Smoothly Transitioning from Ant to Maven: Common Pitfalls
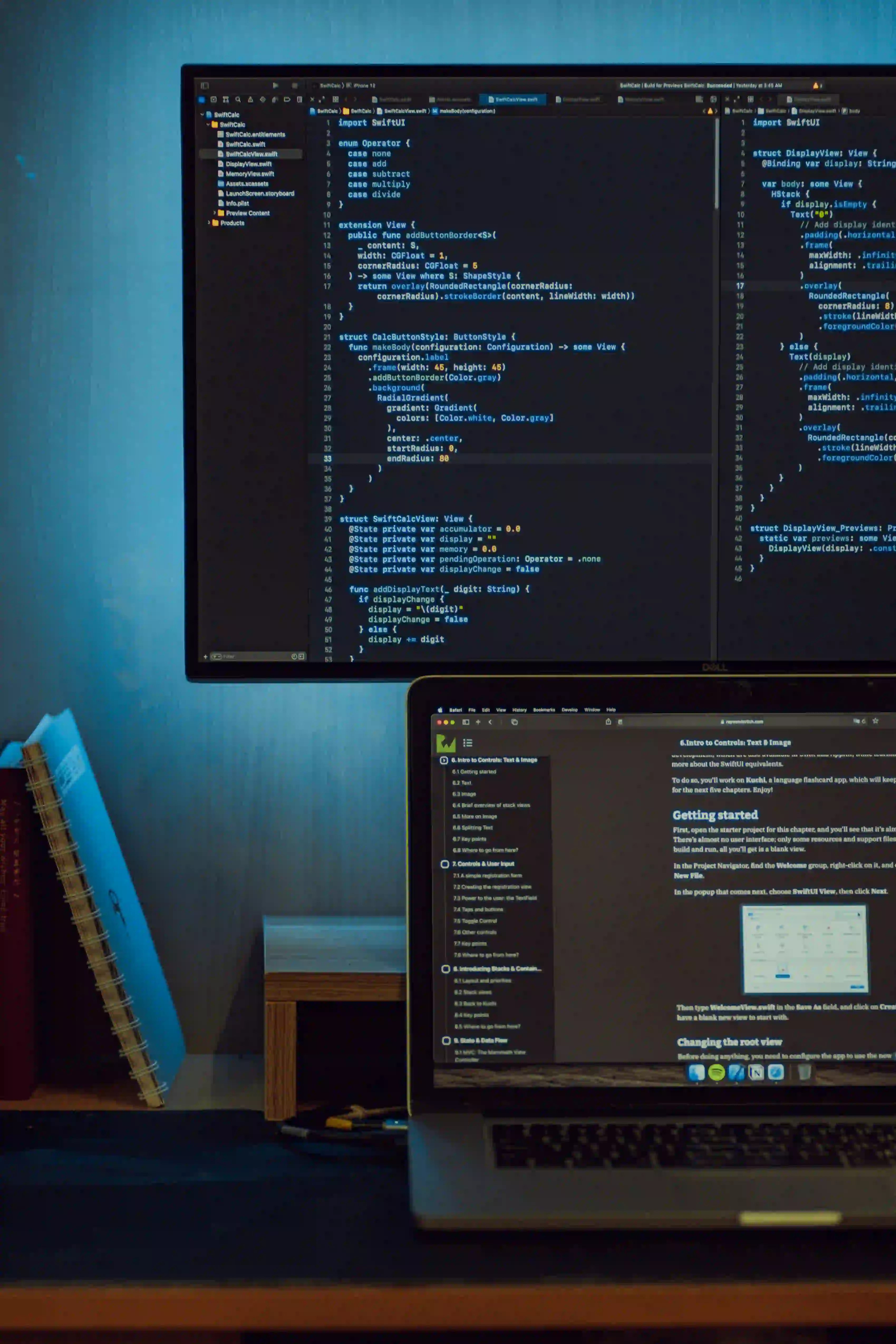
Smoothly Transitioning from Ant to Maven: Common Pitfalls
In the world of Java development, build tools play a crucial role in automating and managing project builds, dependencies, and packaging. Two popular build tools that Java developers often use are Ant and Maven. While Ant has served many developers well, Maven has gained more traction due to its convention-over-configuration approach and powerful dependency management capabilities.
Making the shift from Ant to Maven can undoubtedly streamline your projects, but it is not without challenges. This blog post explores some common pitfalls developers encounter during this transition and how to avoid them, ensuring a smooth transition.
Understanding the Basics: Ant vs. Maven
Before jumping into the transition, let’s establish a foundational understanding of the two tools.
Ant Characteristics:
- Imperative Build Process: Ant allows developers to define the explicit steps required to build their project. Each step (target) is defined using XML and executed sequentially.
- Flexibility: With Ant, you have complete control over the build process, enabling custom tasks and configurations.
- No Dependency Management: Ant does not include built-in dependency management. Developers have to handle and download dependencies manually or use additional tools like Apache Ivy.
Maven Characteristics:
- Declarative Build Process: Maven emphasizes a convention-over-configuration model. Developers simply declare their project structure and dependencies.
- Dependency Management: Maven automatically downloads and manages project dependencies, minimizing the overhead on developers.
- Plugin System: Maven’s powerful plugin ecosystem provides extensive capabilities that can easily integrate into the build process.
Common Pitfalls During Transition
1. Misunderstanding the Maven Model
Maven operates on a specific lifecycle (validate, compile, test, package, verify, install, deploy). Many developers transitioning from Ant may not grasp this model initially.
Solution: Familiarize yourself with Maven’s lifecycle and phases. A good rule of thumb is to think about what you want to accomplish and then map it to the relevant Maven phase.
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-project</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
</dependencies>
</project>
In the example above, we define a basic Maven project with a dependency on Apache Commons Lang. By understanding its lifecycle, you can effectively manage similar dependencies.
2. Overcomplicating the POM Structure
When migrating from Ant, many developers tend to overcomplicate their pom.xml
file. They attempt to recreate complex Ant build scripts in Maven instead of embracing its simplicity.
Solution: Start with a minimal pom.xml
. Gradually add complexity as you understand how Maven works. Focus on the essential parts first.
3. Ignoring the Power of Plugins
Ant users often rely on custom scripts for specific tasks. In Maven, a plethora of plugins are available to perform these tasks.
Solution: Research and utilize Maven’s extensive plugin repository. Find plugins that meet your needs and incorporate them.
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
</build>
In this code snippet, the Maven Compiler Plugin is configured to compile your Java code to version 1.8. This showcases how plugins can replace custom scripts in Ant.
4. Dependency Management Confusion
Moving to Maven means grappling with its dependency management system. Many developers overlook transitive dependencies (dependencies of dependencies) leading to version conflicts.
Solution: Use the mvn dependency:tree
command to visualize your project's dependency tree, allowing you to resolve conflicts.
5. Failing to Embrace Convention Over Configuration
One of the strengths of Maven is its conventions. Many developers coming from Ant may insist on modifying defaults based on their previous configurations.
Solution: Embrace Maven's structure. It may feel restrictive initially but will save you time and effort in the long run.
6. Not Leveraging Profiles
Maven supports profiles, which allow you to define different settings for development, testing, and production.
Solution: Use profiles to manage your environments effectively. For instance, you can create different configurations for production versus development environments.
<profiles>
<profile>
<id>dev</id>
<properties>
<environment>dev</environment>
</properties>
</profile>
<profile>
<id>prod</id>
<properties>
<environment>prod</environment>
</properties>
</profile>
</profiles>
This code shows a simple configuration for two environments. Transitioning away from Ant’s manual approaches helps you to utilize Maven’s capabilities.
Additional Considerations
Transitioning isn't just tactical; it’s also about mindset. Here are a few considerations to keep in mind:
- Gradually Migrate Projects: Avoid transitioning all projects at once. Experience with a single project can significantly ease the transition for future projects.
- Utilize Resources: Utilize online resources, forums, and documentation. Platforms like the Maven User Mailing List can provide invaluable guidance.
- Conduct Training: Implement training sessions within your team to align everyone's understanding of Maven.
My Closing Thoughts on the Matter
Transitioning from Ant to Maven can be challenging, and it often involves avoiding common pitfalls that stem from a lack of understanding of Maven’s paradigms. By recognizing the strengths of each tool and embracing Maven’s capabilities, developers can enhance their productivity and streamline the building process.
If you want a deeper understanding of Maven and its features, consider visiting the official Maven documentation. The journey may be daunting, but the efficiencies gained through Maven are worth the investment. Happy transitioning!