Understanding Bean Scope Confusion in Spring MVC
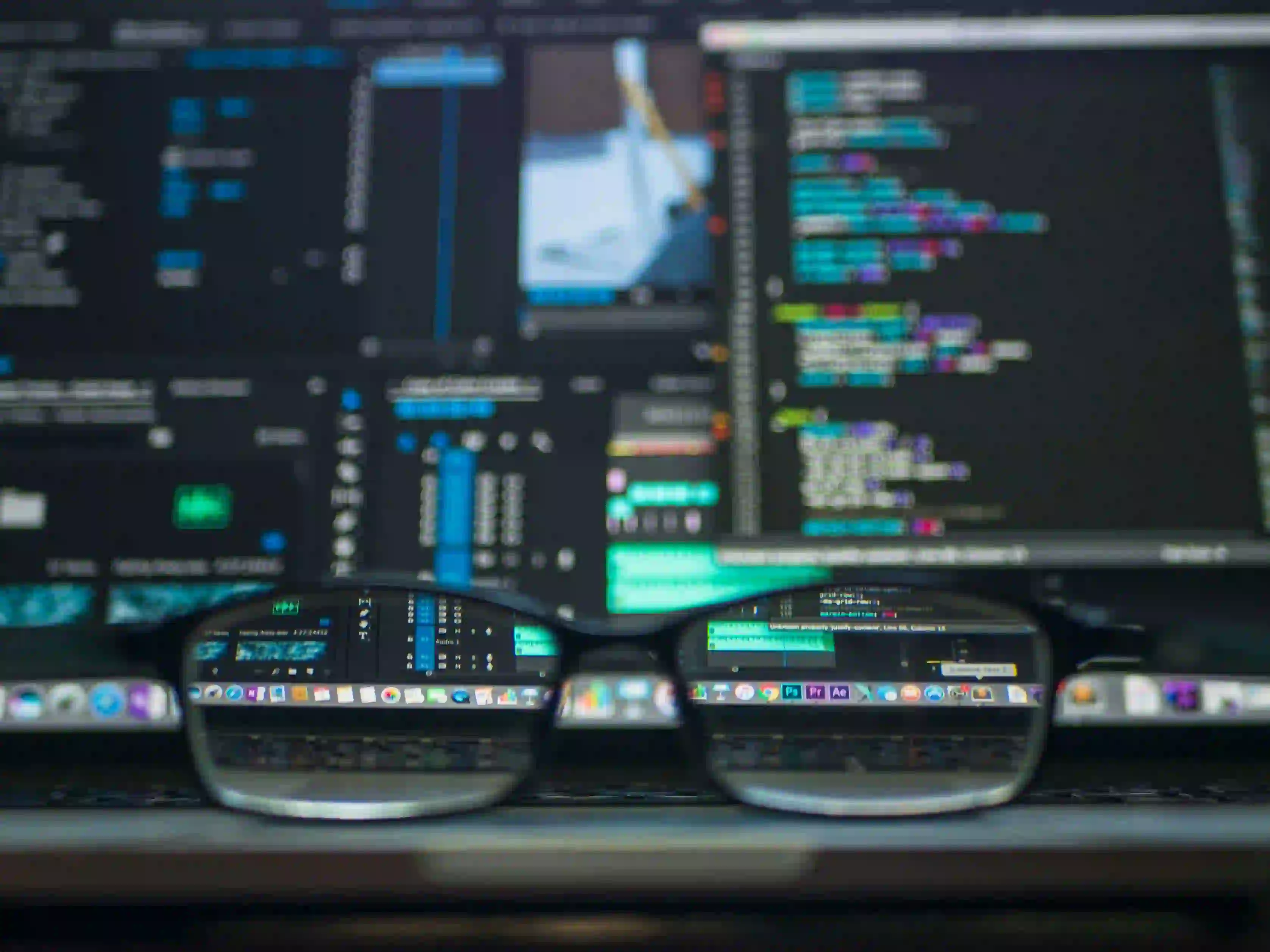
Understanding Bean Scope Confusion in Spring MVC
In the world of Java development, Spring Framework stands as a robust solution for building enterprise-level applications. One of its most powerful features is the concept of dependency injection, managed through the Spring Container. However, encountering confusion around bean scopes is common among developers. This blog post will clarify the different scopes of beans in Spring MVC, helping you make informed decisions when configuring your applications.
What is Spring Bean Scope?
Before diving into the various bean scopes, let's clarify what a Spring bean is. A Spring bean is an object that is instantiated, configured, and managed by the Spring IoC (Inversion of Control) container. The spring bean scope defines the lifecycle and visibility of a bean within the application.
The various bean scopes in Spring include:
- Singleton
- Prototype
- Request (Web-aware scope)
- Session (Web-aware scope)
- Global Session (Web-aware scope)
- Application (default in Spring)
1. Singleton Scope
The singleton scope is the default scope in Spring. When a bean is defined as a singleton, Spring creates exactly one instance of the bean defined in the application context. This means that all requests for that particular bean will return the same instance.
import org.springframework.stereotype.Component;
@Component
public class SingletonBean {
public SingletonBean() {
System.out.println("Singleton bean instance created!");
}
}
Why use Singleton Scope?
Singleton scope is particularly useful when you want to share a common state across your application. This is common for service layers and configuration classes, where a single instance needs to manage shared resources.
2. Prototype Scope
On the other hand, the prototype scope creates a new instance of the bean every time it is requested from the Spring container.
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Component;
@Component
@Scope("prototype")
public class PrototypeBean {
public PrototypeBean() {
System.out.println("Prototype bean instance created!");
}
}
Why use Prototype Scope?
The prototype scope is beneficial when the bean holds state that shouldn't be shared across the application. This is useful in cases of complex objects that may have state-dependent behavior.
3. Request Scope
When working with web applications, request scope is a valuable feature. A bean declared as request-scoped will be created and exist as long as the HTTP request is active.
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Component;
import org.springframework.web.context.WebApplicationContext;
@Component
@Scope(value = WebApplicationContext.SCOPE_REQUEST)
public class RequestScopedBean {
public RequestScopedBean() {
System.out.println("Request-scoped bean instance created!");
}
}
Why use Request Scope?
This is particularly helpful when you need a bean to maintain data for a single request. Examples include form processing or holding request-specific data.
4. Session Scope
Similar to the request scope, but with a broader lifecycle, a session-scoped bean will exist for the duration of an HTTP session. This allows for better state management when dealing with user sessions.
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Component;
import org.springframework.web.context.WebApplicationContext;
@Component
@Scope(value = WebApplicationContext.SCOPE_SESSION)
public class SessionScopedBean {
public SessionScopedBean() {
System.out.println("Session-scoped bean instance created!");
}
}
Why use Session Scope?
Session scope is useful in maintaining user-specific information across multiple requests in a web application. This is ideal for user preferences or login sessions.
5. Global Session Scope
The global session scope is intended for portlet applications. It is similar to session scope, but it is designed to span multiple portlet sessions across the entire global application context.
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Component;
import org.springframework.web.context.WebApplicationContext;
@Component
@Scope(value = WebApplicationContext.SCOPE_GLOBAL_SESSION)
public class GlobalSessionScopedBean {
public GlobalSessionScopedBean() {
System.out.println("Global session-scoped bean instance created!");
}
}
Why use Global Session Scope?
This scope is primarily used when dealing with multiple portlets or application contexts requiring the same session to be accessed globally.
6. Application Scope
Lastly, the application scope creates a bean instance that will be shared across the entire application context. Similar to singleton, but within a web application context.
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Component;
import org.springframework.web.context.WebApplicationContext;
@Component
@Scope(value = WebApplicationContext.SCOPE_APPLICATION)
public class ApplicationScopedBean {
public ApplicationScopedBean() {
System.out.println("Application-scoped bean instance created!");
}
}
Why use Application Scope?
Application scope is useful for stateful beans that should be shared across the entire application but must be different in different web application contexts.
Bean Scope Confusion: Common Pitfalls
-
Misunderstanding Scope Behavior: Developers often misinterpret how scopes behave, especially with singleton and prototype scopes. It's crucial to understand that singletons are great for shared configurations, while prototypes should be used for stateful objects.
-
Thread Safety: Singletons should be thread-safe since multiple threads may access the same instance simultaneously. In contrast, prototypes are inherently thread-safe as a new instance is created for every request.
-
Lazy Initialization Misuse: Developers might enable lazy initialization with singleton beans unnecessarily. Although it does postpone the creation of the bean, using it incorrectly can lead to inconsistencies that are difficult to debug.
-
Web Application Context: Not recognizing that web-aware scopes won't work without a web application context can lead to runtime exceptions. Make sure your beans are defined within a web application context when using these scopes.
A Final Look
Understanding the different bean scopes in Spring MVC is crucial for effective application design and management. Each scope comes with its own set of use cases and behaviors. By grasping these concepts, you can architect applications that are not only efficient but also maintainable.
For more details, check out the Spring Framework Documentation and the Spring Bean Scopes.
Armed with this knowledge, you'll be better prepared to handle the intricacies of Spring MVC and make choices that suit your application needs. Happy coding!