Efficient Data Handling in Java: Tackle SQL Pagination Issues
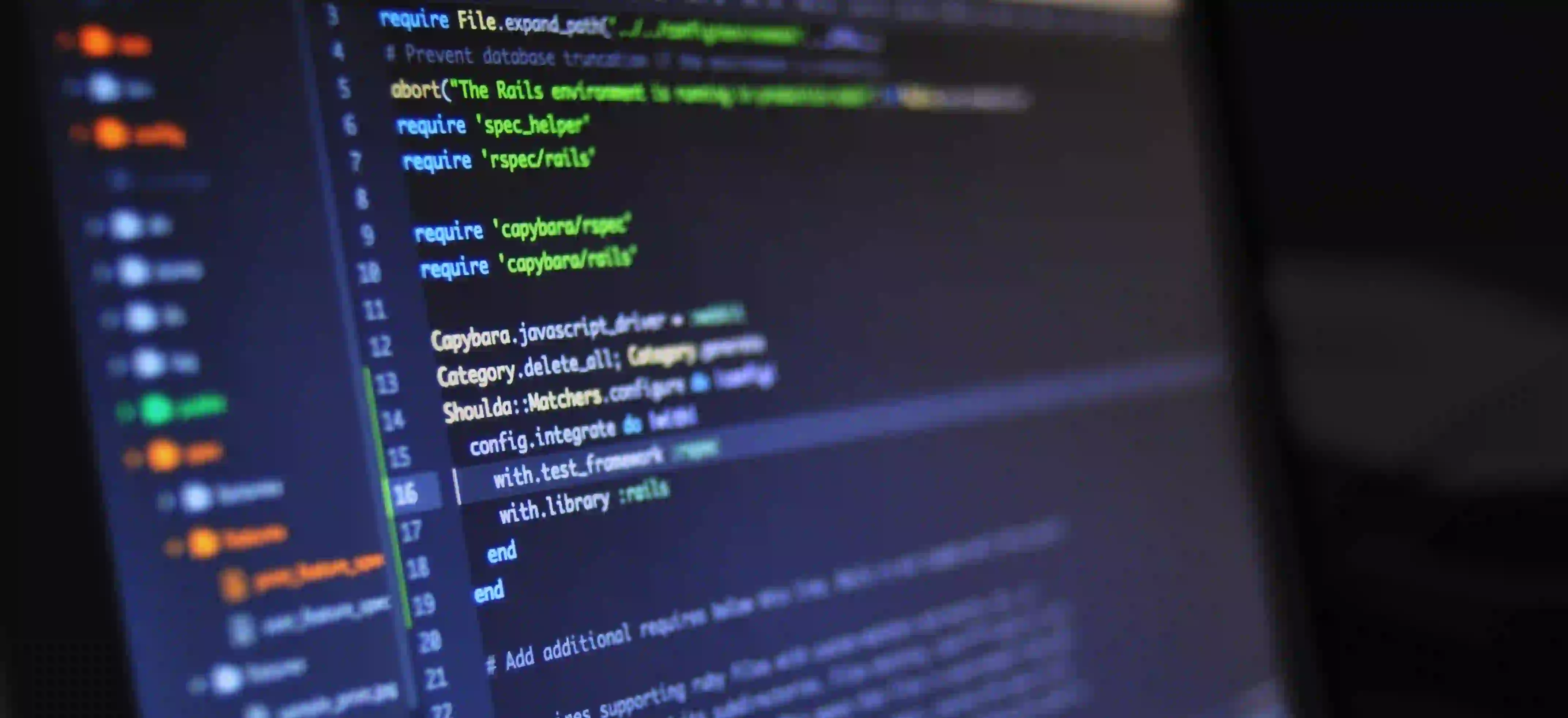
Efficient Data Handling in Java: Tackle SQL Pagination Issues
In an age where applications are increasingly driven by data, efficient data handling is paramount. Pagination plays a crucial role, especially when retrieving large datasets from a database. This blog post aims to explore SQL pagination issues and provide Java solutions to optimize data handling. By implementing effective strategies, you can streamline your API performance and enhance user experience.
What is Pagination?
Pagination is the process of dividing content into discrete pages. In the context of databases, it allows you to fetch data in smaller chunks rather than loading entire datasets at once. This is particularly useful when dealing with large databases. For instance, loading user data in batches of 10 or 20 records rather than all at once saves time and resources.
Why Pagination is Important
- Performance: Loading large datasets all at once can lead to slow response times.
- User Experience: Users can navigate through information easily.
- Resource Management: Minimizes memory consumption, leading to a more stable application.
Common Pagination Techniques
When interacting with SQL databases, you can employ several techniques for pagination:
- OFFSET and LIMIT: The simplest method to fetch a specific range of rows.
- Keyset-based Pagination: More efficient for deep pagination as it uses indexed columns for fetching records.
- Cursor Pagination: Uses a unique identifier to fetch records, providing consistent results.
Let's dive deeper into these methodologies with Java sample code snippets to illustrate the implementation.
1. OFFSET and LIMIT Pagination
This approach is straightforward and widely used.
SQL Query Example
SELECT * FROM users ORDER BY id LIMIT 10 OFFSET 20;
Java Implementation
// Pagination method using OFFSET and LIMIT
public List<User> getUsers(int page, int pageSize) {
String sql = "SELECT * FROM users ORDER BY id LIMIT ? OFFSET ?";
try (Connection conn = DriverManager.getConnection(url, user, password);
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setInt(1, pageSize); // Set limit
pstmt.setInt(2, page * pageSize); // Set offset
ResultSet rs = pstmt.executeQuery();
List<User> users = new ArrayList<>();
while (rs.next()) {
User user = new User();
user.setId(rs.getInt("id"));
user.setName(rs.getString("name"));
users.add(user);
}
return users;
} catch (SQLException e) {
e.printStackTrace();
}
return Collections.emptyList();
}
Why This Works
This method efficiently retrieves a specified number of rows based on page size and offset. However, it can become less efficient with deep pages, as the database must scan through the entire dataset every time.
2. Keyset-based Pagination
Keyset-based pagination is superior for scenarios where performance is crucial, especially with large datasets. This method uses a unique identifier (usually an indexed column) to fetch records.
SQL Query Example
SELECT * FROM users WHERE id > ? ORDER BY id LIMIT 10;
Java Implementation
// Keyset-based pagination method
public List<User> getUsersAfter(int lastId, int pageSize) {
String sql = "SELECT * FROM users WHERE id > ? ORDER BY id LIMIT ?";
try (Connection conn = DriverManager.getConnection(url, user, password);
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setInt(1, lastId); // Set last ID
pstmt.setInt(2, pageSize); // Set limit
ResultSet rs = pstmt.executeQuery();
List<User> users = new ArrayList<>();
while (rs.next()) {
User user = new User();
user.setId(rs.getInt("id"));
user.setName(rs.getString("name"));
users.add(user);
}
return users;
} catch (SQLException e) {
e.printStackTrace();
}
return Collections.emptyList();
}
Why This Works
This approach reduces the overhead of scanning the previous records, resulting in faster data retrieval. It is particularly effective for real-time applications where speed is essential.
3. Cursor Pagination
Cursor pagination involves passing around a marker that indicates the last processed record. This offers consistency but at the expense of complexity.
SQL Query Example
SELECT * FROM users WHERE created_at > ? ORDER BY created_at LIMIT 10;
Java Implementation
// Cursor pagination method
public List<User> getUsersAfterDate(Timestamp lastRetrieved, int pageSize) {
String sql = "SELECT * FROM users WHERE created_at > ? ORDER BY created_at LIMIT ?";
try (Connection conn = DriverManager.getConnection(url, user, password);
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setTimestamp(1, lastRetrieved); // Set last retrieved timestamp
pstmt.setInt(2, pageSize); // Set limit
ResultSet rs = pstmt.executeQuery();
List<User> users = new ArrayList<>();
while (rs.next()) {
User user = new User();
user.setId(rs.getInt("id"));
user.setName(rs.getString("name"));
users.add(user);
}
return users;
} catch (SQLException e) {
e.printStackTrace();
}
return Collections.emptyList();
}
Why This Works
Cursor pagination is beneficial in scenarios where records get inserted regularly. It gives predictable results without skipping any records.
Optimizing Your APIs with Pagination
Optimizing APIs is crucial for maintaining a performant application. Proper pagination not only helps in managing database load but also enhances overall performance. If you are interested in delving deeper into this topic, refer to the article Optimizing APIs: Mastering SQL Pagination & Filtration.
Additional Tips for Pagination
- Use Indexing: Ensure the columns used for filtering are indexed to speed up queries.
- Limit Select Clause: Instead of selecting all columns, select only those you need.
- Cache Frequently Accessed Data: Implement caching strategies for data that changes infrequently.
To Wrap Things Up
Efficient data pagination is not merely a choice but a necessity for any application dealing with significant datasets. It enhances performance, maintains resource efficiency, and ultimately leads to a better user experience. By applying methods like OFFSET and LIMIT, keyset-based pagination, and cursor pagination, you can effectively manage your data handling in Java.
Creating a seamless and performant application requires careful planning, especially when dealing with databases. By understanding these pagination methods and integrating them into your APIs, you can unlock the full potential of your applications.
For more insights on optimizing your APIs, revisit our recommended article on pagination and filtration strategies.
By following these best practices and utilizing the code snippets we've discussed, you're well on your way to mastering SQL pagination in Java. Happy coding!