Why Packaging by Features Improves Code Maintainability
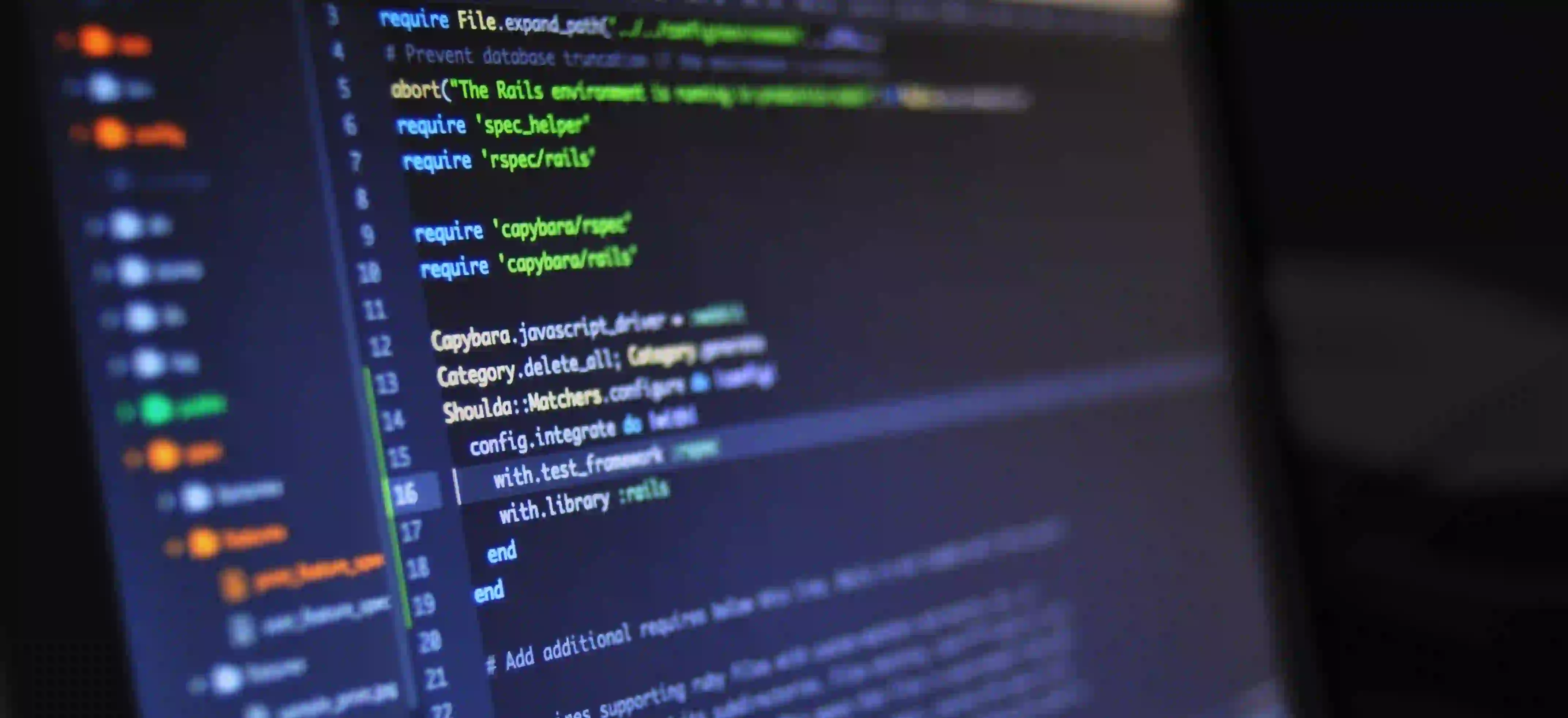
Why Packaging by Features Improves Code Maintainability
In the ever-evolving landscape of software development, maintaining code quality and project longevity has become a critical concern. One approach that has shown significant promise in enhancing code maintainability is packaging by features. This method groups related functionalities together, enabling developers to modify and manage code more efficiently. In this post, we will dive into the concept of packaging by features, illustrate its relevance with code snippets, and highlight why it's a superior method for organizing your codebase.
Understanding the Concept
The Traditional Approach
Traditionally, developers often organized their code by layers or components, such as controllers, models, and views. This layered approach can become cluttered as the code base scales, making it challenging to locate features and modifications. Consider a typical MVC (Model-View-Controller) architecture:
// Traditional MVC Organization
// UserModel.java (Model)
public class UserModel {
public String getUsername() {
// implementation
}
}
// UserController.java (Controller)
public class UserController {
private UserModel userModel;
public void displayUser() {
// implementation
}
}
// UserView.java (View)
public class UserView {
public void printUserDetails(String userName) {
// implementation
}
}
In this setup, each class has a specific responsibility, but when we need to implement an enhancement that affects both the controller and the view, navigating through multiple files can quickly become cumbersome.
Packaging by Features: A Modern Solution
Packaged by features, however, organizes code around functional units - each feature is encapsulated with its related components. This approach not only enhances readability but also fosters improved maintainability. Here is a restructured example:
// UserFeaturePackage
public class UserModel {
public String getUsername() {
// implementation
}
}
public class UserController {
private UserModel userModel;
public void displayUser() {
System.out.println("Displaying user: " + userModel.getUsername());
}
}
public class UserView {
public void printUserDetails(String userName) {
System.out.println("User Name: " + userName);
}
}
Why This Matters
By grouping all components related to user functionalities—model, controller, and view—into a single package, developers can easily locate and edit features. If we need to add a new feature, like user authentication, we can do it in one cohesive area without digging through various folders.
The Benefits of Packaging by Features
1. Improved Readability
One of the most immediate benefits of packaging by features is improved readability. When all classes and resources for a specific feature are in one location, it's straightforward for new developers (or the original author after some time) to understand existing features. They do not have to jump between different packages or modules and can often gain a comprehensive understanding of a feature by looking in one place.
2. Easier Modifications
When working on a feature, any necessary adjustments span across a limited number of files. For example, if you want to change the representation of user information, you'd only need to modify UserModel
and UserView
without searching for a corresponding controller or other unrelated files. This greatly reduces the risk of breaking unrelated functionality.
3. Promotes Scalability
As projects grow, features can become more complex. Organizing by features allows developers to quickly identify and isolate specific functionalities that need more granular attention or scaling without affecting other parts of the system.
4. Facilitates Collaboration
Working in teams often means multiple developers will touch different parts of a project. If your project is organized by features, team members can more easily collaborate without stepping on each other's toes. Developers can work in parallel on different features without causing merge conflicts in unrelated areas.
5. Improved Testing
Each feature can be treated as an independent unit. Using feature packages allows easier integration of unit tests that focus solely on the functionality contained within that package. Separate tests for a user feature can be efficiently executed without interference from other tests.
Example of Feature Testing
Let’s take a look at a simple unit test for our user feature:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class UserModelTest {
@Test
public void testGetUsername() {
UserModel userModel = new UserModel();
userModel.setUsername("JohnDoe");
assertEquals("JohnDoe", userModel.getUsername());
}
}
In this test, we can focus solely on validating the functionality of UserModel
without worrying about other unrelated parts of the system, thanks to the organizational structure fostered by packaging by features.
Challenges and Considerations
Despite its clear advantages, packaging by features is not without challenges:
-
Initial Refactoring: Transitioning from a traditional layered structure to a feature-based one often requires significant refactoring. This process can introduce temporary misalignment in code organization.
-
Deeply Related Features: Sometimes, features may share components that do not fit neatly into the packaging concept. Careful thought must be applied to how overlapping functionalities should be organized.
-
Team Familiarity: Not all team members may be familiar with working in a feature-based structure, which may require additional training or practice periods.
The Closing Argument
In summary, packaging by features offers several advantages that can significantly improve code maintainability. It promotes readability, makes modifications easier, enhances scalability, and facilitates collaboration—all while simplifying testing strategies.
As software development continues to evolve, methodologies that embrace flexibility and efficiency will become increasingly important. By opting for feature-based organization, you can not only streamline your development processes but also ensure that your code remains robust and maintainable through its lifecycle.
For further reading on best practices in code organization, check out articles on Stack Overflow and Martin Fowler’s website for insightful resources on coding principles and architecture.
Incorporating these practices today will set your projects up for success in the long run!