How to Continue a Gradle Build Despite Failed Tasks
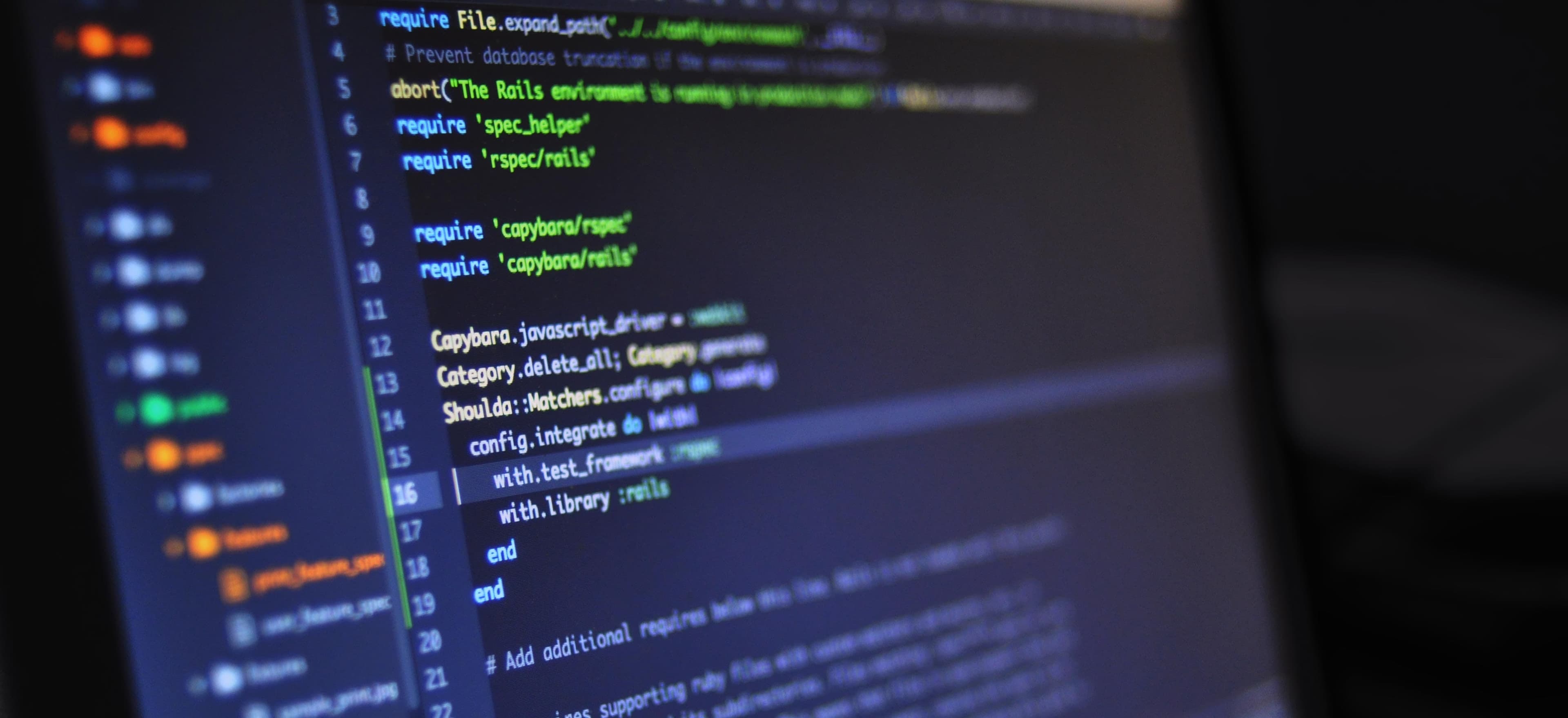
- Published on
How to Continue a Gradle Build Despite Failed Tasks
Gradle is a powerful build tool widely used in Java development. It allows you to automate the tasks involved in building, testing, and deploying your code. However, there may be scenarios during the development process where a particular task fails, and the entire build halts. This can be frustrating, especially if you want to see the results of subsequent tasks. Fortunately, Gradle provides a way to continue the build process despite failed tasks. In this post, we will explore how to achieve this and delve into the implications of doing so.
Understanding Gradle Builds
Before we dive into the specifics, it is important to understand the structure of a Gradle build. Typically, a Gradle build consists of a series of tasks defined in a build script (usually named build.gradle
). These tasks can depend on one another and can represent various phases of the build process, such as compiling code, running tests, or generating documentation.
Here’s a simple Gradle build script example to illustrate:
plugins {
id 'java'
}
repositories {
mavenCentral()
}
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-web'
testImplementation 'org.junit.jupiter:junit-jupiter:5.7.0'
}
tasks.test {
useJUnitPlatform()
}
In this script:
- We apply the Java plugin to configure the project for Java development.
- We define a central repository to fetch dependencies.
- We specify dependencies for the main application and its tests.
The Problem: Failed Tasks in a Build
When a task fails during the Gradle build, the default behavior is to stop the build process entirely. While this is useful under normal circumstances (you want to know if something is wrong), it can hinder your ability to quickly iterate on your code, especially if subsequent tasks do not depend on the outcome of the failed task.
Example of Task Failure
Imagine running the gradle build
command where the tests fail due to a non-critical issue:
> Task :test FAILED
FAILURE: Build failed with an exception.
* What went wrong:
Execution failed for task ':test'.
After this message, you might be unable to execute packaging or deployment tasks that could still succeed.
Continuing the Build Despite Task Failures
To continue the build even when certain tasks fail, you can use the following methods:
1. Command-Line Option
The simplest way to bypass task failures is by using the --continue
command-line option:
gradle build --continue
This tells Gradle to ignore failures in individual tasks and continue executing as many tasks as possible. This is particularly useful when you want to perform other operations that do not depend on the failed task(s).
2. Configuring the Build Script
You can also specify this behavior directly in your build.gradle
file by setting the continueOnFailure
property for specific tasks or for the entire build. Here’s how to do it:
tasks.withType(Test) {
ignoreFailures = true
}
In this example, if any of the test cases fail, the build will still continue to other tasks, but the outputs will indicate where the failures occurred.
3. Considerations for Using Ignore Failures
While it may be tempting to ignore failures, you should proceed with caution. Here are some reasons to be judicious:
- Debugging Difficulties: A build that continues despite failures can lead to confusion and make it harder to detect and fix issues.
- Quality Concerns: If you are deploying code that has known issues, this can lead to instability in production environments.
Reporting Failures
If you choose to continue on failure, consider generating a report to keep track of the failed tasks. You can achieve this by using Gradle's built-in reporting capabilities:
task reportFailures {
doLast {
if (state.failure) {
println "Task '${name}' failed."
}
}
}
tasks.withType(Test).all {
finalizedBy reportFailures
}
This script creates a new task called reportFailures
, which tracks if any test tasks failed. By running this report, you can maintain visibility on what actually went wrong during the build process.
Bringing It All Together
Continuing a Gradle build despite failed tasks can significantly improve your development workflow, especially when working on large projects. However, it is vital to use this feature responsibly and to ensure that you address the underlying issues causing the failures.
If you want to deepen your understanding of Gradle, consider checking out the official Gradle documentation and Gradle best practices for optimizing your build process.
In summary, while Gradle’s --continue
option is a handy tool to help you overcome task failures, always balance the benefits against the need for quality and stability in your projects. Happy coding!