How to Easily Add a Banner to Your Grails Application
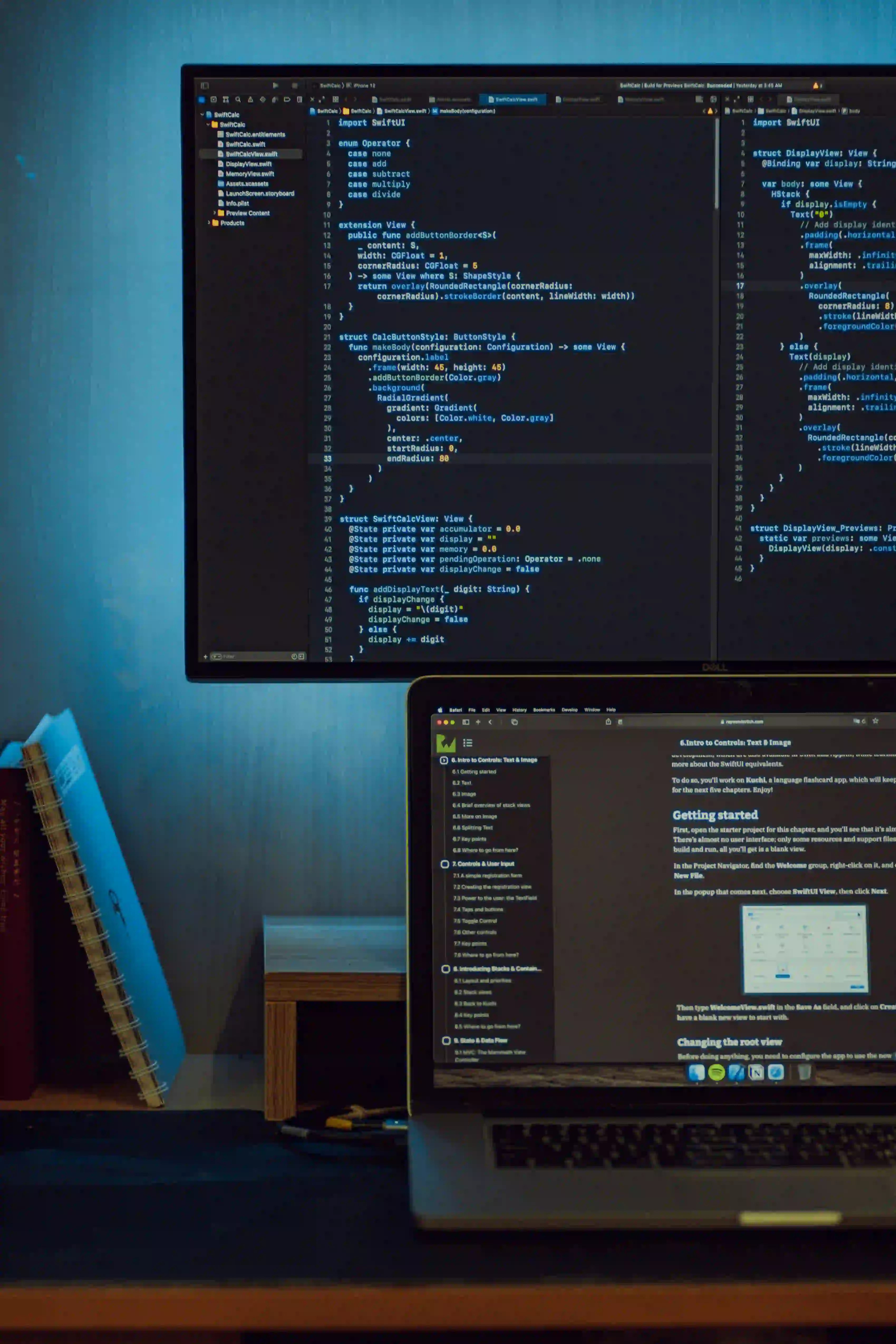
How to Easily Add a Banner to Your Grails Application
When developing a Grails application, you may want to incorporate engaging elements to enhance user experience. One simple and effective method is by adding a banner to your application. Not only does this create visual interest, but it also serves as an effective tool for communication—be it promotional content, announcements, or reminders. In this post, we will explore how you can seamlessly integrate a banner into your Grails application.
Understanding Grails
Before diving into code, it's essential to have a basic understanding of what Grails is. Grails is an open-source web application framework that leverages the Spring framework and Groovy programming language. Designed for developers who appreciate rapid application development, Grails provides a robust environment built on convention over configuration.
Planning Your Banner
Before you start coding, let’s outline some considerations for your banner:
- Purpose: Is the banner for announcements, promotions, or something else? Define its main message.
- Design: Will the banner be static, or do you plan to use dynamic elements like slides or animations?
- Position: Where will the banner be located on your webpage? Consider visibility and UX.
- Responsiveness: Ensure the banner looks good on all devices.
Adding a Banner to Grails Application
Step 1: Create the Banner GSP
Graeme’s Server Pages (GSP) files are similar to HTML and are fundamental for creating views in Grails. Create a GSP file that will hold your banner code. Here’s how to do it:
<!-- grails-app/views/banner.gsp -->
<%@ page contentType="text/html;charset=UTF-8" %>
<html>
<head>
<style>
.banner {
background-color: #4CAF50; /* Green */
color: white;
padding: 15px;
text-align: center;
position: relative;
width: 100%;
z-index: 1000; /* Ensures it stays on top */
}
.banner a {
color: white;
text-decoration: underline;
}
</style>
</head>
<body>
<div class="banner">
Come and check our new features! Visit our <a href="/features">Features Page</a> for more information.
</div>
</body>
</html>
Explanation:
- CSS Styling: This defines styles for the banner, including colors, padding, and positioning. The
z-index
is used to ensure the banner appears over other content. - Content: Dynamic links or texts can easily be modified for different announcements.
Step 2: Include the Banner in Your Main Layout
In Grails, the main layout file is typically located at grails-app/views/layouts/main.gsp
. You can include your banner by using the <g:render>
tag:
<!DOCTYPE html>
<html>
<head>
<title>${title}</title>
<g:layoutHead />
</head>
<body>
<g:render template="/banner" />
<div class="content">
<g:layoutBody />
</div>
<g:layoutResources />
</body>
</html>
Explanation:
- The
<g:render>
tag allows you to include the banner every time the main layout is rendered, ensuring it appears on all pages. - The placement above the content section makes it visible and prominent.
Step 3: Customize the Banner's Behavior
A banner can be enhanced further by incorporating some JavaScript functionality. For example, you may want to allow users to close the banner:
<script>
document.addEventListener('DOMContentLoaded', function() {
const banner = document.querySelector('.banner');
const closeBtn = document.createElement('span');
closeBtn.textContent = '✖'; // Close Icon
closeBtn.style.cursor = 'pointer';
closeBtn.style.float = 'right';
closeBtn.onclick = function() {
banner.style.display = 'none';
};
banner.appendChild(closeBtn);
});
</script>
Explanation:
- This JavaScript code allows users to close the banner seamlessly.
- By creating a close button dynamically, you ensure no additional modifications in the GSP are needed when implementing this feature.
Additional Resources for Enhancements
Adding a static banner is just the beginning. You may want to delve into more advanced techniques like creating slideshows or responsive designs. Here are two resources you might find useful:
- Grails Documentation: Get in-depth knowledge about Grails capabilities and features.
- Bootstrap: Use Bootstrap’s carousel component to create a dynamic banner with images and links.
Testing Your Banner
Always test to ensure that your banner is displayed correctly across different browsers and devices. Take advantage of browser developer tools to check for responsiveness and layout issues.
# If you are using Grails in development mode, run:
grails run-app
Key Takeaways
Adding a banner to your Grails application is straightforward and significantly enhances communication and engagement with your users. By following the steps outlined in this post, you can create a visually appealing and functional banner in no time.
From basic HTML to incorporating JavaScript, the possibilities are virtually endless. Embrace the power of Grails, and continuously strive to enhance user experience with simple yet effective designs.
Happy coding!