Troubleshooting Common JPA Configuration Errors in Spring
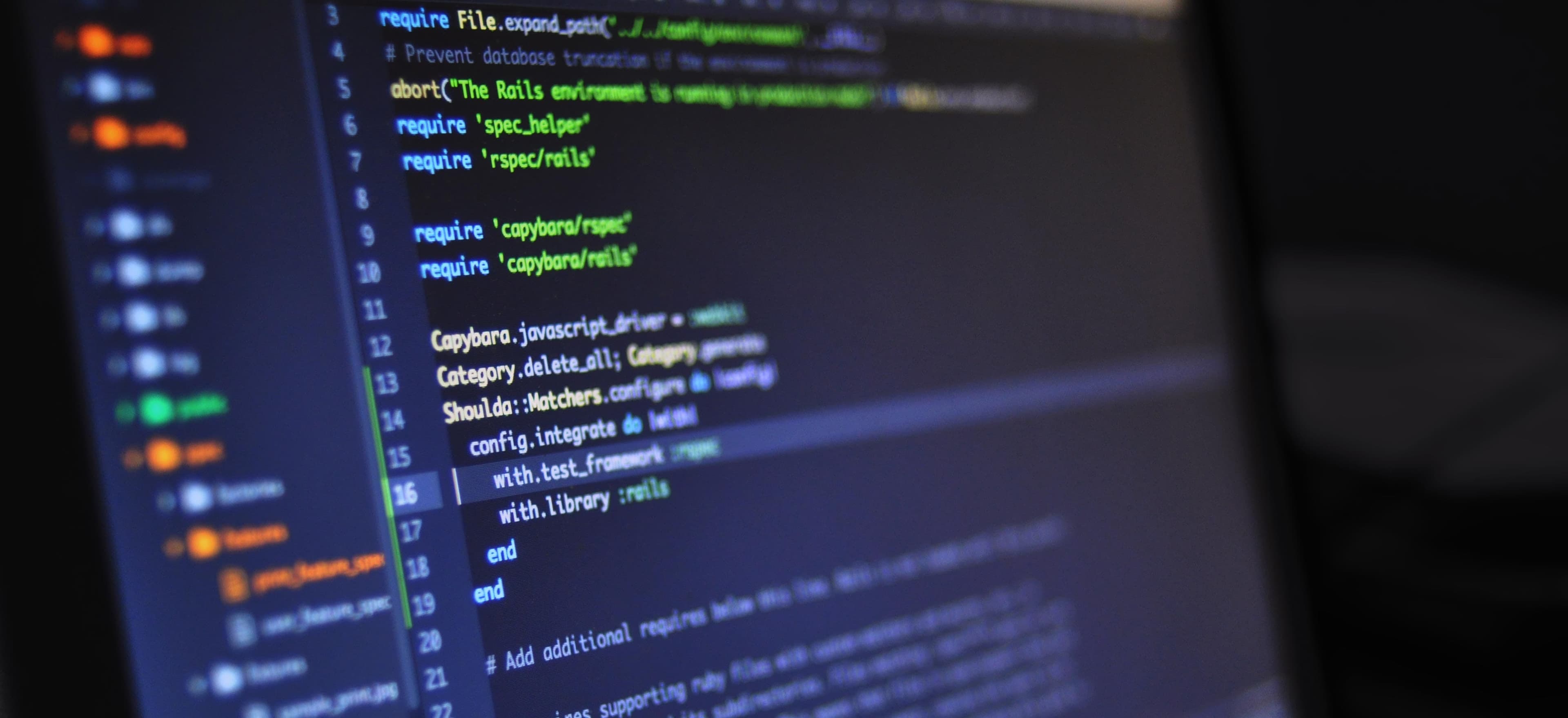
- Published on
Troubleshooting Common JPA Configuration Errors in Spring
The Java Persistence API (JPA) is a powerful specification for managing relational data in Java applications. When combined with Spring, JPA offers a robust framework for database interactions. However, configuration errors can derail even the best setups. In this blog post, we'll explore common JPA configuration errors in Spring applications, how to troubleshoot them, and best practices to avoid these pitfalls.
Understanding JPA and Its Configuration
JPA enables developers to work with databases through Java objects rather than SQL syntax. Spring Data JPA builds on top of JPA, providing easier and more sophisticated ways to interact with the database. The core components include:
- Entity Classes: These are your Java classes annotated with
@Entity
. - Repositories: Interfaces for CRUD operations and custom queries.
- Entity Manager: Handles the persistence of entities and interacts with the database.
To effectively utilize JPA with Spring, proper configuration is critical. Misconfigurations often lead to runtime errors, hindering application performance.
Common JPA Configuration Errors
Below are some of the most frequently encountered issues, along with methods to troubleshoot them.
1. DataSource Configuration Errors
Error: The application fails to start due to misconfigured DataSource.
Solution: Ensure that your application.properties
or application.yml
file contains valid database connection configurations.
# application.properties
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=root
spring.jpa.hibernate.ddl-auto=update
spring.jpa.show-sql=true
In this code snippet, make sure:
- The URL is correct, including the database name.
- The username and password are accurate.
- Use
spring.jpa.hibernate.ddl-auto=set
for development without losing data andupdate
in production.
Check for typos, incorrect ports, or insufficient permissions for database access.
2. Entity Classes Not Recognized
Error: Entities not being recognized, leading to PersistenceException
.
Solution: Ensure that your entity classes are defined correctly and that they are included in the package scanned by Spring.
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
// Getters and Setters
}
Make sure:
- Each entity class annotated with
@Entity
has a no-argument constructor. - The
@Id
annotation marks the unique identifier.
To scan all entities, use the @EntityScan
annotation in the main application class:
@SpringBootApplication
@EntityScan(basePackages = "com.example.myapp.model")
public class MyApp {
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
}
}
3. Missing Transaction Management
Error: Operations do not commit to the database.
Solution: Spring requires transaction management for JPA operations.
@Service
@Transactional
public class UserService {
@Autowired
private UserRepository userRepository;
public void createUser(User user) {
userRepository.save(user);
}
}
Use the @Transactional
annotation on your service layer to manage transactions. If your service method fails, changes will not be committed.
Ensure your repository interface extends JpaRepository
:
public interface UserRepository extends JpaRepository<User, Long> {
}
You can read more about Spring's transaction management here.
4. Hibernate Dialect Configuration
Error: Hibernate unable to determine the dialect of the database.
Solution: Explicitly set the dialect in your configuration.
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQLDialect
Substitute MySQLDialect
with the corresponding dialect of your database (e.g., PostgreSQLDialect
for PostgreSQL). This setting enables Hibernate to generate the appropriate SQL syntax for the database type.
5. Schema Generation Errors
Error: Application cannot start due to schema generation issues.
Solution: Check the spring.jpa.hibernate.ddl-auto
setting. Common values include:
none
: No action will be performed.update
: Updates the schema by adding new columns or tables.create
: Drops existing tables and creates new ones.create-drop
: Drops tables when the session factory is closed.
For development, update
is usually sufficient, but be careful with production databases, where data integrity is crucial.
6. Data Initialization Issues
Error: No initial data in the database upon application start.
Solution: Use data.sql
or schema.sql
files in src/main/resources
.
-- schema.sql
CREATE TABLE user (
id BIGINT AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(255) NOT NULL
);
-- data.sql
INSERT INTO user (username) VALUES ('admin'), ('user1'), ('user2');
Spring will automatically execute these scripts at startup, populating the database. Configuration in application.properties
should allow for these scripts:
spring.datasource.initialization-mode=always
7. LazyInitializationException
Error: Accessing a lazily-loaded entity outside of a transaction context raises an exception.
Solution: Manage your transactions carefully or fetch required data eagerly.
To configure a relationship as eager:
@OneToMany(fetch = FetchType.EAGER)
private List<Order> orders;
Alternatively, ensure your data is accessed within an active transaction. Use @Transactional
or fetch necessary data in the service layer.
Best Practices to Avoid JPA Configuration Issues
- Use Spring Profiles: Configure separate settings for development, testing, and production environments.
- Logging: Enable SQL logging to monitor the generated queries for troubleshooting.
spring.jpa.show-sql=true spring.jpa.properties.hibernate.format_sql=true
- Validation: Utilize JPA validation for entity fields using annotations like
@NotNull
,@Size
, etc. - Unit Testing: Write integration tests for repository and service layers to catch configuration issues early.
- Documentation: Keep your configuration files well documented for current and future developers.
In Conclusion, Here is What Matters
Diagnosing JPA configuration errors in Spring can be challenging, but understanding these common issues and their resolutions can significantly ease the development process. By following best practices and regularly reviewing your configurations, you can maintain a robust technical stack that supports a seamless database interaction layer.
For more information on JPA and Hibernate, consider reading the official Spring Data JPA documentation.
Feel free to leave your questions or experiences in the comments below!
Checkout our other articles