Reducing High CPU Usage from HotSpot GC Threads on Linux
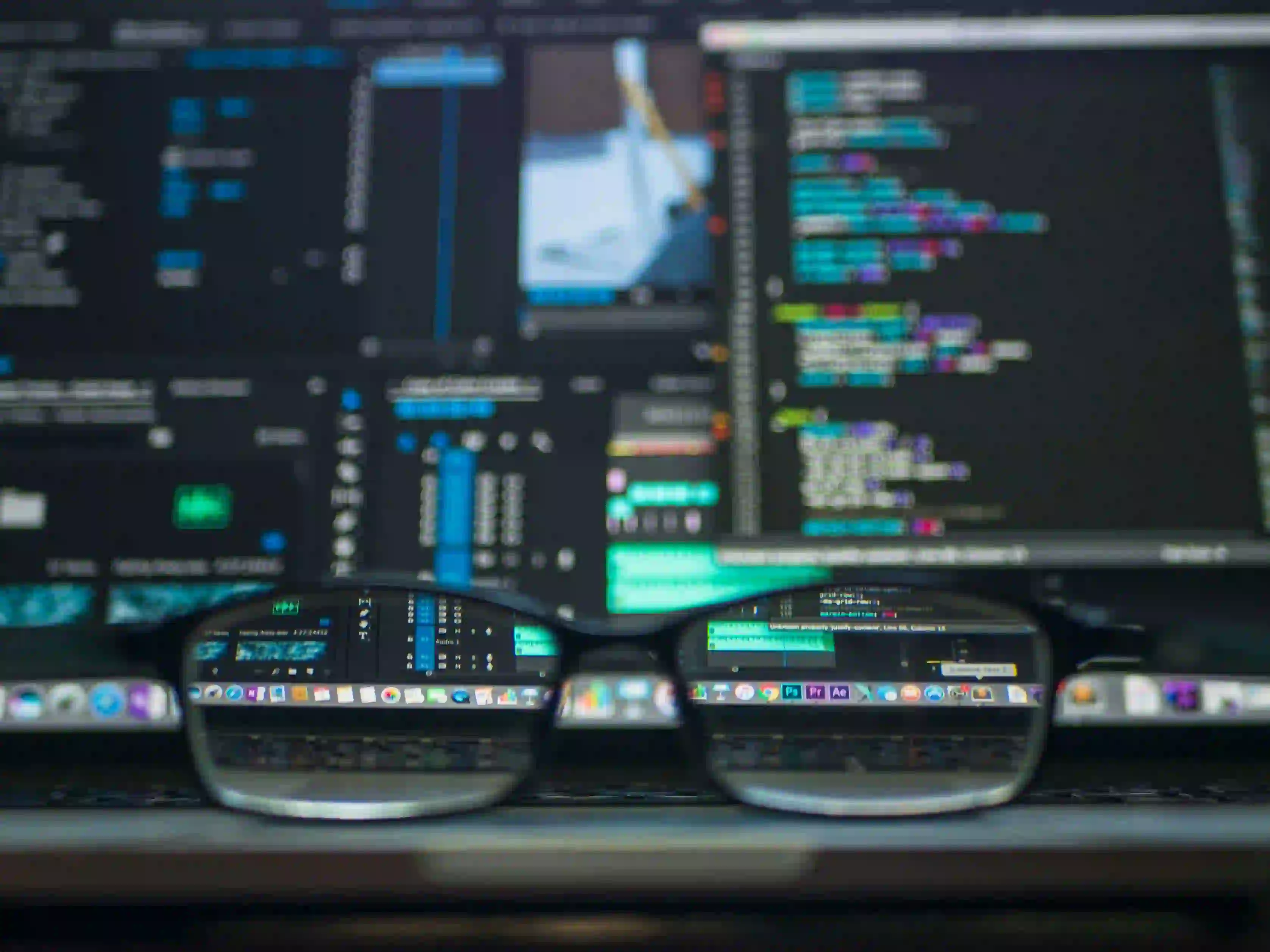
Reducing High CPU Usage from HotSpot GC Threads on Linux
Java applications are revered for their portability and robustness. However, one common issue developers face is high CPU usage caused by the HotSpot Garbage Collector (GC) threads, particularly on Linux systems. This blog post delves into understanding this issue and offers practical solutions. By optimizing garbage collection, you can minimize CPU usage and enhance application performance.
Understanding Garbage Collection in Java
Garbage Collection is an automatic memory management feature in Java. It helps reclaim memory used by objects that are no longer referenced in the application, preventing memory leaks. However, GC processes can become CPU-intensive, particularly if not optimally configured.
The HotSpot JVM is the default Java Virtual Machine that employs different garbage collection algorithms. While these algorithms are efficient, their CPU consumption can spike under certain conditions.
Why High CPU Usage?
High CPU usage from GC threads can stem from several factors:
- Object Creation Rate: An increased rate of object creation can lead to frequent garbage collections.
- Memory Size: A large heap size means more time spent for GC to process the objects.
- GC Algorithm: Using a less-efficient GC algorithm for your workload can also lead to high CPU consumption.
Choosing the Right Garbage Collector
Java offers several garbage collection options, including:
-
Serial Garbage Collector: Suitable for small applications with small heap sizes and will pause all application threads during collections.
-
Parallel Garbage Collector: This is the default GC for most applications. It uses multiple threads for managing heap memory, making it ideal for multi-threaded applications.
-
Concurrent Mark-Sweep (CMS): This minimizes pauses by working concurrently with the application threads.
-
G1 Garbage Collector: This is designed for applications with large heaps and is aimed at providing predictable pause times.
Selecting the Suitable GC Algorithm
Depending on the workload, selecting a suitable GC can significantly reduce CPU usage. For example, to switch to G1 GC you would add the following option to your java
command:
java -XX:+UseG1GC -jar your-application.jar
Configuration Options
The JVM offers numerous configuration parameters to optimize garbage collection. Here are a few common options you can tune:
-
Heap Size: Setting the maximum and initial heap size can drastically alter performance.
🔧snippet.shjava -Xms512m -Xmx4g -jar your-application.jar
Here, we set the initial heap size to 512MB and the maximum to 4GB. The goal is to reduce the frequency of GC events.
-
GC Threads: For multi-threaded applications, consider adjusting the number of GC threads:
🔧snippet.shjava -XX:ParallelGCThreads=4 -jar your-application.jar
Increasing the number of GC threads can decrease overall GC time.
-
Pause Time Goals: Setting a pause time goal can help the G1 GC to adjust its behavior to fit needs:
🔧snippet.shjava -XX:MaxGCPauseMillis=200 -jar your-application.jar
This option aims for a maximum pause time of 200 milliseconds.
Monitoring GC Activity
Using Java VisualVM
Before making any changes, it’s essential to monitor the current behavior of your application’s GC. You can use tools like Java VisualVM or JConsole to visualize memory usage and GC activity. These tools provide useful insight into:
- Frequency of GC events
- Duration of GC pauses
- Heap Memory Usage
Refer to Java VisualVM documentation for further details on installation and usage.
Logging GC Activity
You can enable GC logging to delve deeper into performance issues. A typical command would look like this:
java -Xlog:gc*:file=gc.log:time,uptime,level,tags -jar your-application.jar
This command creates a gc.log
file with pertinent GC metrics, which can be analyzed later to identify potential bottlenecks.
Code Optimization Strategies
In addition to GC tuning, optimizing your Java code can also play a critical role in reducing CPU load.
Minimize Object Creation
Excessive object creation leads to more frequent garbage collections. Instead of creating new objects inside loops, consider reusing objects:
// Not optimal
for (int i = 0; i < 100000; i++) {
String str = new String("Hello World"); // creating new String object
}
// Optimal
String str = "Hello World"; // Single String object
for (int i = 0; i < 100000; i++) {
// Use the existing object instead of creating new ones
}
Use StringBuilder for String Concatenation
When you concatenate strings in a loop, it results in excessive object creation:
// Not optimal
String result = "";
for (String s : strings) {
result += s; // Creates new objects for each concatenation
}
// Optimal
StringBuilder sb = new StringBuilder();
for (String s : strings) {
sb.append(s); // Modifying the same object
}
String result = sb.toString();
Utilize Collections Wisely
Choosing the right collections can also aid in memory management.
- Instead of using a
LinkedList
, which has overhead due to pointers, use anArrayList
both for speed and memory efficiency.
List<String> list = new ArrayList<>(); // More memory-efficient
// Instead of
List<String> list = new LinkedList<>();
Final Thoughts
Managing high CPU usage from HotSpot GC threads is essential for maintaining the performance of Java applications, especially on Linux systems. Instead of tolerating high resource consumption, you can and should employ effective techniques and configurations.
- Choose the right garbage collector for your application’s needs.
- Monitor GC behavior and performance.
- Optimize your code to reduce object creation and improve efficiency.
By systematically implementing these strategies, you will significantly reduce CPU load, thereby enhancing the performance and responsiveness of your Java applications. For more detailed insights, visit the Java Garbage Collection Tuning Guide.
With continual learning and optimization, we can prevent our applications from becoming a victim of their own automated memory management systems. So, roll up your sleeves, apply these techniques, and watch your CPU usage shrink!