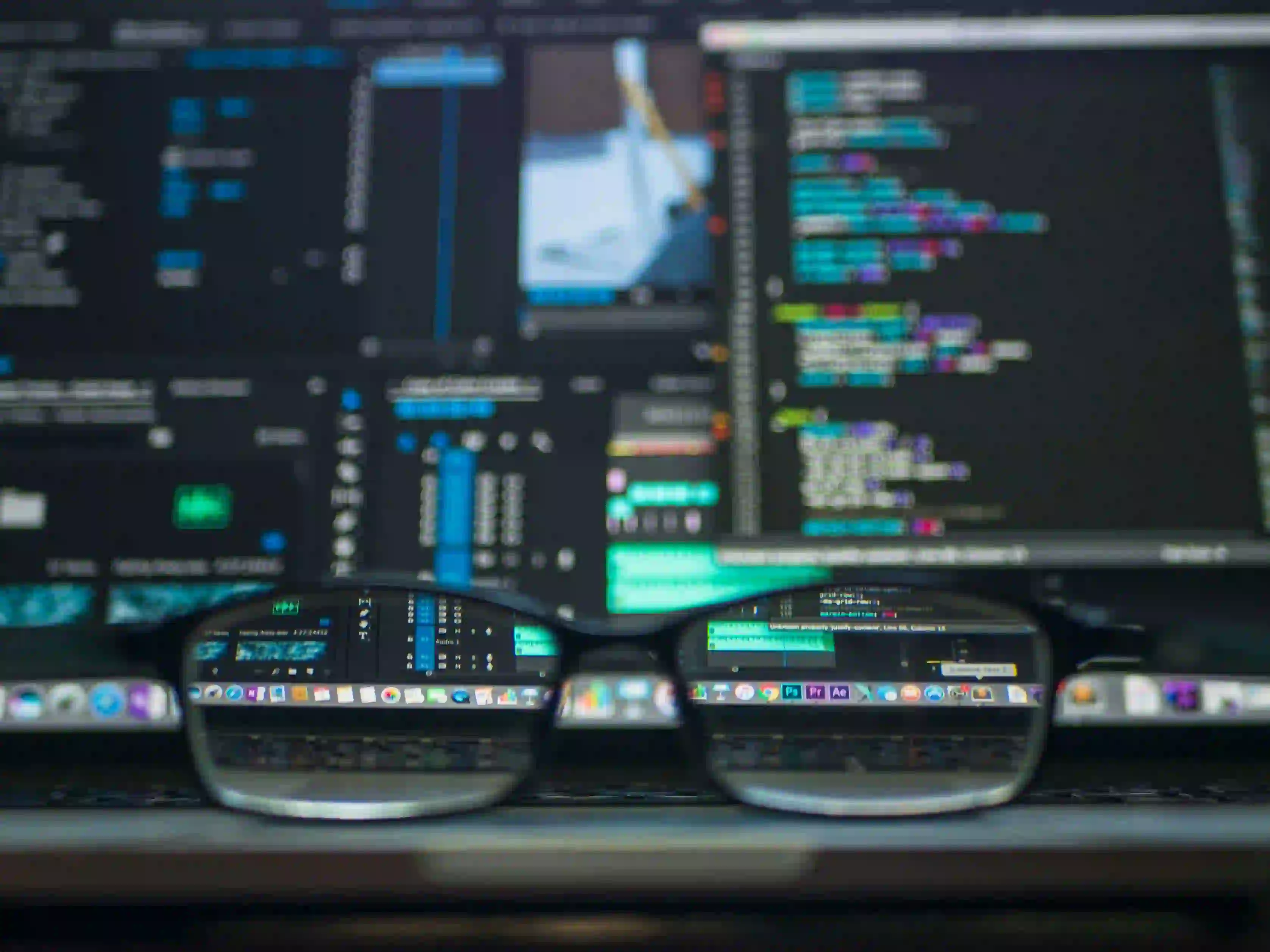
Common Pitfalls When Starting with Serverless Java Functions
Serverless computing has transformed the way developers approach application architecture. With platforms like AWS Lambda, Azure Functions, and Google Cloud Functions, developers can focus on writing code without worrying about infrastructure management. Java, a language known for its robustness and reliability, is increasingly being used in serverless environments. However, there are common pitfalls that Java developers encounter when diving into serverless functions. In this blog post, we will explore these pitfalls, helping you navigate the landscape and develop effective serverless applications.
Understanding the Serverless Architecture
Before we dive into common pitfalls, it’s important to understand the basic principles of serverless computing. In a serverless architecture:
- Event-driven: Functions are triggered by events (HTTP requests, database updates, etc.).
- Stateless: Functions do not maintain state between executions. Each invocation is independent.
- Managed Infrastructure: The cloud service provider handles the scaling and management of infrastructure.
With Java’s strong ecosystem, you can effectively leverage serverless models. However, you should tread carefully to avoid common mistakes.
1. Improper Cold Start Management
The Problem
Java applications are notoriously heavy when it comes to cold starts. Cold starts occur when a function is invoked for the first time or after a period of inactivity, requiring the cloud provider to create a new instance of the service. This delay can lead to slower response times and a poor user experience.
The Solution
-
Optimize Package Size: Minimize the size of your deployment package. Use tools like ProGuard to strip unused classes, fields, and methods from your code.
-
Keep Functions Warm: Implement a warming strategy to periodically invoke your functions. For example, you could use scheduled events or a simple cron job to reduce cold starts.
Example Code
public class WarmingFunction {
public void handleRequest(ScheduledEvent event, Context context) {
context.getLogger().log("Warming up the function!");
// Function execution logic goes here.
}
}
In this snippet, we've created a function that can be scheduled to run at regular intervals. This approach ensures that the Java function stays warm, minimizing cold starts.
2. Resource Management and Limits
The Problem
Serverless functions come with predefined resource limits (memory, execution duration). Java applications can consume a significant amount of memory and have longer execution times, leading to unexpected errors.
The Solution
-
Provision Resources Wisely: Avoid assuming defaults. Monitor your application and tune memory settings in your serverless provider's console.
-
Efficient Code Practices: Optimize your code to use less memory and execute within the allocated time. For instance, avoid unnecessary object creation.
Example Code
public class EfficientFunction {
public String handleRequest(String input, Context context) {
StringBuilder result = new StringBuilder();
for (char character : input.toCharArray()) {
result.append(character);
}
return result.toString();
}
}
This sample demonstrates a more memory-efficient way to handle string concatenation by using a StringBuilder
, which is better than string concatenation for larger inputs.
3. Error Handling and Debugging Challenges
The Problem
Error handling in serverless functions can be complex. Since functions are event-driven, exceptions might not propagate in an intuitive way, leading to difficulties in debugging.
The Solution
-
Centralized Error Handling: Implement a consistent error handling strategy. Create an error response structure and log errors effectively.
-
Use Monitoring Tools: Utilize logging and monitoring tools like AWS CloudWatch or Google Stackdriver to trace errors and performance issues in your serverless functions.
Example Code
public class ErrorHandlingFunction {
public String handleRequest(String input, Context context) {
try {
// Main function logic
return processInput(input);
} catch (Exception e) {
context.getLogger().log("Error processing request: " + e.getMessage());
return "Error occurred while processing your request.";
}
}
private String processInput(String input) {
// Simulated potential error.
if (input == null) {
throw new IllegalArgumentException("Input cannot be null");
}
return "Processed: " + input;
}
}
This code snippet demonstrates a simple error handling mechanism within the serverless function. It ensures that all exceptions are logged and an easy-to-understand error message is returned.
4. Overlooking Configuration Management
The Problem
Configuration management can be cumbersome in serverless applications. Hardcoding configuration parameters into your Java application can lead to issues in different deployment environments (development, staging, production).
The Solution
-
Use Environment Variables: Store configuration settings in environment variables to keep the application flexible across multiple environments.
-
Configuration Management Tools: Consider using tools like Spring Cloud Config to externalize configuration management.
Example Code
public class ConfigurableFunction {
private final String dbUrl = System.getenv("DB_URL");
public String handleRequest(String input, Context context) {
context.getLogger().log("Connecting to DB at: " + dbUrl);
// Database logic using dbUrl
return "Successfully connected to the database.";
}
}
With this approach, the database URL is set as an environment variable, allowing for changes without modifying the codebase.
5. Ignoring Scalability Considerations
The Problem
Java applications can struggle with scalability if designed without consideration of the serverless model. Heavy computational tasks, for instance, can lead to resource exhaustion.
The Solution
-
Break Down Tasks: Decompose complex operations into smaller, manageable serverless functions. Utilize asynchronous processing where possible.
-
Batch Processing: Consider batch processing of events instead of single processing, which can improve scalability.
Example Code
public class BatchProcessingFunction {
public void handleRequest(List<String> inputs, Context context) {
inputs.parallelStream().forEach(input -> {
// Process each input asynchronously
context.getLogger().log("Processing: " + input);
// Your processing logic
});
}
}
By utilizing Java Streams, we can process multiple inputs concurrently, improving performance and scalability.
In Conclusion, Here is What Matters
Navigating the ocean of serverless computing with Java can be daunting. However, by being aware of common pitfalls and employing strategic solutions, you can optimize your serverless functions and provide a seamless user experience. Always remember to monitor and adapt your serverless applications to the usage patterns and specific workloads they face. The combination of Java's versatility and the power of serverless architecture can lead to incredibly efficient and scalable applications.
For those looking to deepen their understanding of serverless architecture and Java development, consider checking out the Serverless Java Framework for practical implementation and best practices. Embrace the serverless revolution, and you'll find that you can build applications faster and more efficiently than ever before!