Common Mistakes When Testing Last Element in Java Arrays
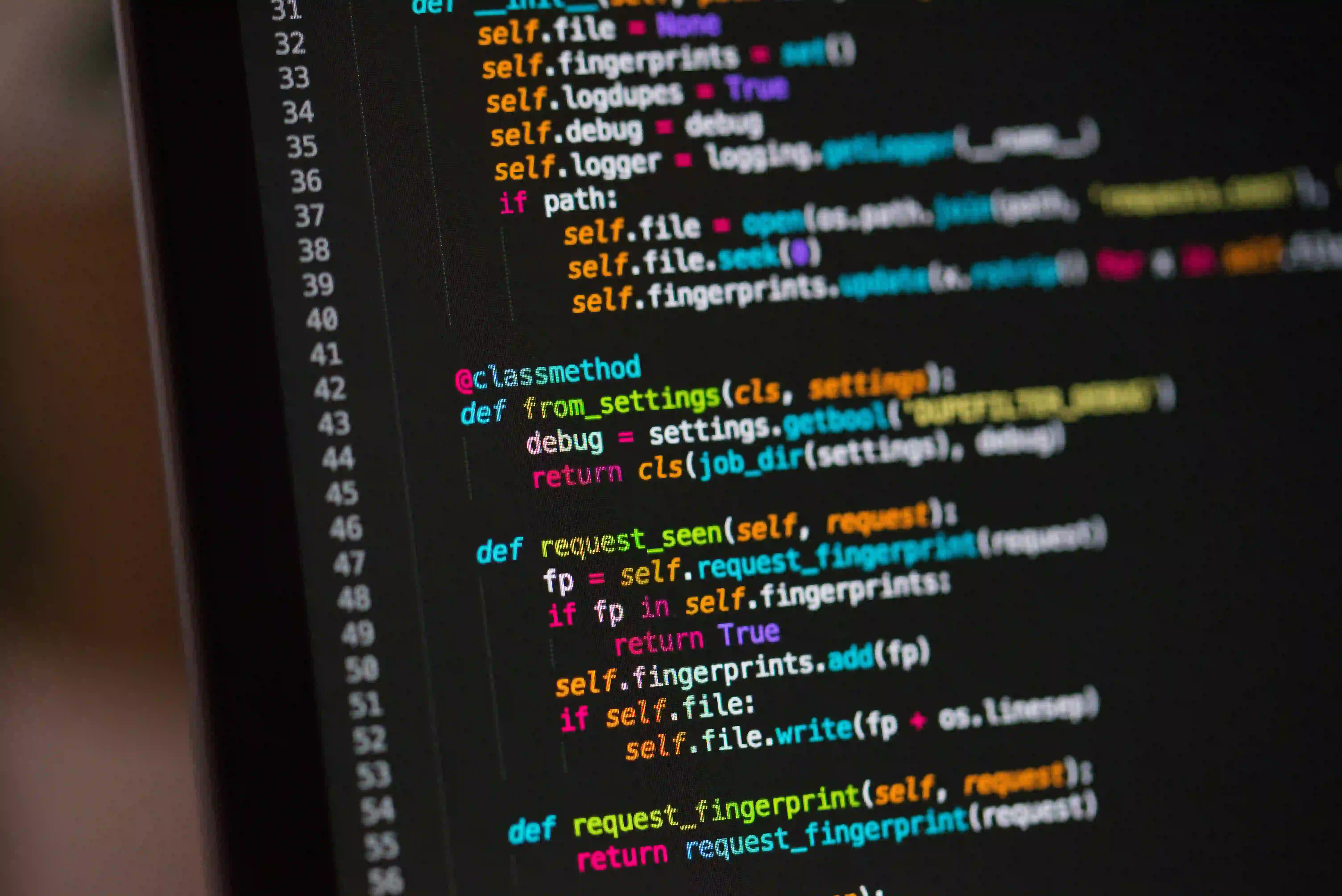
Common Mistakes When Testing Last Element in Java Arrays
Java arrays are powerful and versatile data structures, but their manipulation—especially when accessing specific elements—can lead to some common pitfalls. One of the key actions you might perform with arrays is checking the last element. This seems straightforward, yet there are traps that even seasoned developers can fall into. This blog post explores these common mistakes, providing insights and code examples to deepen your understanding.
Table of Contents
- Understanding Java Arrays
- Common Mistakes
- Off-by-One Errors
- NullPointerException
- Type Mismatch
- Not Using Length Property
- Best Practices
- Conclusion
Understanding Java Arrays
Before diving into mistakes, let’s briefly revisit how Java arrays work. In Java, an array is a contiguous block of memory that contains elements of a specific type. When you declare an array, you need to specify the type and the number of elements.
int[] numbers = new int[5]; // An array of integers with 5 elements
In the above example, the valid index range is from 0 to 4. Remember, Java uses zero-based indexing!
Common Mistakes
1. Off-by-One Errors
One of the most common mistakes occurs when developers forget that an array's index begins at zero. This can lead to an attempt to access the "last" element incorrectly.
Example:
int[] numbers = {10, 20, 30, 40, 50};
// WRONG: This will throw ArrayIndexOutOfBoundsException
int lastElement = numbers[5]; // Mistake! Length is 5, but max index is 4
// CORRECT: Accessing last element properly
int lastElement = numbers[numbers.length - 1];
System.out.println("Last element: " + lastElement); // Outputs: Last element: 50
In this illustration, the first approach leads to an ArrayIndexOutOfBoundsException
because we exceed the bounds of the array. The correct method leverages numbers.length - 1
, ensuring you access the last element safely.
2. NullPointerException
Another pitfall occurs when dealing with uninitialized arrays. If you reference an array before it gets properly initialized, you can run into a NullPointerException
.
Example:
int[] numbers = null;
try {
// Attempting to access the last element of a null array
int lastElement = numbers[numbers.length - 1];
} catch (NullPointerException e) {
System.err.println("Caught a NullPointerException: " + e.getMessage());
}
By attempting to access an element in an uninitialized (null) array, Java throws a NullPointerException
. Always ensure your array is initialized before trying to access its elements.
3. Type Mismatch
Java is a strongly typed language. If you try to store incompatible data types in an array, it can lead to unexpected results. This mistake arises more prominently in collections, but it's crucial to handle arrays correctly as well.
Example:
Object[] mixedArray = new Object[3];
mixedArray[0] = "String value"; // OK
mixedArray[1] = 42; // OK
mixedArray[2] = new int[] {1, 2}; // Not the best usage
// Accessing last element
int lastElement = (int) mixedArray[1]; // Casting needed
System.out.println("Last mixed element: " + lastElement);
In the above example, while we can store mixed types in an Object
array, attempting to cast them without proper checks or understanding can lead to ClassCastException
. Always ensure you check the type before performing operations on your variables.
4. Not Using Length Property
When dealing with arrays, some developers may forget to use the length
property. This is particularly important when passing the length as a parameter or during iteration.
Example:
int[] numbers = {1, 2, 3, 4, 5};
// WRONG: Hardcoding the length
for (int i = 0; i <= 5; i++) { // This should be < 5
System.out.println(numbers[i]);
}
// CORRECT: Utilize the length property
for (int i = 0; i < numbers.length; i++) {
System.out.println(numbers[i]);
}
Not only does hardcoding the array length limit scalability, but it also risks logical errors when array sizes change. Using numbers.length
ensures adaptability—your code can gracefully handle arrays of varying sizes.
Best Practices
To avoid the common mistakes outlined, here are some best practices:
-
Always Check for Null: Before accessing an array’s elements, ensure it is initialized.
-
Use Length Property Consistently: Avoid hardcoding values; use the
length
property to maintain flexibility. -
Be Mindful of Type Compatibility: If an array is defined to hold a specific type, ensure you're only adding compatible types.
-
Implement Error Handling: Wrap your array access logic in try-catch blocks where necessary to catch potential exceptions early.
-
Use Enhanced For Loops: For loops can offer a more straightforward way to iterate through arrays:
☕snippet.javafor (int num : numbers) { System.out.println(num); }
-
Unit Testing: Implement unit tests for your methods that manipulate arrays to catch errors during the development phase.
Closing Remarks
When working with Java arrays, ensuring you’re accessing the last element properly can significantly reduce the likelihood of errors and improve your code’s resilience. By understanding the common mistakes and following best practices, you can enhance both your coding skills and the reliability of your applications.
For further reading on Java array manipulation, check out Java Documentation or take a look at advanced topics such as Collections in Java.
Happy coding!