Java Strategies for Efficient API Pagination and Filtration
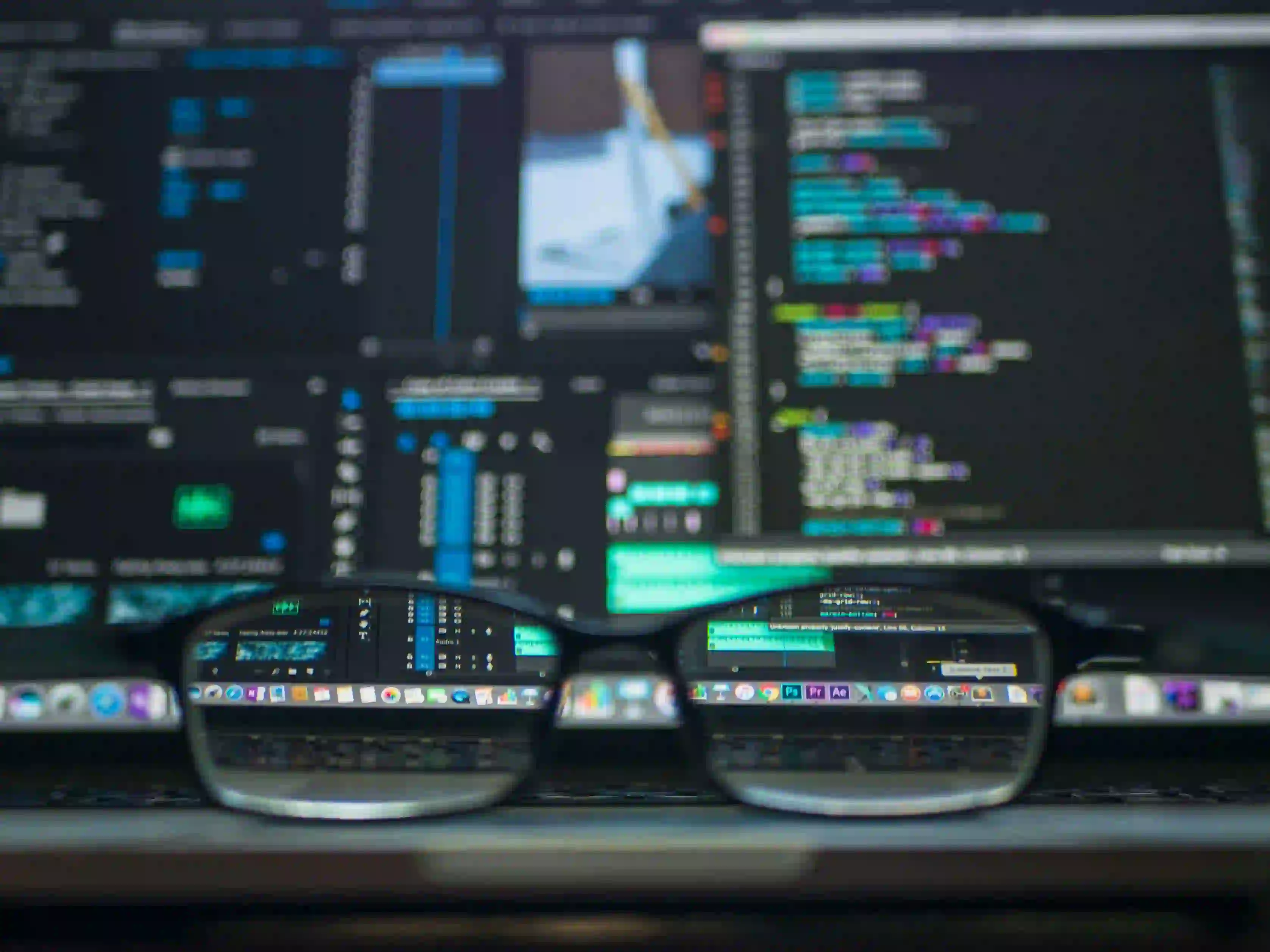
Java Strategies for Efficient API Pagination and Filtration
API development is a cornerstone of modern web applications. With the ever-increasing amounts of data, managing how that data is served to users becomes crucial. This is where effective pagination and filtration come into play. In this post, we'll explore Java strategies for efficiently implementing these features in your APIs.
If you're looking for more on SQL-based solutions, check out the article titled Optimizing APIs: Mastering SQL Pagination & Filtration. It provides invaluable insights which will complement the Java strategies discussed here.
Understanding Pagination
Pagination refers to the process of dividing large datasets into smaller, manageable chunks, or "pages." The primary goal is to enhance user experience by loading data incrementally—this means users aren’t overwhelmed by too much information at once.
In Java, integrating pagination into your API usually involves some combination of query parameters for specifying the page number and the number of items per page. Let's delve into a basic example.
Basic Pagination Example
Here's a simple implementation of pagination in a Java REST API using Spring Boot and JPA.
@RestController
@RequestMapping("/items")
public class ItemController {
@Autowired
private ItemService itemService;
@GetMapping
public ResponseEntity<Page<Item>> getItems(
@RequestParam(defaultValue = "0") int page,
@RequestParam(defaultValue = "10") int size) {
Page<Item> items = itemService.getItems(PageRequest.of(page, size));
return new ResponseEntity<>(items, HttpStatus.OK);
}
}
Why This Works
- PageRequest.of(page, size): This method constructs a new instance of
PageRequest
, which contains the specified page number and size. This format helps JPA know how to segment the data into pages. - ResponseEntity: It encapsulates the response, allowing you to return additional HTTP metadata such as status codes.
Implementing Filtration
Alongside pagination, avenues for filtering data based on specific criteria must be available. This ensures that users can locate the information they need without scrolling through numerous entries.
Filter Implementation
Using the Specification
interface in Spring Data JPA, you can create dynamic queries. Here’s an example showcasing how to filter items based on specific criteria.
public List<Item> filterItems(String category, String searchQuery) {
return itemRepository.findAll((root, criteriaQuery, criteriaBuilder) -> {
List<Predicate> predicates = new ArrayList<>();
if (category != null) {
predicates.add(criteriaBuilder.equal(root.get("category"), category));
}
if (searchQuery != null) {
predicates.add(criteriaBuilder.like(root.get("name"), "%" + searchQuery + "%"));
}
return criteriaBuilder.and(predicates.toArray(new Predicate[0]));
});
}
Why Use Specifications?
- Dynamic Control: Specifications allow for powerful, dynamic query generation depending on the user input and needs.
- Separation of Concerns: The query logic is separated from controller logic, making the codebase cleaner and more maintainable.
Combining Pagination and Filtration
Now that you understand the individual concepts, let's combine both pagination and filtration in one API endpoint.
@GetMapping
public ResponseEntity<Page<Item>> getFilteredItems(
@RequestParam(defaultValue = "0") int page,
@RequestParam(defaultValue = "10") int size,
@RequestParam(required = false) String category,
@RequestParam(required = false) String searchQuery) {
Page<Item> items = itemService.findAllWithFilters(PageRequest.of(page, size), category, searchQuery);
return new ResponseEntity<>(items, HttpStatus.OK);
}
Why is This Important?
- User Experience: By combining pagination and filtering, users can home in on specific data without wading through irrelevant items. This keeps the application fast and efficient.
- Performance: Optimizing how data is retrieved and rendered will reduce server load and improve API response times.
Best Practices for API Design
In addition to implementing pagination and filtration, consider these best practices for designing your API.
1. Use HTTP Status Codes Appropriately
Returning the correct HTTP status codes helps clients handle errors effectively. For instance, return a 404 Not Found
for requests where no data matches given criteria.
2. Document Your API
Make sure your API endpoints are well-documented. Tools like Swagger UI or Spring Rest Docs can help in visualizing the API’s capabilities, showing clients how to interact with it.
3. Handle Edge Cases
Always validate your input. Implement checks and balances to ensure that downtimes are reduced and potential exploits are mitigated.
4. Caching
Consider implementing caching mechanisms for frequently accessed data. This reduces the load on your database and speeds up API responses.
The Last Word
Implementing efficient pagination and filtration in your Java APIs can greatly enhance user experience and system performance. By utilizing Spring Data JPA's powerful features alongside best practices, you can create a robust, efficient backend.
As you explore these strategies, refer back to the article on Optimizing APIs: Mastering SQL Pagination & Filtration for SQL-centric techniques that can further enhance your understanding and execution.
With these insights, you are now equipped to enhance your Java API's performance significantly. Happy coding!