Unlocking Application Performance: Common Bottlenecks Explored
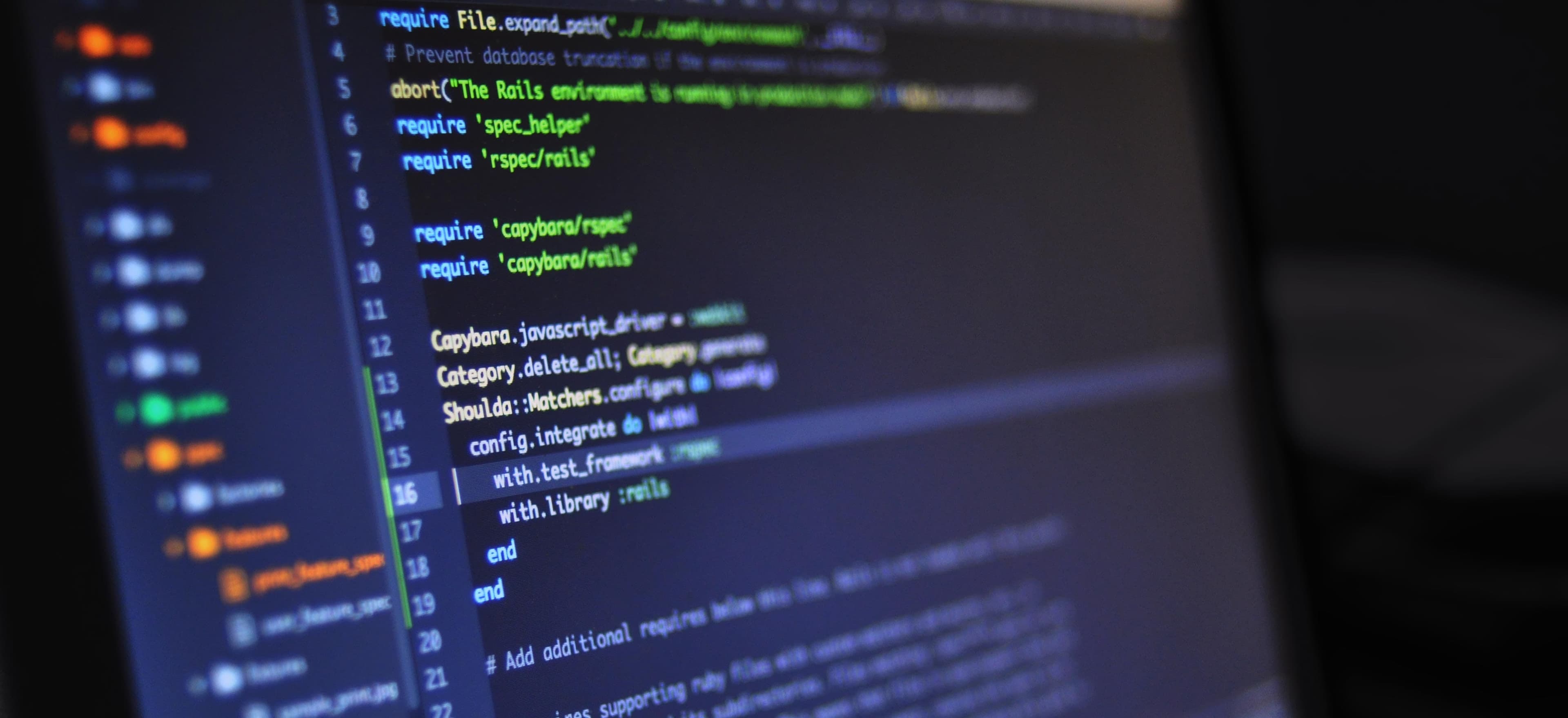
- Published on
Unlocking Application Performance: Common Bottlenecks Explored
Application performance can make or break the user experience. In this blog post, we will delve into common performance bottlenecks that developers encounter in their applications. We'll explore their causes, implications, and most importantly, how to resolve them. By the end of this post, you will have a toolkit of strategies to enhance your application's performance effectively.
Understanding Performance Bottlenecks
A performance bottleneck occurs when a certain component of an application restricts the overall flow of operations. This confinement can lead to slow response times, unresponsive interfaces, and even application crashes. To unlock your application's potential, it is crucial to identify and mitigate these bottlenecks.
Types of Performance Bottlenecks
Bottlenecks can typically be categorized into three key areas:
- CPU Bottlenecks
- I/O Bottlenecks
- Database Bottlenecks
Each category has its unique indicators and solutions.
1. CPU Bottlenecks
Symptoms
- High CPU utilization
- Slow processing times
- Increased latency
Causes
CPU bottlenecks often occur due to complex computations or inefficient algorithms. When the CPU is overwhelmed by processing tasks, it cannot handle additional requests, leading to degraded performance.
Identifying and Resolving CPU Bottlenecks
To identify CPU bottlenecks, you can use performance profiling tools like Java Mission Control or VisualVM. These tools provide insights into thread behavior and execution time.
Here’s a simple code example of handling an operation using concurrency to alleviate CPU bottlenecks:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class CPUBottleneckExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(Runtime.getRuntime().availableProcessors());
for(int i = 0; i < 100; i++) {
executor.submit(() -> {
performHeavyCalculation();
});
}
executor.shutdown();
// Await termination or handle thread completion
}
private static void performHeavyCalculation() {
// A simulation of complex computations
for (int i = 0; i < 1000000; i++) {
Math.sqrt(i);
}
}
}
Why? Using a thread pool optimizes CPU usage by allowing multiple threads to run concurrently, thereby utilizing the available CPU resources effectively.
2. I/O Bottlenecks
Symptoms
- Slow data retrieval
- High disk usage
- Network delays
Causes
I/O bottlenecks often arise from slow disk operations, inefficient file handling, or suboptimal network calls. If your application spends too much time waiting for I/O operations to complete, it will impact overall performance.
Identifying and Resolving I/O Bottlenecks
To spot I/O bottlenecks, you can analyze logs or use monitoring tools like New Relic and Prometheus.
Here’s an example of optimizing file reading operations:
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.List;
public class IOBottleneckExample {
public static void main(String[] args) {
try {
List<String> lines = readFile("example.txt");
lines.forEach(System.out::println);
} catch (Exception e) {
e.printStackTrace();
}
}
private static List<String> readFile(String filePath) throws Exception {
return Files.readAllLines(Paths.get(filePath));
}
}
Why? Using NIO (New I/O) APIs like those in java.nio.file
enhances performance by providing asynchronous processing capabilities, reducing wait times for file operations.
3. Database Bottlenecks
Symptoms
- Slow database queries
- Increased connection times
- Frequent timeouts or errors
Causes
Database bottlenecks can arise from poor query design, insufficient indexing, or high concurrent user loads. An improperly managed database can significantly hinder application performance.
Identifying and Resolving Database Bottlenecks
Using database monitoring tools such as MySQL Workbench or pgAdmin can help identify slow queries.
Here is an example of optimizing a SQL query with indexing:
CREATE INDEX idx_user_email ON users(email);
Why? Creating an index on the email field enhances query performance, allowing the database engine to quickly locate user records based on email addresses.
Best Practices for Performance Optimization
Code Optimization
Always look for opportunities to optimize your code. This includes:
- Minimizing the use of expensive operations.
- Reducing loops and unnecessary calculations.
- Caching frequently accessed data.
Monitoring and Profiling
Continuous monitoring is key to identifying bottlenecks early on. Using tools and services such as:
will help you actively track performance metrics, allowing you to detect anomalies swiftly.
Load Testing
Before deploying applications, conduct load tests to ensure performance under various user loads. Tools like Apache JMeter or Gatling can help simulate real-world traffic conditions.
Database Optimization
Keep your database in an optimal state by:
- Regularly updating statistics.
- Implementing connection pooling.
- Using prepared statements to enhance performance.
Final Thoughts
Performance bottlenecks can significantly impact the user experience of your application. By understanding the common types of bottlenecks, identifying their causes, and implementing effective resolutions, you can ensure that your application's performance remains robust and resilient.
For further reading, consider exploring:
- Java Performance Tuning
- Effective Java
With proper monitoring, optimization, and the right tools at your disposal, you will be well-equipped to enhance the performance of your applications and deliver a stellar user experience. Happy coding!