Overcoming Common Pitfalls with Java 8 Method References
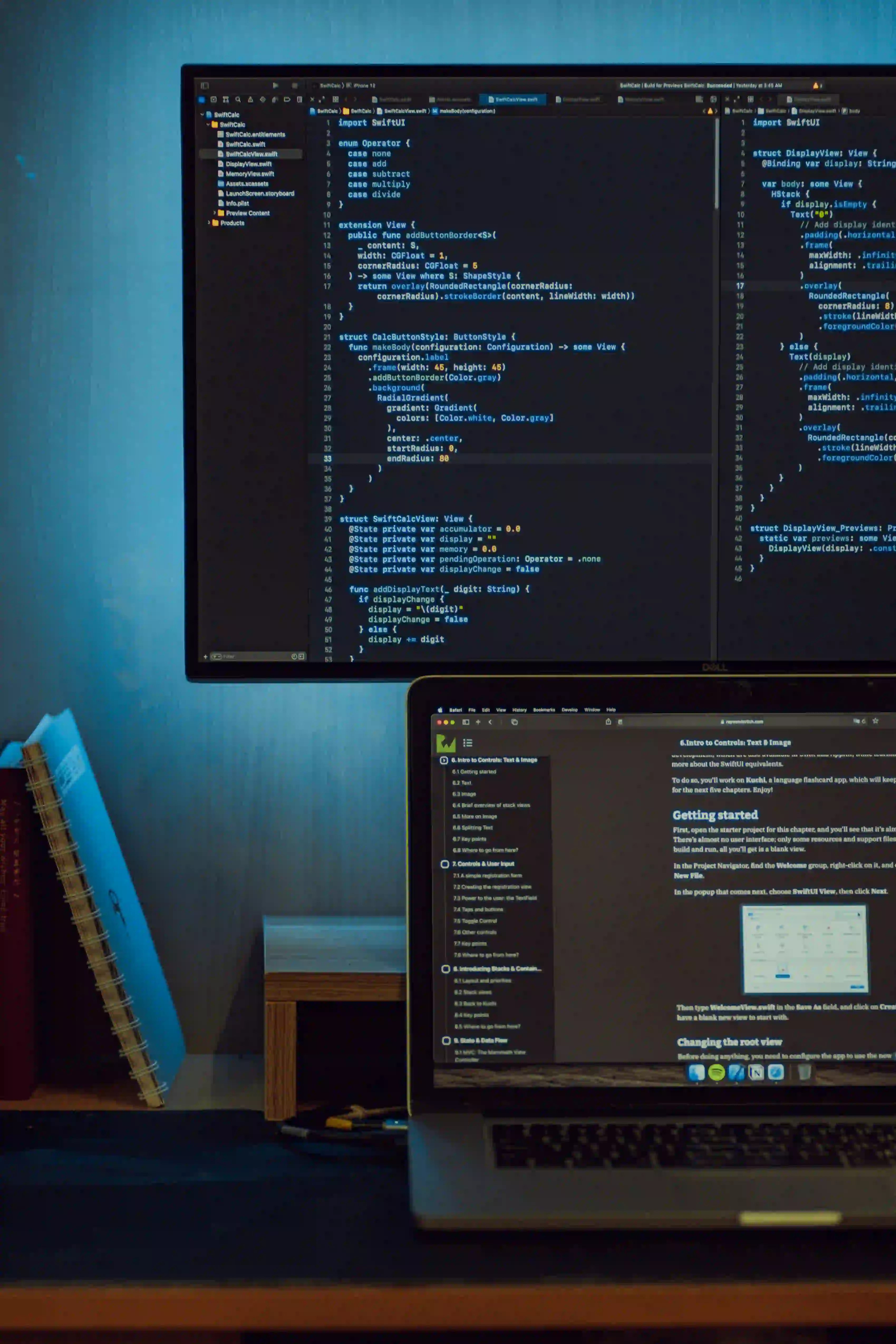
Overcoming Common Pitfalls with Java 8 Method References
When Java 8 introduced method references, it marked a significant evolution in the way developers could build applications. Method references provide a succinct way to refer to methods and streamline the implementation of functional interfaces. However, navigating this powerful feature can lead to some common pitfalls for developers, particularly those new to functional programming. This blog post will illuminate these pitfalls and provide guidance on how to effectively utilize method references in your Java applications.
Understanding Method References
Before diving into pitfalls, let's clarify what method references are. A method reference is a shorthand notation of a lambda expression to call a method. It allows you to refer to methods or constructors without needing to implement the method body explicitly.
Here's a basic example:
List<String> names = Arrays.asList("Anna", "Bob", "Charlie");
// Using a lambda expression
names.forEach(name -> System.out.println(name));
// Using a method reference
names.forEach(System.out::println);
In this example, System.out::println
serves as a method reference for the System.out.println(String)
method. The second approach is more concise and often more readable.
Common Pitfalls with Method References
1. Misunderstanding the Context
One major pitfall when using method references is misunderstanding their context. Method references require that the method being referenced matches the functional interface' expected behavior.
Example:
Suppose you have a functional interface:
@FunctionalInterface
interface StringModifier {
String modify(String input);
}
If you attempt to reference a method that does not conform to StringModifier
, you will run into issues.
public class Modifier {
public static String toUpperCase(String input) {
return input.toUpperCase();
}
}
// This works
StringModifier modifier = Modifier::toUpperCase;
// This does not work
StringModifier wrongModifier = String::valueOf; // Compile-time error
In this case, String::valueOf
will not compile because it does not match the expected signature of StringModifier
. Always ensure that the method reference aligns properly with the target functional interface.
2. Overusing Method References
Another common pitfall is overusing method references in situations where lambda expressions might be clearer. Method references can make code terse, but at times they can sacrifice readability.
Example:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.sort(Comparator.comparing(String::length).reversed());
While this is concise, if the logic behind sorting isn’t clear to new developers, it might confuse them. In such cases, a lambda might be easier to understand:
names.sort((s1, s2) -> Integer.compare(s2.length(), s1.length()));
In summary, choose clarity over brevity—particularly when your code will be read and maintained by others.
3. Ignoring Nulls
Another oversight developers often make is failing to account for nulls, which can lead to unexpected errors. When working with collections or streams, a null
value can throw a NullPointerException
.
List<String> names = Arrays.asList("Anna", null, "Charlie");
names.forEach(System.out::println); // This will print the name and then throw an exception
Solution: Always ensure that your data is non-null or handle potential nulls during processing, especially if there's a possibility of encountering them.
names.stream()
.filter(Objects::nonNull) // Issoignores nulls
.forEach(System.out::println);
4. Static and Instance Context Confusion
Another common pitfall arises from confusion between static and instance method references. It's important to choose the correct context for your method reference.
This example demonstrates a common confusion:
public class Example {
public void instanceMethod() {
System.out.println("Instance method called");
}
public static void staticMethod() {
System.out.println("Static method called");
}
}
// Wrong: trying to refer to an instance method from a static context
Runnable r = Example::instanceMethod; // Compile-time error
// Correct usage
Example example = new Example();
Runnable r = example::instanceMethod; // This works
Static methods can be referred to directly without an instance, while instance methods require an object reference.
5. Forgetting Overloaded Methods
Method references can lead to ambiguity when dealing with overloaded methods. Java doesn't know which overload to choose based on a method reference alone unless the context of the functional interface clears it up.
Example:
public class Overloaded {
public static void print(int number) {
System.out.println("Integer: " + number);
}
public static void print(String text) {
System.out.println("String: " + text);
}
}
// This will cause a compile-time error
Consumer c = Overloaded::print; // Ambiguous
To avoid this, ensure that the functional interface context clears up which method you mean:
Consumer<Integer> intConsumer = Overloaded::print; // Resolved to int version
Consumer<String> stringConsumer = Overloaded::print; // Resolved to string version
The Bottom Line
Java 8 method references are a powerful feature that can greatly improve the readability and conciseness of your code. However, it is essential to be aware of common pitfalls to avoid potential issues.
By understanding the context, choosing clarity over brevity, handling null values gracefully, using the correct static or instance method references, and being cautious with overloaded methods, you can harness the full potential of method references without falling into traps that lead to bugs and maintainability issues.
For further reading, consider checking out these Java documentation resources:
By keeping these principles in mind, you will be well-equipped to incorporate method references effectively in your Java applications. Happy coding!