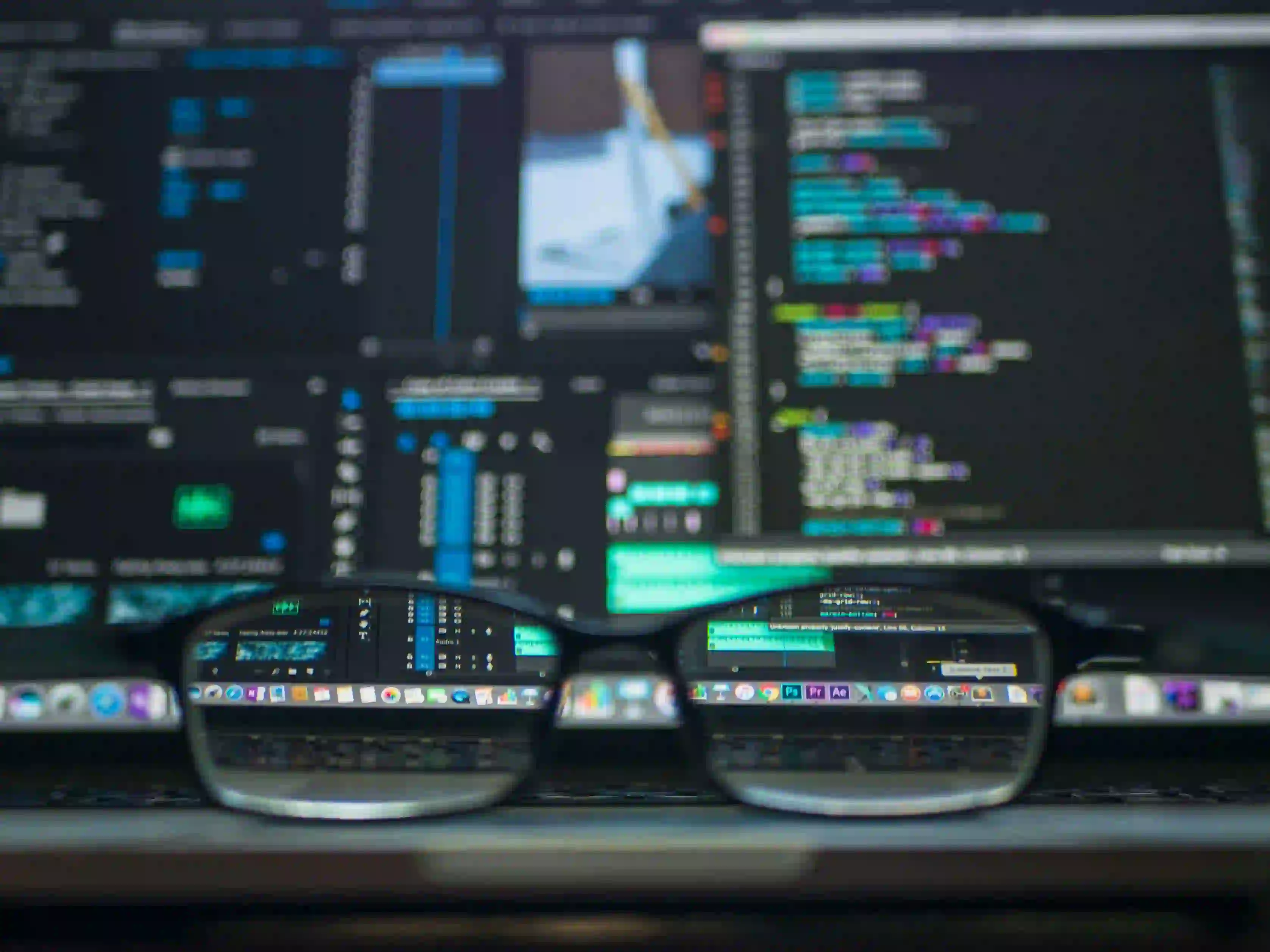
Understanding CORS in Java Applications: A Quick Guide
Cross-Origin Resource Sharing (CORS) is a critical concept every web developer should grasp. It enables or restricts web applications running at one origin (domain) to make requests to resources on another origin. This article aims to demystify CORS, especially in the context of Java applications.
Before diving deeper, if you want a foundational understanding of CORS, I recommend checking out the article titled Demystifying CORS: Solving Cross-Origin Resource Sharing.
What is CORS?
CORS is a security feature built into web browsers that helps control how and when resources can be shared between different origins. In simpler terms, the same-origin policy restricts how a document or script loaded from one origin can interact with resources from another origin. CORS provides a way to overcome this limitation, allowing developers to specify who can access which resources.
Major CORS Concepts:
- Origin: An origin consists of the protocol, domain, and port of a URL. For example,
https://example.com:443
. - Preflight Request: A CORS mechanism where the browser sends an HTTP OPTIONS request to the server before the actual request.
- Access-Control-Allow-Origin: A crucial response header that specifies which origins can access the resources.
Why is CORS Necessary?
Consider a scenario where a frontend application hosted on https://myapp.com
tries to fetch resources from an API hosted on https://api.example.com
. Without CORS, the browser would block this request to protect the user's data and prevent attacks such as Cross-Site Request Forgery (CSRF).
Understanding CORS helps you implement secure web applications while ensuring seamless communication across different services.
Implementing CORS in Java
In Java applications, you can implement CORS in various ways, depending on your framework. Here, we will focus on two popular Java frameworks: Spring Boot and Java Servlet.
1. CORS in Spring Boot
Spring Boot offers built-in support for CORS, making it quite straightforward to configure. Here is a simple example.
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
@EnableWebMvc
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/api/**") // Applying CORS to specific endpoints
.allowedOrigins("https://myapp.com") // Allowing specific origin
.allowedMethods("GET", "POST", "PUT", "DELETE") // Specifying allowed methods
.allowedHeaders("*") // Allowing all headers
.allowCredentials(true); // If credentials are to be included
}
}
Explanation
addMapping("/api/**")
: This applies CORS settings to any endpoint that starts with/api/
.allowedOrigins("https://myapp.com")
: Only requests from this origin are accepted.allowedMethods
: This specifies which HTTP methods are allowed. Adjust these methods based on your application's requirements.allowedHeaders("*")
: This line allows all headers in the CORS request.allowCredentials(true)
: If your application requires cookies, this option allows sending credentials.
2. CORS in Java Servlets
If you are using plain Java Servlets, you'll need to configure CORS manually. Here is how you can do it:
import javax.servlet.Filter;
import javax.servlet.FilterChain;
import javax.servlet.FilterConfig;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.annotation.WebFilter;
import java.io.IOException;
@WebFilter("/*")
public class CorsFilter implements Filter {
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
response.setHeader("Access-Control-Allow-Origin", "https://myapp.com");
response.setHeader("Access-Control-Allow-Methods", "GET, POST, PUT, DELETE, OPTIONS");
response.setHeader("Access-Control-Allow-Headers", "*");
response.setHeader("Access-Control-Allow-Credentials", "true");
// For pre-flight requests
if ("OPTIONS".equalsIgnoreCase(((HttpServletRequest) request).getMethod())) {
response.getWriter().write("");
return;
}
chain.doFilter(request, response);
}
@Override
public void init(FilterConfig filterConfig) throws ServletException {}
@Override
public void destroy() {}
}
Explanation
- The
CorsFilter
class implementsFilter
, allowing us to intercept requests and responses. setHeader
methods add CORS headers for responses. This makes the resource accessible based on the specified policies.- At the end,
chain.doFilter(request, response)
processes the incoming request in the normal flow if the request is not an OPTIONS request.
Debugging CORS Issues
Even with the correct implementation, CORS issues can arise. Common problems include:
- Origin Not Allowed: If the request origin is not in the allowed origins list, it will fail.
- Method Not Allowed: Ensure the methods used in the requests are included in allowed methods.
- Preflight Request Fails: If your server does not handle the OPTIONS method correctly, it will cause problems.
You can diagnose most CORS issues using your browser's developer tools. Look for failed network requests in the "Network" tab for clues.
To Wrap Things Up
Understanding and implementing CORS in your Java applications is crucial for ensuring security while maintaining the flexibility of your web services. Whether you work with Spring Boot or Servlets, proper CORS configuration can mitigate risks while allowing seamless communication between different domains.
To further enhance your understanding of CORS, visit the article titled Demystifying CORS: Solving Cross-Origin Resource Sharing for more insights.
Being aware of CORS is not just about compliance; it’s about enhancing the security posture of your web applications. Proper implementation helps you create a smoother and safer user experience.