Efficient Data Fetching: Java Solutions for SQL Pagination
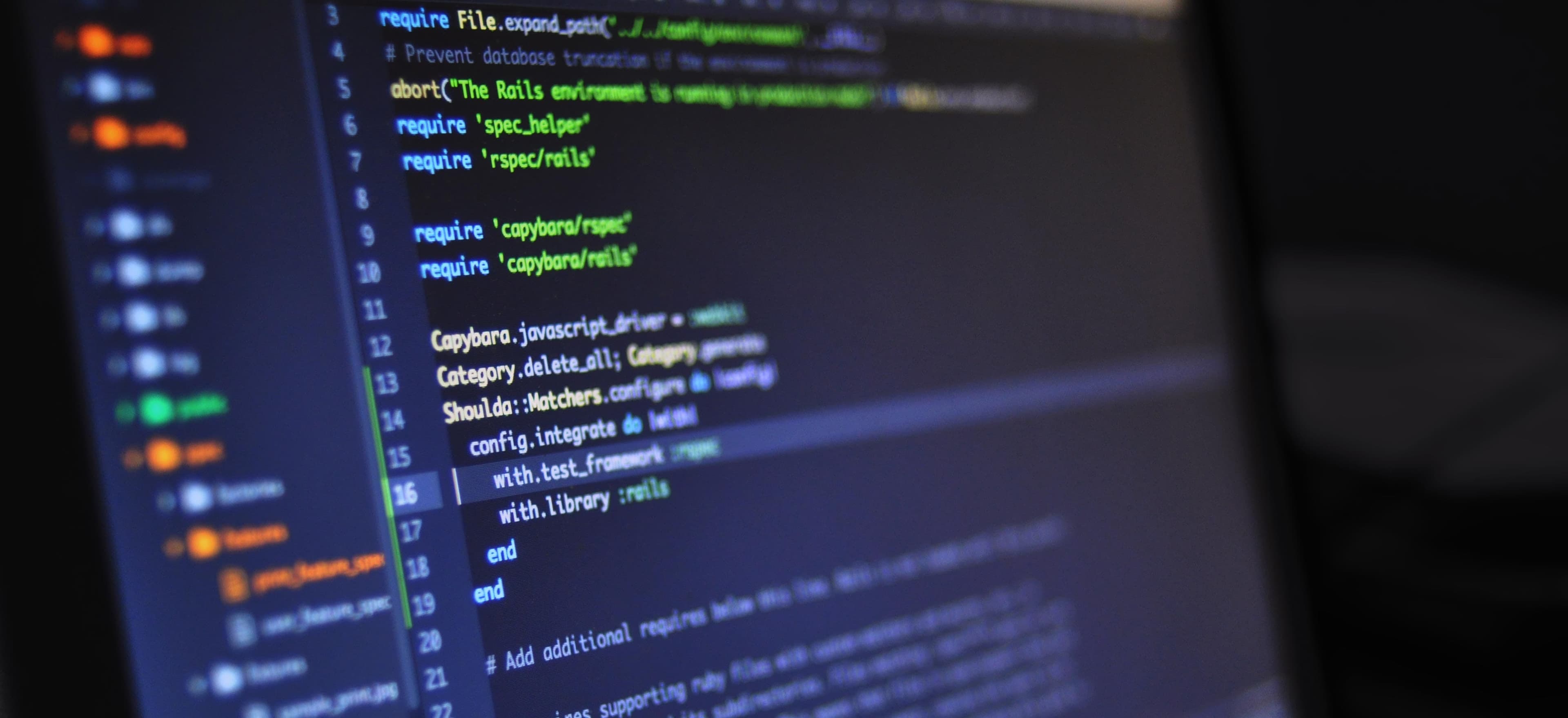
- Published on
Efficient Data Fetching: Java Solutions for SQL Pagination
Data is the lifeblood of modern applications. Efficiently managing and retrieving this data is essential for providing a seamless user experience. One common challenge in data management is ensuring that large datasets can be fetched efficiently without overwhelming the server or the client. This is where SQL pagination comes into play. In this article, we will delve into how to implement pagination in Java and optimize your API requests.
What is SQL Pagination?
SQL pagination refers to the process of dividing large datasets into smaller, more manageable chunks or pages. It enables applications to retrieve only a subset of data at a time, which improves performance and reduces memory usage.
Why Use Pagination?
Here are a few reasons:
- Improved Load Times: Fetching only a portion of data improves the speed at which users can access it.
- Optimized Database Usage: This reduces the strain on the database server when handling large queries.
- Better User Experience: Users can find what they need quicker, encouraging them to stay longer on your application.
For a deeper understanding of optimizing APIs and mastering SQL pagination and filtration, check out Optimizing APIs: Mastering SQL Pagination & Filtration.
Setting Up the Environment
Before we dive into the code, let’s ensure that our development environment is ready for building a Java application that utilizes SQL pagination.
Prerequisites
- Java Development Kit (JDK)
- Maven or Gradle for dependency management
- A database (e.g., MySQL, PostgreSQL)
- JDBC driver for your selected database
- An IDE (e.g., IntelliJ IDEA, Eclipse)
Example Database Schema
Let's create a basic table to work with:
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100) NOT NULL,
email VARCHAR(100) NOT NULL UNIQUE
);
In this example, we will be working with a users
table containing user data.
Implementing Pagination in Java
To demonstrate pagination in Java, we can utilize JDBC to connect to the database and execute SQL queries. Below is a step-by-step guide along with code snippets.
Step 1: Setting Up the Database Connection
You need to establish a connection to the database. Here’s a basic implementation:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnector {
private static final String URL = "jdbc:mysql://localhost:3306/your_database";
private static final String USER = "your_username";
private static final String PASSWORD = "your_password";
public static Connection connect() {
try {
return DriverManager.getConnection(URL, USER, PASSWORD);
} catch (SQLException e) {
e.printStackTrace();
return null;
}
}
}
Why Use This Code?
This code initializes a connection to the MySQL database using JDBC. It is essential because any data fetching operations rely on a stable database connection.
Step 2: Implementing Pagination Logic
Now that we can connect to the database, we can implement the pagination functionality. We will create a method that retrieves a specific page of users based on the requested page number and page size.
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
public class UserService {
public List<User> getUsers(int pageNumber, int pageSize) {
List<User> users = new ArrayList<>();
String query = "SELECT * FROM users LIMIT ? OFFSET ?";
try (Connection connection = DatabaseConnector.connect();
PreparedStatement preparedStatement = connection.prepareStatement(query)) {
int offset = (pageNumber - 1) * pageSize;
preparedStatement.setInt(1, pageSize);
preparedStatement.setInt(2, offset);
ResultSet resultSet = preparedStatement.executeQuery();
while (resultSet.next()) {
users.add(new User(resultSet.getInt("id"), resultSet.getString("name"), resultSet.getString("email")));
}
} catch (SQLException e) {
e.printStackTrace();
}
return users;
}
}
Why Use Prepared Statements?
Prepared statements are crucial for preventing SQL injection attacks. By using placeholders for parameters, we can safely execute queries without directly incorporating user input.
Step 3: Creating a User Model
To handle user data conveniently, we will create a simple User model class.
public class User {
private int id;
private String name;
private String email;
public User(int id, String name, String email) {
this.id = id;
this.name = name;
this.email = email;
}
// Getters and Setters
public int getId() {
return id;
}
public String getName() {
return name;
}
public String getEmail() {
return email;
}
}
Why Model Classes?
Model classes provide a structured way to manage data. It also makes your code more readable and maintainable, offering easy access to attributes.
Step 4: Testing the Pagination
Finally, we will create a simple main method to test our pagination functionality. In a real-world scenario, you would typically expose this through a REST API.
public class Main {
public static void main(String[] args) {
UserService userService = new UserService();
int pageNumber = 1; // Changing this number retrieves different pages
int pageSize = 10; // Number of records per page
List<User> users = userService.getUsers(pageNumber, pageSize);
for (User user : users) {
System.out.println("ID: " + user.getId() + ", Name: " + user.getName() + ", Email: " + user.getEmail());
}
}
}
Why Test the Pagination?
Testing allows you to see the practical output of your pagination logic. By modifying page numbers, you can confirm that only the correct portion of data is fetched.
In Conclusion, Here is What Matters
SQL pagination is an essential technique for handling massive datasets efficiently. By following the examples in this guide, you can easily implement pagination in your Java applications and significantly enhance both performance and user experience.
Don’t forget to explore more about optimizing your APIs with pagination by reading Optimizing APIs: Mastering SQL Pagination & Filtration.
Next Steps
If you want to take your pagination implementation further, consider:
- Implementing advanced filtering options.
- Adding sorting capabilities to your queries.
- Building a user interface to manage and visualize your paginated data.
Remember, well-implemented pagination is one of the keys to building robust and responsive applications. Happy coding!
Checkout our other articles