Overcoming SessionStorage Limits for Tab Data Sharing
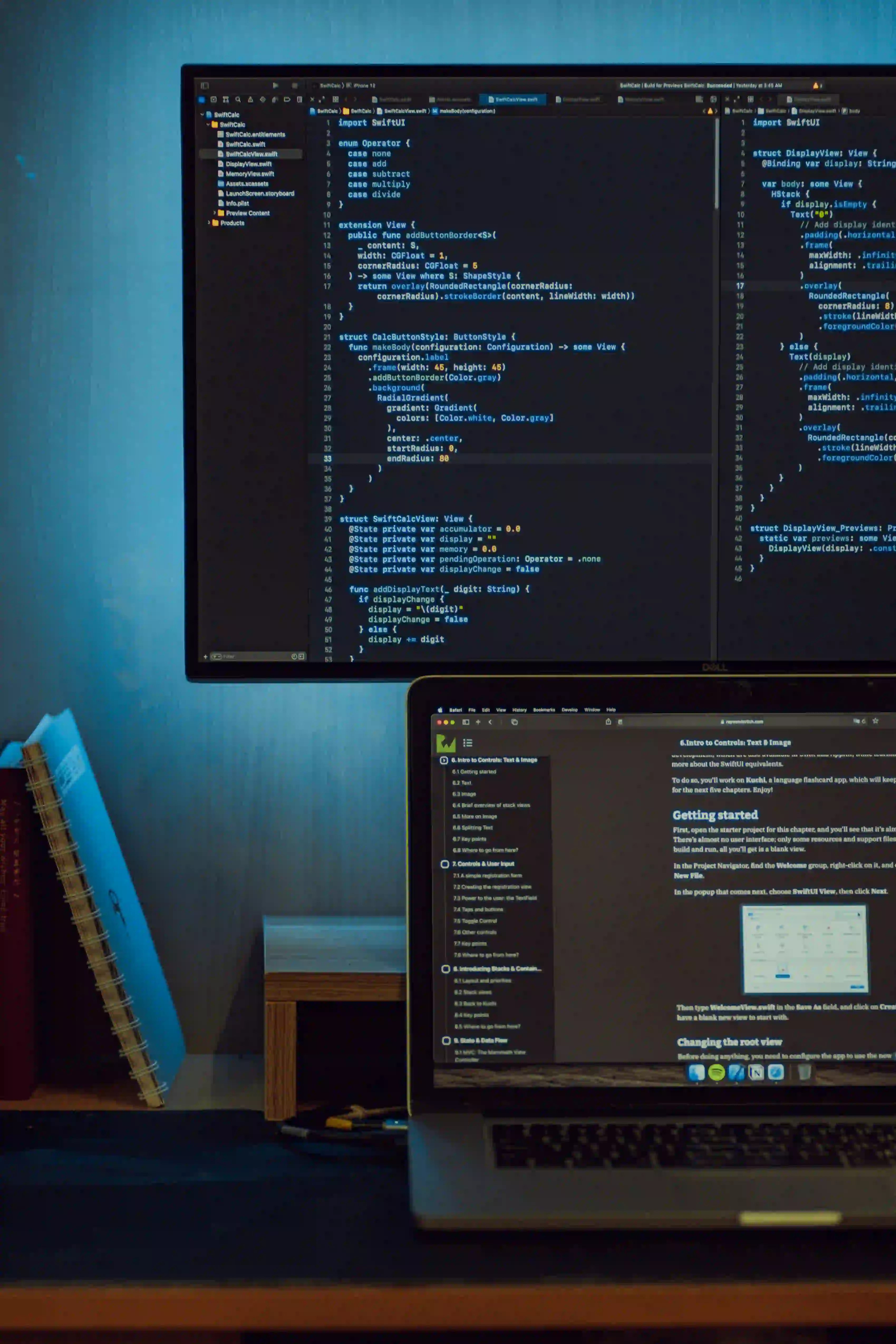
Overcoming SessionStorage Limits for Tab Data Sharing
In modern web applications, sharing data between tabs or windows remains a common need. However, there can be limitations when using certain storage methods, such as SessionStorage
, primarily because it is confined to the same tab. This means, by design, data saved in one tab cannot be accessed by another tab, even if they belong to the same domain. Fortunately, there are strategies to overcome these limitations. In this blog post, we will explore various methods to efficiently manage tab data sharing while maintaining a seamless user experience.
Understanding SessionStorage
Before diving into the solutions, it’s important to understand how SessionStorage
works. This web storage feature allows you to store data for the duration of the page session. Data persists as long as the tab or the browser window is open. Once the tab is closed, the data is cleared.
// Setting a session storage item
sessionStorage.setItem('key', 'value');
// Retrieving a session storage item
const value = sessionStorage.getItem('key');
// Removing a session storage item
sessionStorage.removeItem('key');
Limitations of SessionStorage
-
Single Tab Accessibility: Each tab has its unique instance of
SessionStorage
. This means that data saved in one tab cannot be directly accessed in another tab. -
Data Size Limit: Browsers typically impose a limit on the storage capacity (usually around 5MB). While this is sufficient for many use cases, more complex applications may require additional storage solutions.
-
No Cross-Tab Communication: Changes made in
SessionStorage
in one tab do not automatically inform any other open tabs.
Options for Tab Data Sharing
To overcome the limitations of SessionStorage
, there are various strategies that you can employ:
1. Using LocalStorage with BroadcastChannel API
An effective way to share data across tabs is using LocalStorage
in combination with the BroadcastChannel API
. This method allows different tabs to communicate with each other seamlessly.
- Store data in LocalStorage.
- Listen for messages from other tabs via BroadcastChannel.
- Update the local storage value when notified.
Here’s how you might implement this:
// Create a BroadcastChannel
const channel = new BroadcastChannel('tab-channel');
// Setting data in LocalStorage and broadcasting it
function setItem(key, value) {
localStorage.setItem(key, value);
channel.postMessage({ key, value });
}
// Listen for messages from other tabs
channel.onmessage = function(event) {
const { key, value } = event.data;
localStorage.setItem(key, value);
// Potentially update your UI or handle the new value
console.log(`Received update: ${key} = ${value}`);
};
// Example usage
setItem('username', 'john_doe');
In the code above, we create a BroadcastChannel
to facilitate communication between tabs. When we set an item in LocalStorage
, we also broadcast a message to any other open tabs. This way, we can maintain updated state across different tabs with minimal effort.
2. Using Cookies
Another way to share data is through cookies. Cookies are accessible across tabs in the same domain. However, they come with size and performance limitations compared to other methods. Cookies also require more complex management regarding expiry and scopes.
// Create a cookie
function setCookie(name, value, days) {
let expires = "";
if (days) {
const date = new Date();
date.setTime(date.getTime() + (days * 24 * 60 * 60 * 1000));
expires = "; expires=" + date.toUTCString();
}
document.cookie = name + "=" + (value || "") + expires + "; path=/";
}
// Read a cookie
function getCookie(name) {
const nameEQ = name + "=";
const ca = document.cookie.split(';');
for (let i = 0; i < ca.length; i++) {
let c = ca[i];
while (c.charAt(0) === ' ') c = c.substring(1, c.length);
if (c.indexOf(nameEQ) === 0) return c.substring(nameEQ.length, c.length);
}
return null;
}
// Example usage
setCookie('sessionToken', '123456', 1);
console.log(getCookie('sessionToken'));
While using cookies, it's crucial to note their size limitation (typically around 4KB), making them suitable mainly for smaller data items.
3. Utilizing Server-Side Storage
For applications that require sharing larger amounts of data or more complex data structures, server-side storage becomes essential. Here’s how you can implement this:
- On data change, send information via AJAX to the server.
- Server saves the data and ensures it's associated with a user session.
- Other tabs periodically fetch the latest data from the server or listen for specific events through techniques such as WebSockets or Server-Sent Events.
fetch('/updateData', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ key: 'username', value: 'john_doe' })
});
4. Leveraging WebSockets
WebSockets offer a powerful method for real-time communication between the server and multiple tabs. When user activity occurs in one tab, an event can trigger a WebSocket message that notifies all connected clients (including other tabs) to update their state.
const socket = new WebSocket('ws://yourserver.com/socket');
// When the socket is open, send a message
socket.onopen = function() {
socket.send(JSON.stringify({ key: 'username', value: 'john_doe' }));
};
// Receiving messages from the socket
socket.onmessage = function(event) {
const data = JSON.parse(event.data);
// Update your tab's local state based on the received data
console.log(`Received update: ${data.key} = ${data.value}`);
};
WebSocket connections provide a persistent connection that can be ideal for applications requiring frequent and real-time data updates.
Key Takeaways
In summary, while SessionStorage
is a straightforward option for managing data within a single tab, it does not cater to scenarios requiring data sharing across multiple tabs. By utilizing methods like LocalStorage
, BroadcastChannel API
, cookies, server-side storage, and WebSockets, you can build a robust solution that overcomes these limitations.
For more foundational knowledge on web storage APIs, you can explore the MDN documentation on Web Storage and learn about WebSockets.
By employing these methods, you'll not only enhance user experience but also create a more connected and functional web application. Choose the method that best fits your application needs, and empower your users with a responsive and resourceful experience!
Feel free to reach out if you have any questions or need further clarifications on any of these methods! Happy coding!