How to Resolve JSON Parsing Errors with Jackson
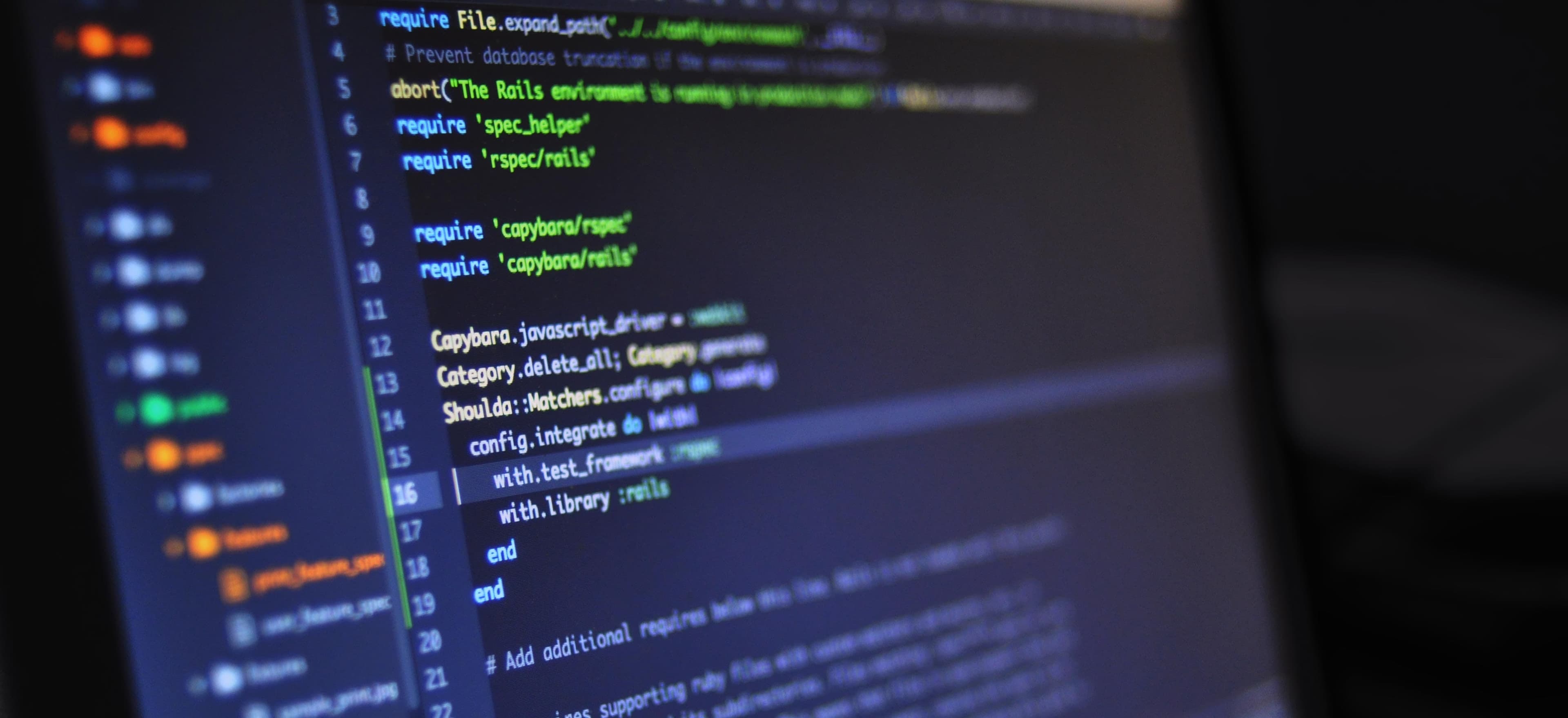
- Published on
How to Resolve JSON Parsing Errors with Jackson
In the world of software development, JSON (JavaScript Object Notation) has become one of the most prevalent formats for transmitting and storing data. Its lightweight and human-readable format makes it a favorite choice for RESTful APIs and many web services. With the use of libraries like Jackson, parsing JSON in Java has never been easier. However, developers often come face-to-face with JSON parsing errors that can halt their progress. In this blog post, we will explore common JSON parsing errors in Jackson and provide practical solutions to resolve them.
What is Jackson?
Jackson is a popular Java library used for processing JSON. This powerful library allows developers to convert Java objects to JSON and vice versa seamlessly. With Jackson's streaming, databind, and annotation features, it stands as a fundamental tool in a Java developer's toolkit.
For those new to Jackson, you can check out Jackson's official documentation to get started.
Common JSON Parsing Errors
When working with JSON in Jackson, some errors frequently arise:
- Unexpected token errors
- Mismatched types
- Missing fields
- Invalid JSON structure
Let’s dive into each of these errors with examples and solutions.
1. Unexpected Token Errors
What is it?
This error occurs when Jackson encounters a token in the JSON that does not match the expected format based on the defined class structure.
Example:
Imagine we have the following JSON string:
{
"name": "John",
"age": "30" // Age is provided as a string
}
And we are trying to parse it into a Person class defined as follows:
public class Person {
private String name;
private int age;
// Getters and setters omitted for brevity
}
When you run the code to parse this JSON, you might see:
Unexpected token (VALUE_STRING), expected VALUE_NUMBER_INT
Solution:
To solve this, ensure that the types in your JSON match those in your Java class. You can either modify your JSON data or switch your age property to String
:
public class Person {
private String name;
private String age; // Changed to String to match JSON
}
2. Mismatched Types
What is it?
This error occurs when the JSON data type does not correspond to the Java class data type. Type mismatches can cause parsing errors that halt execution.
Example:
Consider the following JSON:
{
"name": "Alice",
"age": 25,
"isMarried": false
}
With the same Person class as before, if we introduce a new property, isMarried, of a different type:
public class Person {
private String name;
private int age;
private String isMarried; // This should be a boolean
}
Parsing this JSON would lead to an error like:
Cannot deserialize value of type `java.lang.Boolean` from Boolean value ('false')
Solution:
Make sure the types match correctly in your Java class. Update the isMarried field to a boolean:
public class Person {
private String name;
private int age;
private boolean isMarried; // Changed to boolean
}
3. Missing Fields
What is it?
Jackson can handle missing fields in JSON, but that may lead to default values in Java objects. This can sometimes introduce confusion in your application.
Example:
With the following JSON:
{
"name": "Bob"
}
And a Person class:
public class Person {
private String name;
private int age; // Age is omitted in JSON
// Getters and setters omitted for brevity
}
When you attempt to parse this JSON, the age field will be set to its default value (0
), potentially leading to incorrect logic in your application.
Solution:
Consider using annotations to control how Jackson handles missing fields. You can utilize the @JsonProperty
annotation to set default values or require fields:
public class Person {
private String name;
@JsonProperty(defaultValue = "18") // Default age if not provided
private int age;
// Getters and setters omitted for brevity
}
4. Invalid JSON Structure
What is it?
This error arises when the JSON is not correctly formatted, which will prevent Jackson from parsing it entirely.
Example:
An invalid JSON structure like:
{
"name": "Eve",
"age": 30,
}
Notice the trailing comma after 30
? This structure is deemed invalid.
Solution:
Ensure that your JSON is correctly formatted. If you want to validate your JSON manually, consider using JSONLint. Alternatively, you can use a try-catch block in your code to catch JsonParseException
quickly:
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.databind.ObjectMapper;
ObjectMapper objectMapper = new ObjectMapper();
try {
String jsonString = "{ \"name\": \"Eve\", \"age\": 30, }"; // Invalid JSON
Person person = objectMapper.readValue(jsonString, Person.class);
} catch (JsonParseException e) {
System.out.println("Invalid JSON structure: " + e.getMessage());
}
Best Practices for Handling JSON Parsing Errors
-
Validate Your JSON: Always validate your JSON files or strings using a validator before processing them.
-
Use Proper Annotations: Jackson provides various annotations like
@JsonProperty
,@JsonIgnore
, and@JsonInclude
to control serialization and deserialization effectively. -
Implement Error Handling: Always implement try-catch blocks to handle potential exceptions gracefully.
-
Keep JSON Structures Simple: Complex nested structures can lead to more errors. Try to keep it as simple as possible, especially when interfacing with multiple APIs.
-
Use DTOs: Utilize Data Transfer Objects (DTOs) to encapsulate incoming JSON structures. This approach makes it easier to manage and troubleshoot parsing errors.
Key Takeaways
JSON parsing is a critical concern in modern Java applications, especially when working with REST APIs or web services. While using Jackson can streamline the process, understanding common parsing errors and how to resolve them is essential for a smooth development experience. By following the strategies and best practices outlined in this post, you can significantly reduce the time spent debugging JSON parsing issues.
Whether you're a beginner or an experienced Java developer, mastering JSON parsing with Jackson is key to building robust, data-driven applications. Ready to start parsing and handling JSON? Dive into your project today!
If you'd like to learn more about handling data in Jackson, check out Baeldung's guide on Jackson for more in-depth knowledge. Happy coding!