Demystifying Checked vs. Unchecked Exceptions in Java
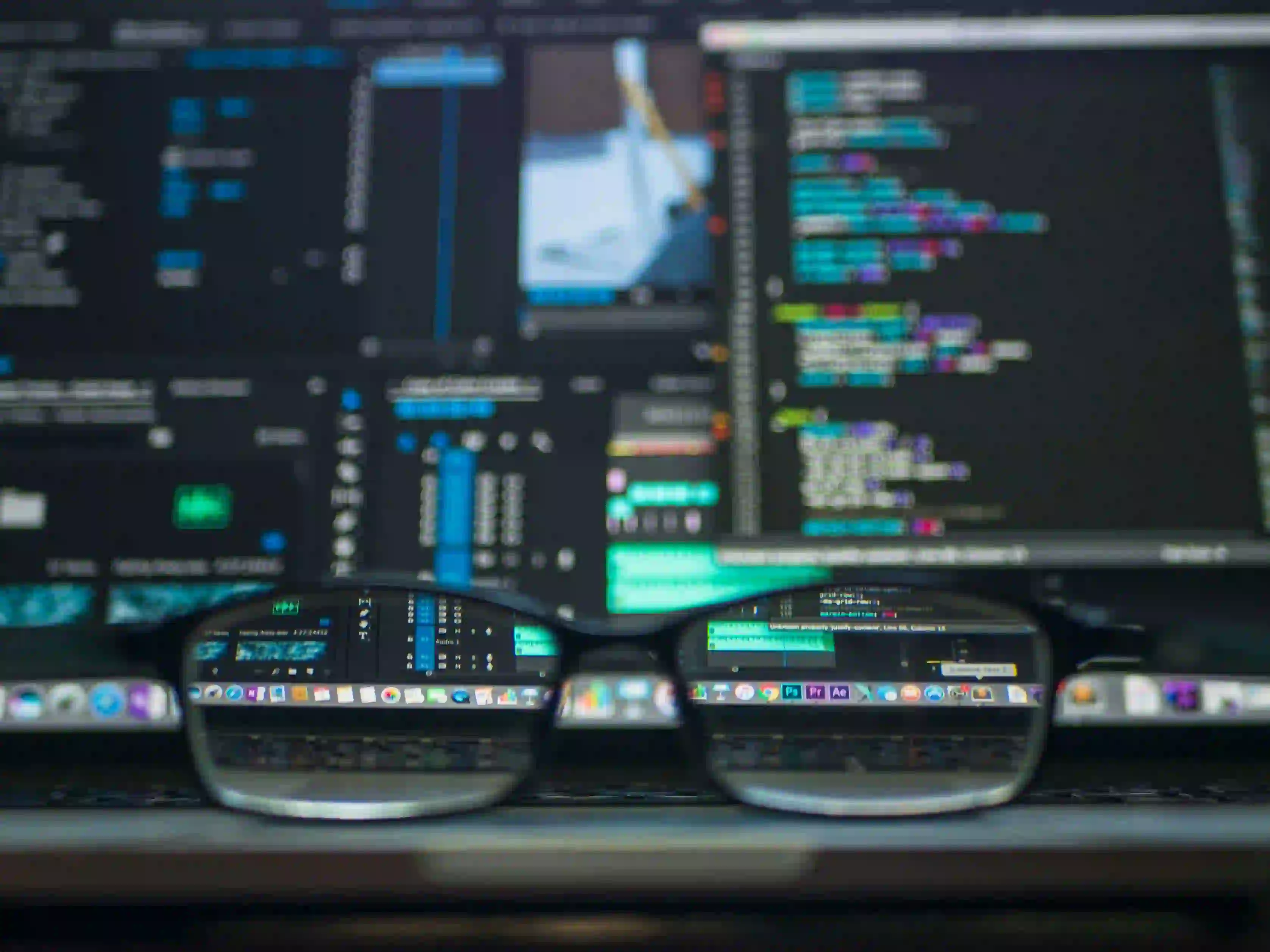
Demystifying Checked vs. Unchecked Exceptions in Java
In the realm of Java programming, handling errors and exceptions is critical for creating robust applications. Among the many concepts to grasp in Java exception handling, the distinction between checked and unchecked exceptions stands out. This blog post aims to clarify these differences and help you master exception handling in Java.
What are Exceptions?
In Java, an exception is an event that disrupts the normal flow of a program. These disruptions may arise from various issues, such as invalid user input, file not found errors, or failure in network connectivity.
Exception handling in Java is done through the use of try
, catch
, and finally
blocks. Here, we'll delve into two major categories of exceptions: checked and unchecked exceptions.
Checked Exceptions
Checked exceptions are those exceptions that are checked at compile-time. This means that if a method might throw a checked exception, it must either handle it with a try-catch block or declare it using the throws
keyword in its method signature.
Characteristics of Checked Exceptions
- Compile-time Validation: The Java compiler ensures that checked exceptions are handled, thus promoting error handling.
- Declaration: If a method throws a checked exception, it should declare this explicitly.
- Examples: Common checked exceptions include
IOException
,SQLException
, and custom exceptions that extendException
.
Why Use Checked Exceptions?
Checked exceptions are particularly beneficial in scenarios where the programmer can anticipate a potential problem. They force the developer to recognize possible failure points in their code.
For example, consider a file reading operation:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FileReadExample {
public static void readFile(String filePath) {
BufferedReader reader = null;
try {
reader = new BufferedReader(new FileReader(filePath));
String line = null;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
System.err.println("An IOException occurred: " + e.getMessage());
} finally {
try {
if (reader != null) {
reader.close();
}
} catch (IOException e) {
System.err.println("Error closing the reader: " + e.getMessage());
}
}
}
public static void main(String[] args) {
readFile("nonexistent_file.txt");
}
}
In this example, if nonexistent_file.txt
does not exist, the program will handle the IOException
smoothly. The try-catch
block ensures that the error does not lead to program termination.
Unchecked Exceptions
Unchecked exceptions, on the other hand, are not checked at compile-time. They are subclasses of RuntimeException
and Error
, and they generally represent programming errors, such as logic flaws or incorrect API usage.
Characteristics of Unchecked Exceptions
- Run-time Validation: These exceptions are validated at runtime, meaning the compiler does not require handling.
- Implicit Handling: Developers are not forced to explicitly either handle or declare them, though it is often good practice to do so.
- Examples: Java's built-in unchecked exceptions include
NullPointerException
,ArrayIndexOutOfBoundsException
, andArithmeticException
.
Why Use Unchecked Exceptions?
Unchecked exceptions are often used to indicate programming errors that could have been avoided with proper coding. They convey issues that the programmer should fix rather than exceptional circumstances to handle gracefully.
Here’s an example where an unchecked exception occurs:
public class DivisionExample {
public static int divide(int numerator, int denominator) {
return numerator / denominator; // May throw ArithmeticException
}
public static void main(String[] args) {
try {
int result = divide(10, 0); // This will throw ArithmeticException
System.out.println("Result: " + result);
} catch (ArithmeticException e) {
System.err.println("Cannot divide by zero: " + e.getMessage());
}
}
}
Here, attempting to divide by zero will cause an ArithmeticException
. This demonstrates an error that could have easily been avoided by ensuring that the denominator was not zero.
Key Differences
To summarize, here are the paramount differences between checked and unchecked exceptions:
| Feature | Checked Exceptions | Unchecked Exceptions |
|--------------------------|---------------------------------------|-------------------------------------|
| Compilation Check | Checked at compile time | Not checked; checked at runtime |
| Inheritance | Inherit from Exception
| Inherit from RuntimeException
|
| Handling Requirement | Must be handled or declared | Not mandatory to handle or declare |
| Examples | IOException
, SQLException
| NullPointerException
, IllegalArgumentException
|
Best Practices for Exception Handling
- Use Checked Exceptions for Recoverable Conditions: If your application can recover from certain conditions (like file not found), prefer checked exceptions.
- Use Unchecked Exceptions for Programming Errors: When the error stems from a mistake in logic, utilize unchecked exceptions to signal that the programmer needs to rectify the code.
- Don't Overuse Exceptions: Exception handling should not be used for flow control. Reserve it for unexpected conditions.
- Log Exception Details: When catching exceptions, always log complete error information to aid in debugging.
In Conclusion, Here is What Matters
Understanding the distinction between checked and unchecked exceptions is pivotal for effective exception handling in Java. By utilizing checked exceptions for recoverable situations and unchecked exceptions for programming errors, you can create cleaner, more robust applications.
For a deeper exploration of Java exceptions, refer to Java Exception Handling provided by Oracle, and dive into the nuances of exception hierarchy and best practices.
By mastering this aspect of Java, you will not only improve the quality of your code but also enhance its maintainability and reliability. Happy coding!