Handling Java Date-Time Fields with Spring WebClient
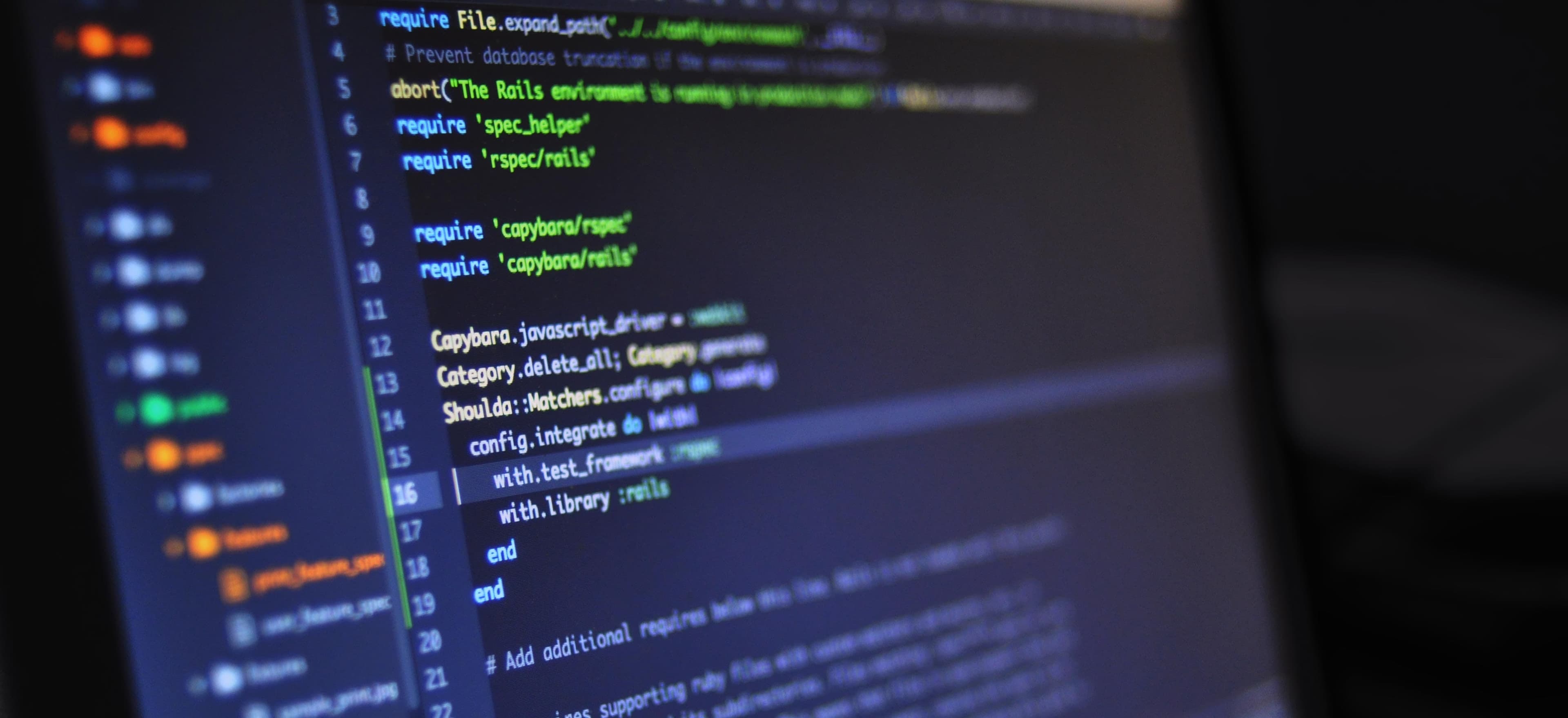
- Published on
Handling Java Date-Time Fields with Spring WebClient
In the landscape of modern web applications, handling date and time effectively is crucial. Java offers powerful tools for managing date and time through its java.time
package. When combined with Spring WebClient, it results in an efficient and streamlined way to handle date-time fields over HTTP. This blog post will explore how to manage Java date-time fields while interacting with APIs using Spring WebClient.
Why Use Spring WebClient?
Spring WebClient is part of the Spring WebFlux framework and serves as a non-blocking, reactive client designed for making HTTP requests. It simplifies interaction with RESTful services while supporting various synchronization and deserialization features. Unlike RestTemplate
, WebClient is built for asynchronous programming, making it suitable for applications that require high throughput and low latency.
Understanding Java Date-Time API
Java introduced a new Date-Time API in Java 8 with the java.time
package, addressing issues seen in older date-time libraries like java.util.Date
. It provides an intuitive way to manage local dates, times, durations, and time zones. Here’s a quick overview of some commonly used classes:
- LocalDate: Represents a date without time-zone.
- LocalTime: Represents a time without date.
- LocalDateTime: Combines LocalDate and LocalTime.
- ZonedDateTime: Represents a date-time with time-zone.
Prerequisites
Before diving in, ensure you have the following in your development environment:
- Java 11 or later
- Spring Boot 2.5 or later
- Maven or Gradle build tool
You can set up a new Spring Boot project using Spring Initializr.
Maven Dependency
To use Spring WebClient, include the following dependency in your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
Gradle Dependency
If you are using Gradle, add the following to your build.gradle
:
implementation 'org.springframework.boot:spring-boot-starter-webflux'
Example API to Work With
For this example, we will simulate an API that provides user information, including a registration date (as LocalDateTime
).
API Endpoint: GET /api/users/{id}
Sample Response:
{
"id": 1,
"name": "John Doe",
"registrationDate": "2023-09-15T10:15:30"
}
The registrationDate
field is in ISO-8601 format, which is the default format supported by Java's LocalDateTime
.
Making an HTTP GET Request with WebClient
Let’s create a simple Spring Boot application that retrieves user information from the API using WebClient.
Configuration Class
First, we need to set up a WebClient bean in our configuration class:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.reactive.function.client.WebClient;
@Configuration
public class WebClientConfig {
@Bean
public WebClient.Builder webClientBuilder() {
return WebClient.builder();
}
}
Service Class
Next, we implement a service class that will handle the API interactions.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.web.reactive.function.client.WebClient;
import reactor.core.publisher.Mono;
import java.time.LocalDateTime;
@Service
public class UserService {
private final WebClient webClient;
@Autowired
public UserService(WebClient.Builder webClientBuilder) {
this.webClient = webClientBuilder.baseUrl("http://localhost:8080").build(); // Adjust the base URL accordingly
}
public Mono<UserResponse> getUserById(int userId) {
return webClient.get()
.uri("/api/users/{id}", userId)
.retrieve()
.bodyToMono(UserResponse.class);
}
}
Data Model
Now, let’s define our data model that corresponds to the API response.
import java.time.LocalDateTime;
public class UserResponse {
private int id;
private String name;
private LocalDateTime registrationDate;
// Getters and Setters
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public LocalDateTime getRegistrationDate() {
return registrationDate;
}
public void setRegistrationDate(LocalDateTime registrationDate) {
this.registrationDate = registrationDate;
}
}
How it Works
- The
UserService
class uses WebClient to make an asynchronous HTTP GET request to the user API. - The
retrieve()
method initiates the request and thebodyToMono()
returns aMono<UserResponse>
that represents the response body. - The
LocalDateTime
field inUserResponse
is automatically deserialized by Spring, thanks to its built-in support for the ISO-8601 format.
Handling Error Responses
One of the significant advantages of using WebClient is its error handling capabilities. You can customize how errors are handled while requesting data.
Here's how you can implement error handling in your getUserById
method:
public Mono<UserResponse> getUserById(int userId) {
return webClient.get()
.uri("/api/users/{id}", userId)
.retrieve()
.onStatus(status -> status.is4xxClientError(), clientResponse ->
Mono.error(new RuntimeException("User not found"))
)
.onStatus(status -> status.is5xxServerError(), clientResponse ->
Mono.error(new RuntimeException("Server Error"))
)
.bodyToMono(UserResponse.class);
}
In this function, we evaluate the HTTP response's status. If it's a 4xx error, we throw a RuntimeException that can be handled elsewhere in our application. This approach leads to better error management and user experience.
Putting It All Together
Finally, let’s create a simple REST controller to expose our service.
Controller Class
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.MediaType;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
import reactor.core.publisher.Mono;
@RestController
public class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping(value = "/users/{id}", produces = MediaType.APPLICATION_JSON_VALUE)
public Mono<UserResponse> getUser(@PathVariable int id) {
return userService.getUserById(id);
}
}
Testing the API
You can use tools like Postman or curl to test your API. Simply hit the endpoint with a valid user ID.
curl http://localhost:8080/users/1
You should receive a response similar to:
{
"id": 1,
"name": "John Doe",
"registrationDate": "2023-09-15T10:15:30"
}
Key Takeaways
Properly handling date-time fields in Java while making network calls can dramatically improve the reliability of your applications. This blog discussed how to leverage Spring WebClient along with Java’s java.time
package for efficient HTTP communication with date-time data. The ability to handle errors gracefully and manage async requests makes Spring WebClient a robust choice for modern Java applications.
Additional Resources
With this knowledge, you're ready to start building more robust and efficient APIs!