Enhancing Java Skills: Must-Read Books for Developers
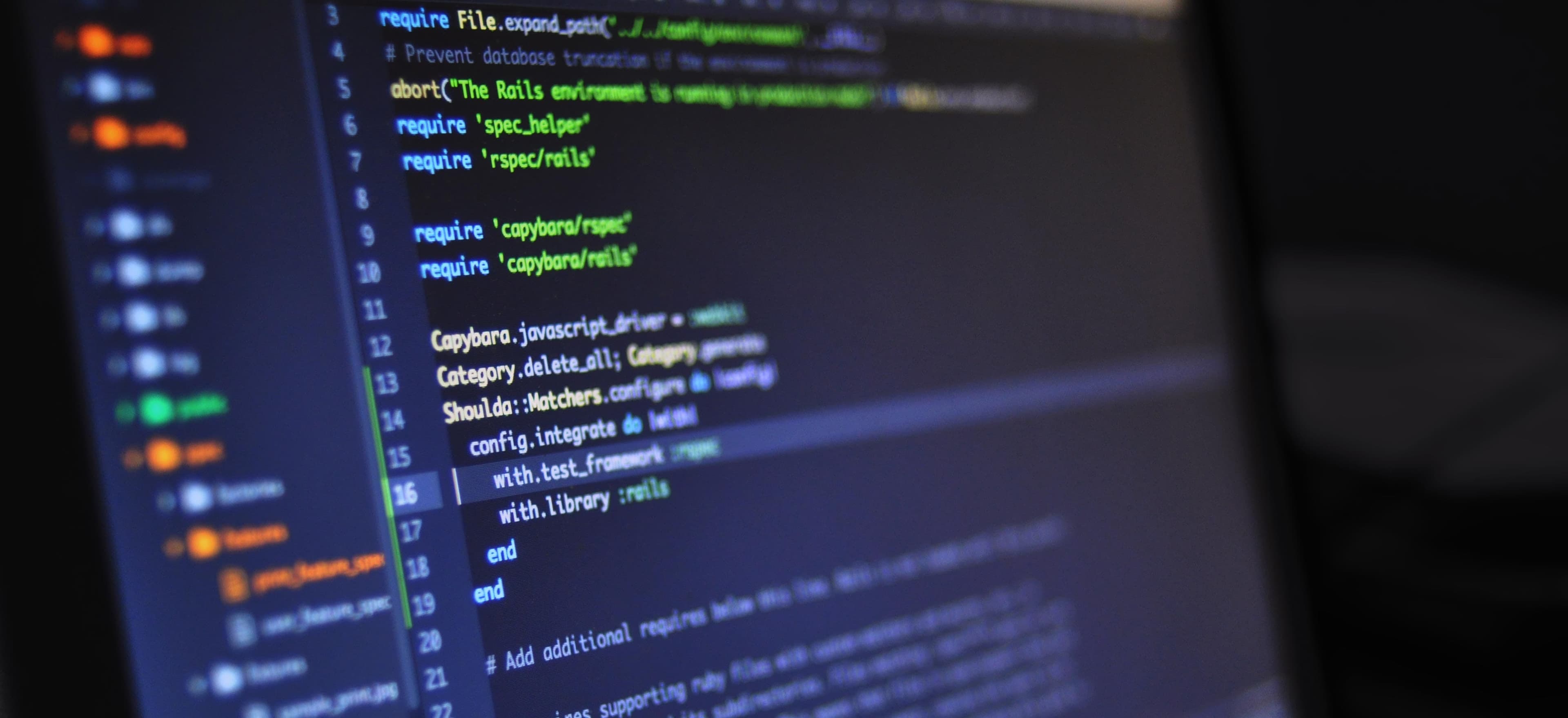
- Published on
Enhancing Java Skills: Must-Read Books for Developers
In the continually evolving world of programming, staying updated and enhancing your skills is imperative. Java, one of the most popular programming languages since its inception in 1995, remains a vital tool for developers. Whether you are a beginner or an experienced programmer looking to refine your expertise, acquiring knowledge from the right sources can dramatically improve your programming prowess. This blog post will explore a curated list of must-read books for Java developers.
Why Reading Books Matters
While the internet offers a plethora of resources, books provide a structured and depth-oriented approach to learning. Well-compiled books can offer insights, best practices, and a deeper understanding of concepts that popular articles or video tutorials may not fully cover.
Top Must-Read Books for Java Developers
1. Effective Java by Joshua Bloch
Effective Java is often regarded as the "bible" of Java programming. Written by Joshua Bloch, one of the original authors of Java, this book offers best practices for programming in Java.
Key Takeaways:
-
The Importance of Static Factory Methods:
Instead of using constructors, static factory methods provide a way to create instances with more flexibility. Here’s why:
public class Pizza { private final Size size; private Pizza(Size size) { this.size = size; } public static Pizza large() { return new Pizza(Size.LARGE); } }
This code improves readability and allows for more clarity in the creation of pizza instances.
-
Understanding Immutability:
Immutability is critical in Java, especially in multi-threaded applications. By making objects immutable, you avoid numerous problems related to shared mutable state.
2. Java Concurrency in Practice by Brian Goetz
Concurrency can be one of the most challenging aspects of programming. Java Concurrency in Practice provides a comprehensive overview of concurrent programming in Java.
Key Takeaways:
-
Thread Safety:
The concept of ensuring that multiple threads do not interfere with each other can be illustrated with this code snippet:
public class Counter { private int count; public synchronized void increment() { count++; } public synchronized int getCount() { return count; } }
Synchronizing the increment method ensures that only one thread can modify count at a time.
-
Deadlock Resolution:
Understanding deadlocks – where two or more threads are blocked forever, waiting for each other – is crucial. The book provides strategies for designing multi-threaded applications that minimize the chances of deadlocks.
3. Java: The Complete Reference by Herbert Schildt
Java: The Complete Reference serves as a comprehensive guide, making it suitable for beginners and experienced programmers alike. This book dives deep into Java fundamentals and advanced topics.
Key Takeaways:
-
In-Depth Language Features:
Schildt covers Java’s core features such as Java Syntax, Data Types, and OOP principles extensively, which are crucial for anyone looking to master the language.
-
Java 11 Updates:
The book touches on the latest Java updates, allowing developers to stay current with new features such as
var
, which simplifies variable type declarations.
4. Clean Code: A Handbook of Agile Software Craftsmanship by Robert C. Martin
Clean Code is not limited to any programming language but is a must-read for all developers. This book tackles the importance of writing clean and maintainable code.
Key Takeaways:
-
Naming Things:
Martin emphasizes that clear and descriptive names are critical for code readability. For example:
double calculateCircleArea(double radius) { return Math.PI * radius * radius; }
The method name itself describes what the function does, promoting clarity.
-
Avoiding Magic Numbers:
Magic numbers can lead to confusion. Instead of using values directly in your code, define meaningful constants.
public static final double PI = 3.141592653589793;
5. Head First Java by Kathy Sierra and Bert Bates
For those who prefer an engaging and visual learning style, Head First Java offers a unique approach. It employs a conversational style with lots of illustrations, making complex topics easier to grasp.
Key Takeaways:
-
Visual Learning:
The book simplifies intricate concepts, such as OOP principles, through visual aids and analogies, making them more relatable.
-
Hands-On Exercises:
Learning by doing is one of the most effective methods, and "Head First Java" ensures that you get your hands dirty with coding exercises.
Final Thoughts
Upgrading your Java skills isn't just about hands-on coding. It's about diving deep into the theory, best practices, and principles that govern good programming. The books highlighted in this post can help you refine your existing knowledge and introduce you to new concepts that may reshape your understanding of Java.
Investing time in reading these essential books can pay dividends in your career as a Java developer. Whether you choose to read them cover-to-cover or refer back to them as a resource guide, each book promises valuable insights.
As technology continues to evolve, your growth as a developer relies on commitment and continuous learning. Make it a goal to pick up one of these books and discover the wealth of knowledge they contain in your journey to enhance your Java skills.
Feel free to leave your thoughts or share additional resources in the comments section! Happy coding!