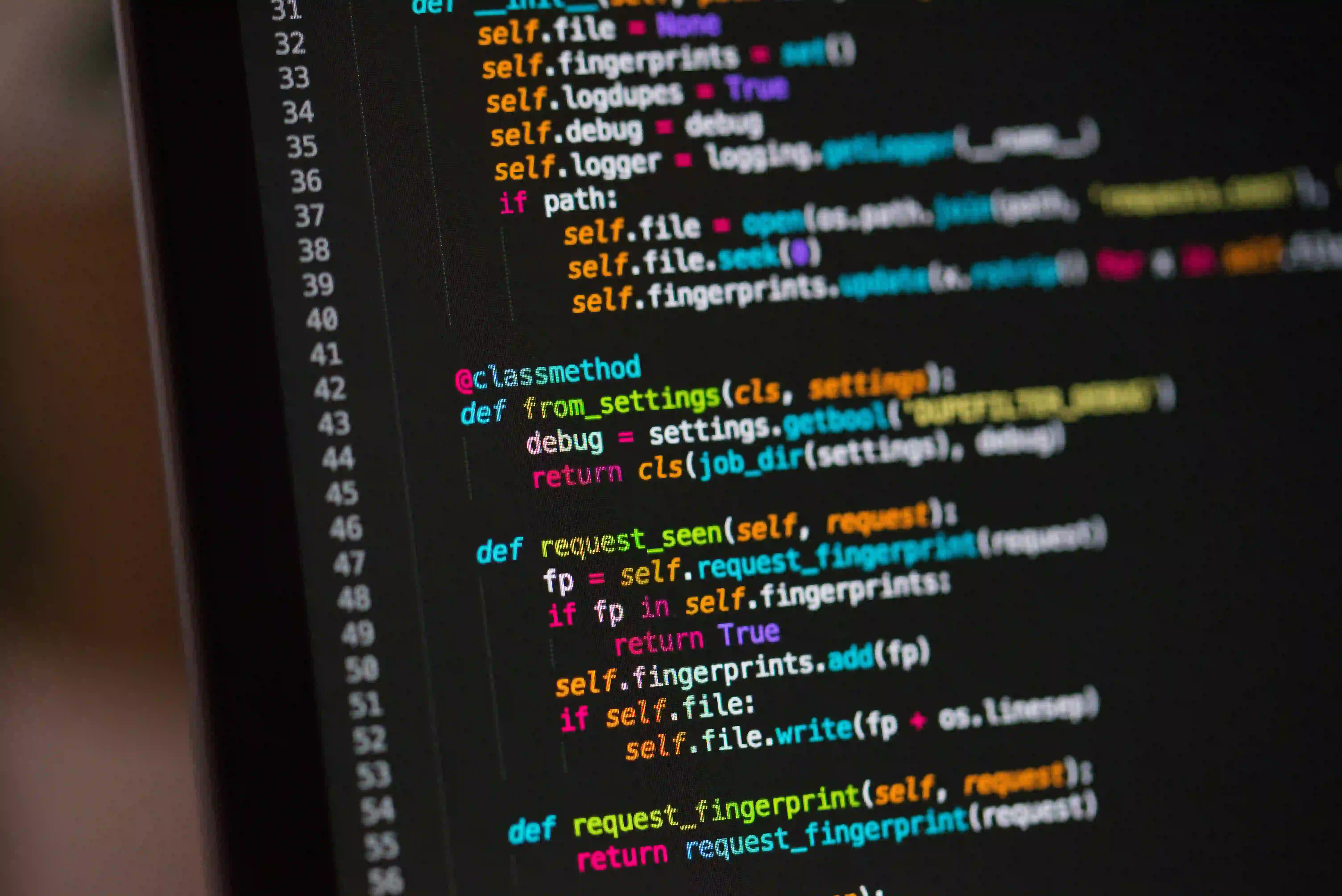
Mastering CSS Flexbox for Java Layouts: Side-by-Side Divs
Creating visually appealing and functional layouts is fundamental in web development. While Java is primarily known for backend development, it often works in tandem with HTML and CSS to present content effectively on the front end. One of the challenges many web developers face is arranging elements on a page. In this article, we’ll delve into how to use CSS Flexbox to arrange three divs side by side in desktop view—ensuring your Java-based layouts look professional and modern.
What is CSS Flexbox?
CSS Flexbox, short for "Flexible Box Layout," is a powerful layout model introduced in CSS3. It allows developers to design complex layouts in a more efficient and predictable manner. The main advantage of using Flexbox is its ability to allow items within a container to be dynamically arranged and sized without the need for clearing floats or using explicit positioning.
Benefits of Using Flexbox:
- Responsive Design: Easily control the layout on different screen sizes.
- Alignment Control: Align items both vertically and horizontally without complex calculations.
- Order Manipulation: Change the visual order of the content without modifying the HTML.
Getting Started
Before diving into code, let’s set up a simple Java Servlet that serves an HTML page. This basic setup will help us focus on the CSS and layout part.
Java Servlet Setup
Here’s a straightforward Servlet that serves a basic HTML page:
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
public class SimpleLayoutServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html>");
out.println("<head>");
out.println("<link rel='stylesheet' type='text/css' href='styles.css'>");
out.println("</head>");
out.println("<body>");
out.println("<div class='container'>");
out.println("<div class='box'>Div 1</div>");
out.println("<div class='box'>Div 2</div>");
out.println("<div class='box'>Div 3</div>");
out.println("</div>");
out.println("</body>");
out.println("</html>");
}
}
Understanding the Code
- HttpServlet: The main class extending
HttpServlet
, where we override the doGet method to handle GET requests. - PrintWriter: Outputs HTML to the client.
- HTML Structure: Each
div
has a class of "box," and they are all within a parentdiv
called "container." This structure will be styled with CSS Flexbox.
CSS Flexbox Properties
Now, let's create the styles.css
file to add some styles using Flexbox.
body {
font-family: Arial, sans-serif;
margin: 20px;
}
.container {
display: flex; /* Enables Flexbox */
justify-content: space-between; /* Distributes space between items */
}
.box {
background-color: lightblue; /* Background color for visual clarity */
padding: 20px;
flex: 1; /* Allows the boxes to grow equally */
margin: 10px; /* Adds spacing between boxes */
text-align: center;
}
Explanation of the CSS Rules
- display: flex: Declares the container as a flex container, enabling Flexbox properties for child elements.
- justify-content: space-between: Distributes the space evenly between the flex items, making them appear side by side.
- flex: 1: This allows all boxes to take equal space within the container. Change this value to manipulate the size distribution between boxes.
- margin: Provides a gap between the boxes, enhancing the layout's visual appeal.
Complete Code Walkthrough
Let’s put everything together with the following directory structure:
/webapp
|-- SimpleLayoutServlet.java
|-- styles.css
Now, with the Java Servlet and CSS File ready, deploy your application and navigate to the servlet's URL. You should see three divs displayed side by side.
Why Use Flexbox?
Using Flexbox simplifies your CSS in comparison with traditional layout methods, such as floating elements. It aids in maintaining your layout without the complexities associated with float clearing and positioning.
If you’re looking to enhance your layout capabilities further, check out the article titled Struggling to Arrange Three Divs Side by Side in Desktop View.
Advanced Flexbox Techniques
While the above example demonstrates basic usage, Flexbox has several advanced features to explore:
Aligning Items Vertically
You can use align-items
to control vertical alignment within the flex container. Here’s how you can modify the .container
class to vertically center the boxes:
.container {
display: flex;
justify-content: space-between;
align-items: center; /* Vertically centers items */
}
Responsive Adjustments
Flexbox makes it easy to create responsive designs. You can implement media queries to change the layout based on screen size:
@media (max-width: 600px) {
.container {
flex-direction: column; /* Stacks items vertically on small screens */
}
}
Lessons Learned
Mastering CSS Flexbox is essential for any web developer, especially when pairing it with Java technologies. By understanding how to arrange divs side by side effectively, you can create appealing and functional layouts for your web applications.
In this article, we've covered the basics of setting up a Java servlet, implementing CSS Flexbox for layout, and touching on responsive design features. With this knowledge, you are now equipped to tackle your layout challenges with confidence.
For more guidance on complex layouts, the article on arranging three divs side by side in desktop view could further benefit your understanding and application of these concepts. Happy coding!