Why Getters and Setters are Essential in Modern Coding
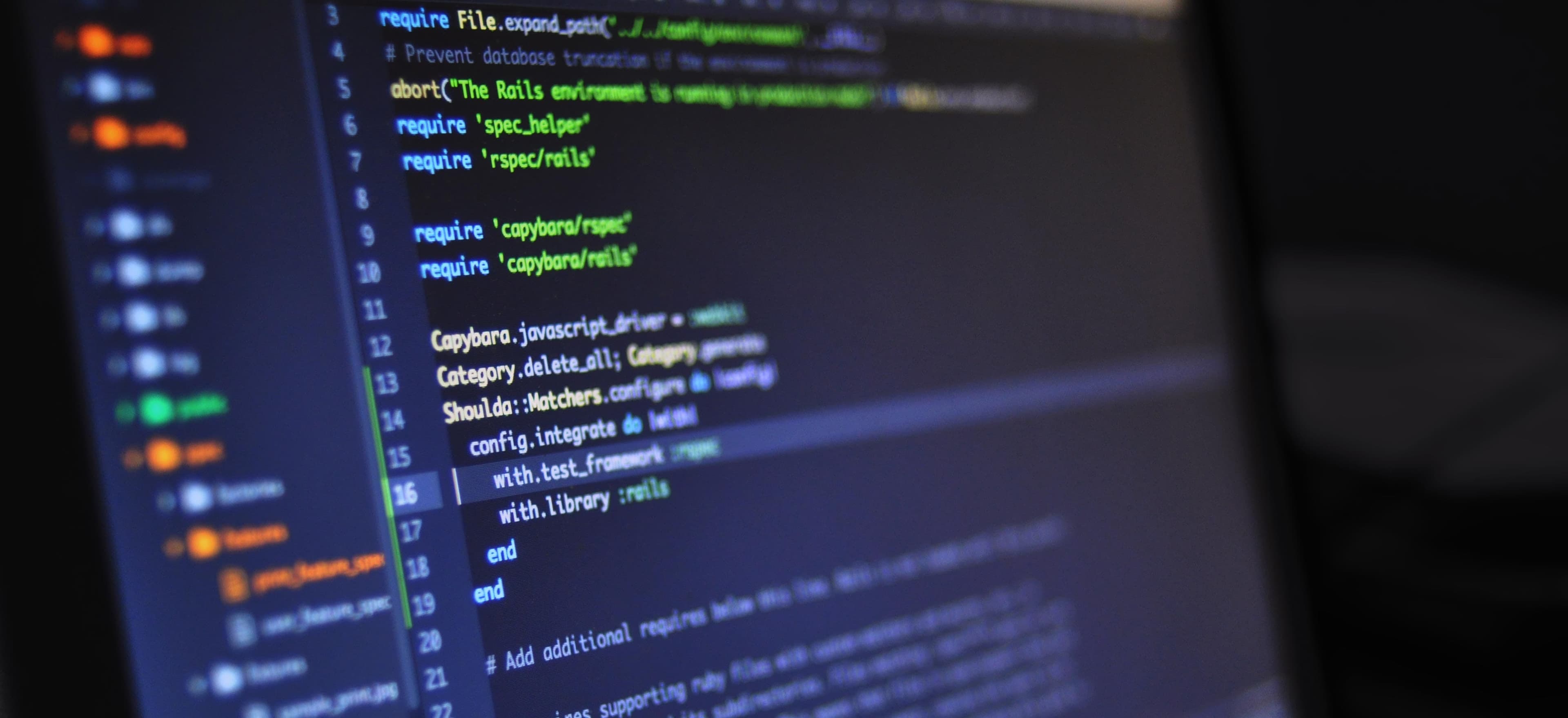
- Published on
Why Getters and Setters are Essential in Modern Coding
In the world of object-oriented programming, encapsulation is one of the key principles that enhance code maintainability and robustness. A crucial part of this principle involves the use of getters and setters. While on the surface they may seem like mere formalities, these methods play significant roles in promoting better software practices. In this blog post, we will delve deep into why getters and setters are still essential in modern coding, particularly in Java.
What are Getters and Setters?
Getters and setters are methods that provide access to private fields in a class. Getters retrieve the value of a variable, and setters update the variable's value. Here is a simple example:
public class Person {
private String name;
private int age;
// Getter for name
public String getName() {
return name;
}
// Setter for name
public void setName(String name) {
this.name = name;
}
// Getter for age
public int getAge() {
return age;
}
// Setter for age
public void setAge(int age) {
if (age > 0) { // simple validation
this.age = age;
} else {
throw new IllegalArgumentException("Age must be positive");
}
}
}
In this example, getName
and getAge
methods retrieve values of name
and age
, respectively. On the other hand, setName
and setAge
methods allow for controlled modification of these fields.
The Importance of Getters and Setters
1. Encapsulation
The principle of encapsulation is a cornerstone of object-oriented programming. By marking variables as private and exposing them via getters and setters, we restrict direct access to the internal state of an object. This prevents unintended interference and misuse. For instance, in the setAge
method shown above, we enforce a validation check to ensure age can only be positive. Direct access would have bypassed this check:
Person person = new Person();
person.setAge(-5); // Throws IllegalArgumentException
2. Data Validation and Control
Using setters allows developers to enforce business rules and constraints on data. Imagine a scenario where you want to ensure that a user cannot set a negative age. The setter can handle this validation internally, ensuring that the object remains in a valid state. Without a setter, you’d have to add this logic scattered throughout your codebase.
3. Flexibility and Maintainability
Getters and setters promote flexibility. If you decide to change how a variable is stored, you can easily do so without modifying external code that interacts with the object. For instance, if we later change the age
from an int
to a LocalDate
representing birthdate, updating our getter and setter alone suffices:
private LocalDate birthDate;
public LocalDate getBirthDate() {
return birthDate;
}
public void setBirthDate(LocalDate birthDate) {
this.birthDate = birthDate;
}
Your client code remains unchanged, thus improving maintainability.
4. Readability and Documentation
Getters and setters act as a form of documentation. They explicitly communicate the operations that can be performed on a class's fields. The method names getAge
and setAge
clearly indicate what they do. This self-documenting feature saves time for other developers reading your code or even for you when revisiting your code after a long time.
5. Future-Proofing Your Code
In modern programming environments, APIs can evolve quickly. Using getters and setters allows APIs to add more logic in the future without disrupting existing client code. For instance, say you initially just want to store a name. Later, you may want to implement logging or notifications whenever a name is changed. You can achieve this by modifying the setter:
public void setName(String name) {
logChange(name); // New logging logic
this.name = name;
}
6. Support for Lazy Loading and Caching
Another practical application arises in performance optimizations. For example, what if you had an expensive calculation that should be performed only when necessary? Using a getter, you can implement lazy loading:
private Integer cachedValue;
public Integer getExpensiveValue() {
if (cachedValue == null) {
cachedValue = computeExpensiveValue(); // Calculate once
}
return cachedValue;
}
In this scenario, the calculations are deferred until the first request for the value, improving performance.
7. Compatibility with Frameworks
Many modern frameworks leverage getter and setter methods to auto-generate behavior, like serialization or data binding. For example, JavaBeans standards necessitate public getters and setters to allow frameworks like JavaServer Faces or Hibernate to manipulate your objects seamlessly.
Final Considerations
Getters and setters may seem outdated or unnecessary at times, especially as we embrace modern features in programming, such as records in Java 14, which automatically generate access methods. However, understanding their foundational roles in encapsulation, data validation, maintainability, and the overall structure of code makes it clear that they are essential in modern coding practices.
Not only do they contribute to cleaner and more organized code, but they also ensure flexibility and longevity in applications under constant evolution.
For more information on Java best practices, you might find the following links useful:
Consider integrating getters and setters faithfully in your projects. They may require an extra line or two of code, but the benefits far outweigh the costs. Happy coding!
Checkout our other articles