Mastering Distributed Tracing: Common Pitfalls with Zipkin
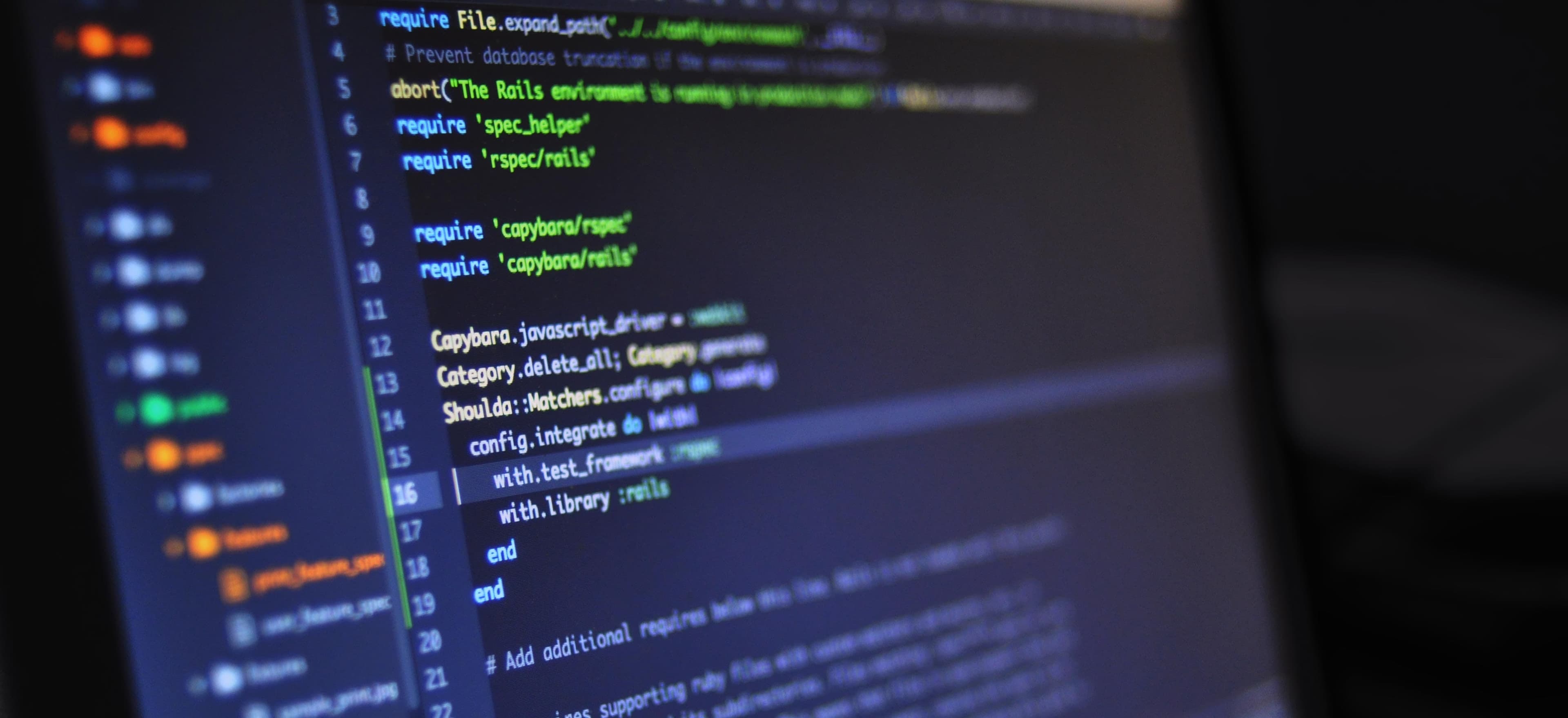
- Published on
Mastering Distributed Tracing: Common Pitfalls with Zipkin
In the fast-paced world of software development, understanding how to effectively trace requests across distributed systems is essential. As microservices architectures gain popularity, so does the need for monitoring solutions like Zipkin. Zipkin aids in troubleshooting latency and performance issues. However, leveraging Zipkin efficiently requires navigating through potential pitfalls. This blog post aims to shed light on these challenges, articulate their significance, and guide you through best practices for successful implementation.
What is Distributed Tracing?
Distributed tracing is a methodology used for monitoring applications through tracing calls distributed across multiple services. It enables developers and operators to analyze the path of requests, identify bottlenecks, and enhance overall application performance.
Why Zipkin?
Zipkin is an open-source tracing system that helps gather performance data and monitor microservices. It provides a solid base for understanding traces, visualizing the call flow, and debugging complex interactions.
Common Pitfalls in Using Zipkin
While Zipkin provides significant advantages, improper implementation can lead to several pitfalls. Here are some common issues you might encounter:
1. Incomplete Trace Context Propagation
When a request moves through multiple services, trace context needs to be passed effectively. Failure to propagate context leads to fragmented traces that do not give a complete overview of the request lifecycle.
Solution:
Always include the tracing context in service calls. See the code snippet below:
import brave.Tracing;
import brave.Tracer;
import brave.propagation.StringPropagation;
public class TraceService {
private final Tracer tracer;
public TraceService(Tracing tracing) {
this.tracer = tracing.tracer();
}
public void processRequest() {
// Start a new trace
try (Tracer.SpanInScope spanInScope = tracer.withSpanInScope(tracer.nextSpan())) {
// Perform operations here
}
}
}
Why? This ensures that every invocation includes tracing information, allowing for complete trace visibility. Always make it a habit to wrap service calls with trace spans.
2. Failure to Leverage Annotations
Annotations can provide insightful context about operations during the lifecycle of a request. Ignoring this can result in losing crucial insights.
Solution:
Inject annotations into your spans that signify key events or errors. Here's an example:
public void orderService() {
Span span = tracer.nextSpan().name("order-processing").start();
try (Tracer.SpanInScope ws = tracer.withSpanInScope(span)) {
// Simulating order processing
if (orderFailed) {
span.annotate("Order processing failed");
// Error handling logic
}
// Normal flow
} finally {
span.finish();
}
}
Why? By annotating important events, you get a granular view of your application's behavior, enabling targeted troubleshooting.
3. Ignoring Performance Overhead
Another pitfall involves the performance overhead introduced by tracing — particularly when the logging volume is high. Suboptimal configurations can affect application performance.
Solution:
Configuring an appropriate sampling rate can mitigate this issue. The following snippet demonstrates configuring Zipkin for sampling:
zipkin.sampler.type=PROBABILITY
zipkin.sampler.probability=0.1
Why? This configuration allows you to collect 10% of requests, providing insights without overwhelming system resources. Tune the sampling rate based on your system’s needs.
4. Inconsistent Span Naming Conventions
Inconsistent naming can make it challenging to understand the traces. Without uniformity, developers may get lost when analyzing spans.
Solution:
Establish a naming convention and documentation that outlines how to name spans appropriately. An example could be:
public void paymentService() {
Span span = tracer.nextSpan().name("payment-processing").start();
...
}
Why? Consistent span naming helps in recognizing patterns, leading to a clearer understanding of application flow and facilitating quicker resolution of issues.
5. Failing to Set Proper Timeouts
Ignoring timeouts can lead to traces that hang indefinitely, making it hard to diagnose issues. Properly managing timeouts is crucial.
Solution:
Set clear timeouts for your services to prevent such scenarios:
ScheduledExecutorService executorService = Executors.newScheduledThreadPool(1);
Callable<Object> task = () -> {
// Service operation
return null;
};
Future<Object> future = executorService.submit(task);
try {
future.get(5, TimeUnit.SECONDS);
} catch (TimeoutException e) {
// Handle timeout
}
Why? This practice allows your application to gracefully handle scenarios where requests take too long, ensuring that your tracing data remains actionable.
6. Lack of Visualization Tools
Without proper visualization, it can be challenging to make sense of trace data. Raw trace logs offer limited insights when isolated from appropriate UI representation.
Solution:
Integrate Zipkin with visualization tools to better understand your application's behavior. Using Zipkin’s own UI dashboard can provide a visual representation of traces, making it easier to analyze.
Why? Interactive visualizations help you immediately see relationships and dependencies between calls, turning data into actionable intelligence.
In Conclusion, Here is What Matters
Distributed tracing with Zipkin offers tremendous value for developers working with modern microservices. By following best practices and being aware of the common pitfalls, you can leverage this powerful tool effectively. Complete trace propagation and well-defined conventions around span naming not only ease the troubleshooting process but facilitate profound insights into your application’s performance.
For further reading and deeper dives into distributed tracing, explore Zipkin's official documentation or check out The Twelve-Factor App methodology for microservices best practices.
By being mindful of the challenges discussed, and applying the recommended solutions, mastering distributed tracing will no longer be an arduous task. Instead, it will become an essential part of your development and monitoring toolkit.