Overcoming Common Pitfalls in Automation Testing
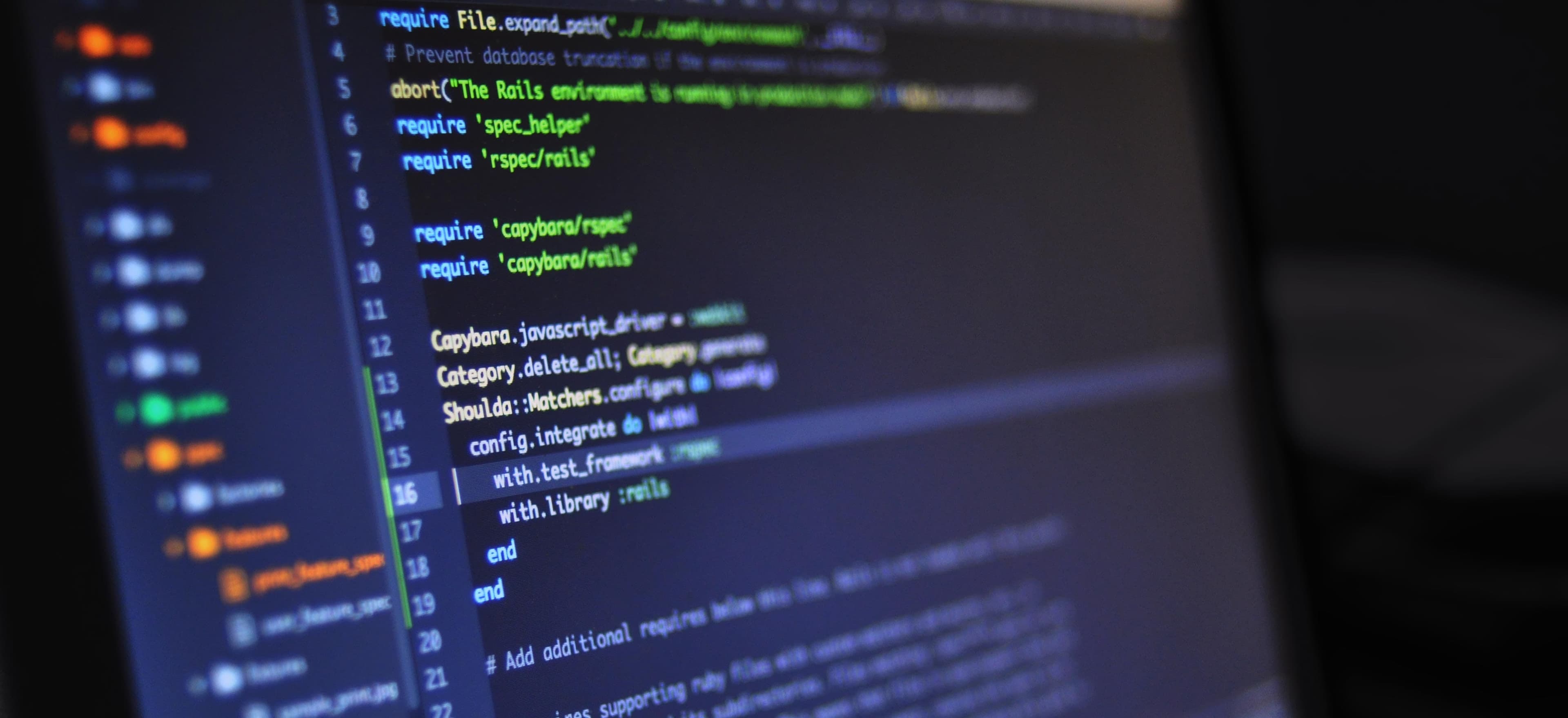
- Published on
Overcoming Common Pitfalls in Automation Testing
Automation testing is a vital process in the realm of software development. As applications grow in complexity, ensuring their quality through automated tests becomes not just beneficial but necessary. However, automation testing comes with its own set of challenges. In this blog post, we’ll discuss the common pitfalls when implementing automated testing and how to overcome them using best practices.
Understanding Automation Testing
Before we dive into the pitfalls, let’s clarify what automation testing entails. Automation testing uses specialized tools and scripts to execute tests on software applications automatically. It helps in saving time, increasing accuracy, and allowing for the repetitive execution of tests.
However, it’s essential to recognize that automation is not a silver bullet—it requires thoughtful implementation and maintenance.
Common Pitfalls in Automation Testing
- Lack of Clear Strategy
A clear automation strategy is paramount for success. Many teams jump into automation without understanding the scope or the desired outcome.
Solution:
-
Define Objectives: Before beginning, outline what you aim to achieve. Is it speed? Accuracy? Coverage?
-
Prioritize Tests: Focus on automating the most critical tests first.
Here's a simple approach to help prioritize tests:
List<Test> tests = getAllTests(); List<Test> criticalTests = tests.stream() .filter(test -> test.isCritical()) .collect(Collectors.toList());
This code snippet filters tests to identify critical ones, ensuring the team concentrates on what matters most.
- Choosing the Wrong Tools
The vast array of tools available can be overwhelming. Picking the wrong tool can lead to ineffective testing processes.
Solution:
-
Evaluate Tools Based on Requirements: Consider compatibility with the tech stack, community support, and learning curves.
-
Pilot Projects: Run a small pilot project using the tool before full-scale implementation.
For further exploration on selecting testing tools, visit this resource.
- Neglecting Test Maintenance
As applications evolve, so do the tests. Failing to maintain automated tests leads to a growing accumulation of broken or outdated test cases.
Solution:
-
Regular Reviews: Schedule periodic test reviews and update them as necessary.
-
Refactor Regularly: Just like code, automated tests should be refactored for clarity and efficiency.
Here’s an example of refactoring a test method to improve readability:
public void testLoginSuccess() { login(username, password); assertTrue(isUserLoggedIn()); }
This method improves readability by being clear on what the test aims to validate.
- Ignoring Test Reports
Automated tests generate a wealth of data. Ignoring these reports can mean missing critical insights into quality and performance.
Solution:
-
Implement Reporting Tools: Use reporting frameworks like JUnit or TestNG that provide clear visual feedback.
-
Analyze Trends: Regularly review test results to identify patterns that could indicate larger problems.
Here's how you can implement a simple reporting system in JUnit:
@After public void tearDown() { System.out.println("Test Outcome: " + (result.wasSuccessful() ? "Success" : "Failure")); }
This code snippet provides immediate feedback on the outcome of each test.
- Over-Automation
It’s tempting to automate everything—after all, automation is designed to improve efficiency. However, not all tests should be automated.
Solution:
- Identify Suitable Test Cases: Typically, tests that are repetitive, time-consuming, or prone to human error are the best candidates for automation.
- Combine Approaches: Manual testing still holds value for exploratory tests and UX assessments.
- Insufficient Training
Many teams lack the knowledge needed to create effective automated tests, leading to poorly written scripts that offer little value.
Solution:
-
Training and Workshops: Invest in training programs for your team.
-
Mentorship: Pair experienced testers with those new to automation.
If you're looking for online courses, platforms like Coursera or Udacity offer excellent resources.
- Not Using Version Control
Automation scripts can become outdated or collide with changes, leading to confusing errors and bugs.
Solution:
-
Version Control Systems: Use tools like Git to manage and track changes in your test scripts.
-
Branching Strategies: Implement strategies to keep your automated tests aligned with the main development branch.
Here’s a sample Git command for committing your automated test code:
git add . git commit -m "Updated automated test scripts"
This command is essential for keeping your test changes tracked.
- Neglecting Environment Management
Inconsistent testing environments can lead to tests passing in one environment while failing in another.
Solution:
- Use Containers or VM: Tools like Docker can ensure that your test cases run in the same environment every time.
- Configuration Management: Use tools like Ansible or Puppet to standardize environments.
In Conclusion, Here is What Matters
Automation testing is an essential process in modern software development, but it requires careful planning and upkeep to harness its full potential. By avoiding common pitfalls, teams can streamline their automated testing processes, enhance their software quality, and ultimately deliver superior products to users.
Action Steps
- Assess your current automation strategy.
- Identify areas for improvement using the solutions outlined above.
- Implement a rigorous review and maintenance schedule.
For those interested in diving deeper, Automate Testing offers an extensive resource hub filled with guidelines and best practices.
By following these guidelines, you can enhance your software testing processes, ensure consistent and reliable results, and ultimately drive your software projects to success. Remember, while automation can significantly aid testing efforts, it thrives with the right strategy, tools, and ongoing commitment. Happy testing!