Improving Proximity Queries in Lucene with Automatons
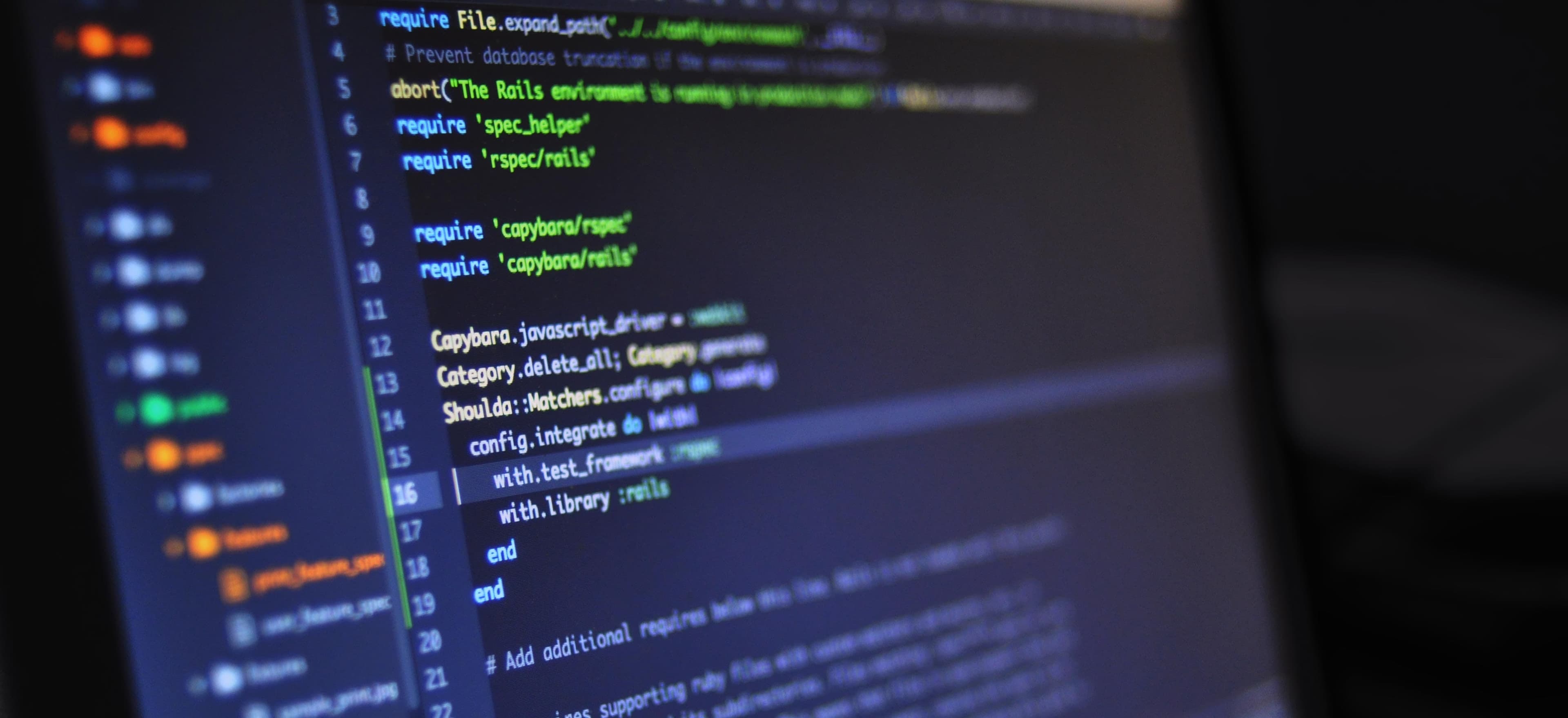
- Published on
Improving Proximity Queries in Lucene with Automatons
Proximity queries are vital in full-text search systems like Apache Lucene. They allow users to find terms that are close to each other within documents, enhancing the search experience. However, when it comes to handling large datasets, the efficiency and speed of these queries can become a significant bottleneck. In this blog post, we'll explore how using automatons can streamline proximity queries in Lucene, improving performance without sacrificing accuracy.
Understanding Proximity Queries
Before diving into optimizations, let’s clarify what proximity queries are. These queries return documents where the specified terms appear within a specific distance from each other. For example, the query "apple banana"~5
finds documents that contain the words "apple" and "banana" within five words of each other.
Why Proximity Queries Matter
- User Intent: Users expect search results that match their intentions based on proximity.
- Relevancy: Closer terms often mean more relevant results.
- Complex Phrasing: Modern search engines handle complex queries, requiring nuanced processing.
However, with increasing datasets, traditional methods of processing these queries can lead to performance issues.
Introducing Automatons
Automatons are finite state machines that can recognize patterns. These mathematical models are extremely useful in processing strings and can be integrated into Lucene to handle proximity queries more efficiently.
Benefits of Using Automatons in Lucene
- Increased Speed: With their structured approach, automatons can reduce the number of unnecessary comparisons.
- Flexibility: They can adapt dynamically to query changes while maintaining speed.
- Memory Efficiency: Automatons can compactly represent many states, reducing overhead.
How to Implement Automatons in Lucene
Now, let’s get into the practical implementation of automatons in Lucene for optimizing proximity queries.
Step 1: Setting Up Your Environment
Make sure you have Apache Lucene properly set up in your project. You can easily include the Lucene dependency in a Maven project.
<dependency>
<groupId>org.apache.lucene</groupId>
<artifactId>lucene-core</artifactId>
<version>9.4.1</version>
</dependency>
<dependency>
<groupId>org.apache.lucene</groupId>
<artifactId>lucene-queryparser</artifactId>
<version>9.4.1</version>
</dependency>
Step 2: Building the Automaton
Next, you need to build your automaton that defines the states and transitions for processing the proximity query. Lucene provides utilities for creating and managing automatons.
Here is an example of creating a simple automaton for our proximity query scenario:
import org.apache.lucene.util.automaton.Automaton;
import org.apache.lucene.util.automaton.State;
import org.apache.lucene.util.automaton.AutomatonOperations;
public class ProximityAutomaton {
public Automaton buildAutomaton(String term1, String term2, int proximity) {
State start = new State();
State term1State = new State();
State term2State = new State();
State end = new State();
// Configure the states
start.addTransition(new Transition(term1.charAt(0), term1.charAt(0), term1State));
start.addTransition(new Transition(term2.charAt(0), term2.charAt(0), term2State));
// Handle proximity
for (int i = 1; i <= proximity; i++) {
term1State.addTransition(new Transition(term2.charAt(0), term1.charAt(0), end));
term2State.addTransition(new Transition(term1.charAt(0), term2.charAt(0), end));
}
// Create the automaton
Automaton automaton = new Automaton();
automaton.addState(start);
automaton.addState(term1State);
automaton.addState(term2State);
automaton.addState(end);
automaton.setInitialState(start);
automaton.setAcceptState(end);
return automaton;
}
}
Explanation of the Code
- States: We define states for the starting point, after matching term1, after matching term2, and the end state.
- Transitions: The transitions between states represent the input characters that lead from one state to another.
- Proximity Logic: The loop considers the maximum distance allowed between term1 and term2.
Step 3: Integrating with Lucene Indexing and Searching
After building the automaton, the next step is to integrate it into your indexing and searching processes. You can utilize Lucene features to accommodate queries using your automaton with the following approach:
import org.apache.lucene.index.IndexReader;
import org.apache.lucene.index.DirectoryReader;
import org.apache.lucene.store.Directory;
import org.apache.lucene.store.RAMDirectory;
import org.apache.lucene.search.IndexSearcher;
import org.apache.lucene.search.Query;
public class ProximitySearch {
public IndexSearcher indexSearcher(Directory index) throws IOException {
IndexReader reader = DirectoryReader.open(index);
return new IndexSearcher(reader);
}
public void performSearch(IndexSearcher searcher, Automaton automaton, int k) throws IOException {
// Convert automaton to Lucene Query
Query query = new AutomatonQuery("fieldName", automaton);
TopDocs results = searcher.search(query, k);
// Output results
for (ScoreDoc scoreDoc : results.scoreDocs) {
Document doc = searcher.doc(scoreDoc.doc);
System.out.println("Found document: " + doc.get("fieldName"));
}
}
}
Explanation of the Search Implementation
- IndexSearcher: This class is essential for querying an index.
- AutomatonQuery: The automaton is converted to a Lucene query allowing tight integration with Lucene's scoring and ranking algorithms.
To Wrap Things Up
Leveraging automatons for proximity queries in Lucene can lead to significant performance improvements, especially in large datasets with complex queries. The structured representation of states and transitions allows your queries to be processed efficiently while maintaining accuracy.
Further Reading
For those interested in diving deeper, consider the following resources:
By implementing the techniques discussed here, you can enhance the search experience in your applications, leading to better relevancy and faster query responses. Happy coding!
Checkout our other articles