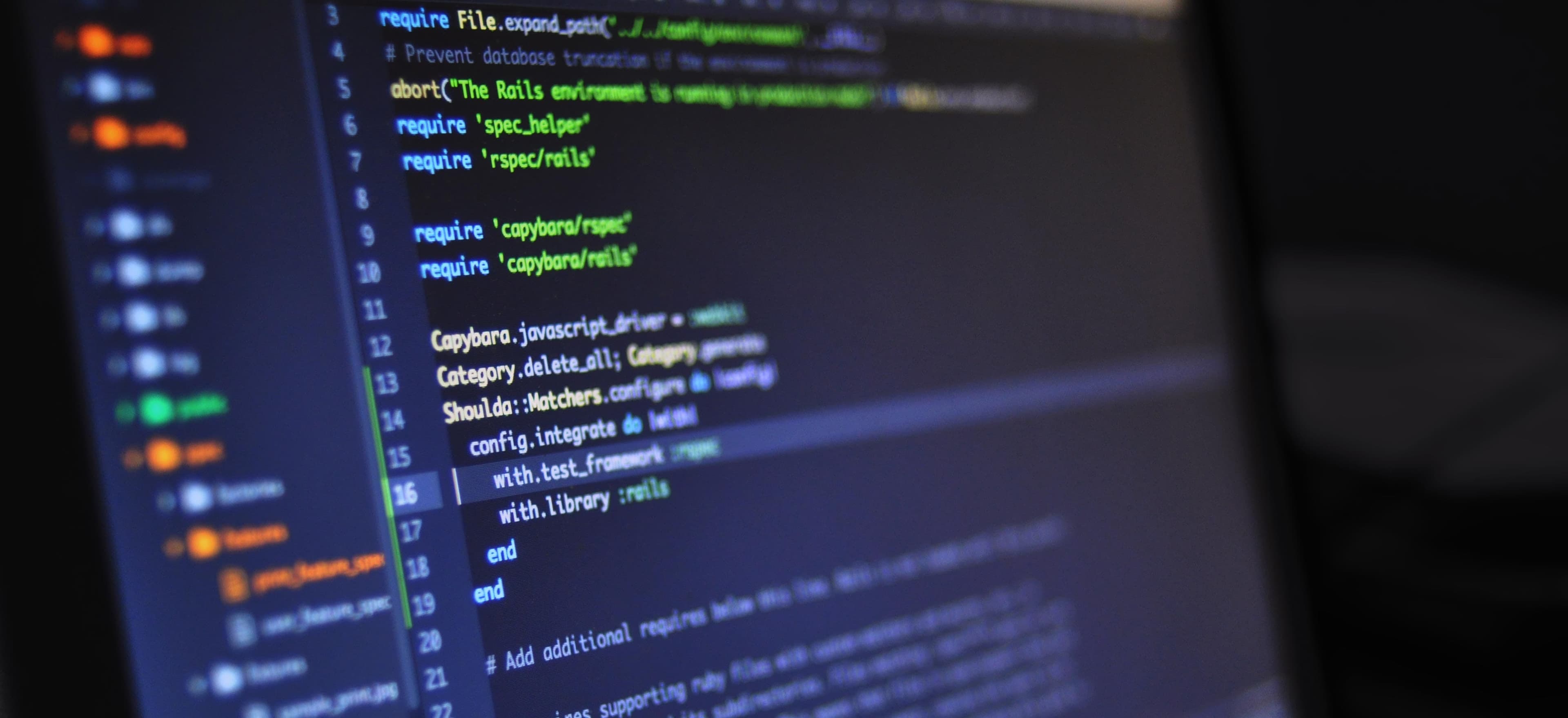
- Published on
Mastering Flexbox: Aligning Three Divs in Java Web Apps
In the world of web development, layout plays a crucial role in the user experience. Well-aligned elements not only enhance aesthetic appeal, but they also improve functionality and usability. If you’re working on a Java web application, mastering CSS Flexbox can help you align your elements—such as divs—effortlessly. This post will guide you through the ins and outs of Flexbox, specifically focusing on how to arrange three divs side by side in a desktop view.
What is Flexbox?
Flexbox, or the Flexible Box Layout, is a CSS layout model designed to provide a more efficient way to lay out and align elements in a container—even when their sizes are unknown or dynamic. Utilizing Flexbox enables you to distribute space naturally among items in a container, making it perfect for responsive design and fluid layouts.
Setting Up Your HTML Structure
Let's start with a simple HTML structure to hold our three divs. Prepare your HTML file with the following markup:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Flexbox Example</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="container">
<div class="box box1">Box 1</div>
<div class="box box2">Box 2</div>
<div class="box box3">Box 3</div>
</div>
</body>
</html>
Analyzing the Structure
This HTML consists of a container that holds three individual boxes. By adding CSS styles later, you will be able to align these divs effectively side by side in a desktop view. The classes box1
, box2
, and box3
let you target each div separately if needed.
Styling with CSS Flexbox
Next, let’s dive into CSS. Create a styles.css
file and add the following code to implement Flexbox:
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
}
.container {
display: flex; /* Enable Flexbox for the container */
justify-content: space-between; /* Space out children evenly */
padding: 20px; /* Add some padding to the container */
background-color: #f0f0f0; /* Background color for visual contrast */
}
.box {
flex: 1; /* Each box will take equal space */
margin: 0 10px; /* Add some margin between boxes */
padding: 20px; /* Padding inside each box */
background-color: #4CAF50; /* Box color */
color: white; /* Text color */
text-align: center; /* Center text horizontally */
border-radius: 5px; /* Rounded corners */
}
Explanation of CSS Properties
-
display: flex: This turns the container into a flex container, allowing its children (the boxes) to be flex items.
-
justify-content: space-between: This evenly distributes the space between the boxes. The first box aligns to the start of the container, the last box aligns to the end, and the remaining space is filled with equal gaps.
-
flex: 1: This property allows each box to grow and occupy an equal share of the available space within the container.
-
margin: It adds spacing between the boxes, enhancing visibility.
-
padding: Ensures the content within each box has enough breathing room.
Testing the Layout
Once you have implemented your HTML and CSS, you can open your HTML file in any modern browser. You should see the three boxes aligned side by side. This layout will automatically adjust based on the width of your browser window due to the responsive capabilities of Flexbox.
Making It Responsive
With web applications increasingly being used on various devices, responsiveness is paramount. To ensure your layout works across different screen sizes, you can use media queries. Below is an example of how to modify your CSS to accommodate smaller screens:
@media (max-width: 768px) {
.container {
flex-direction: column; /* Stack boxes vertically on smaller screens */
}
.box {
margin: 10px 0; /* Adjust margins to stack properly */
}
}
Understanding Media Queries
-
@media (max-width: 768px): This rule applies styles to screens that are 768 pixels wide or narrower, a common breakpoint for tablets and mobile devices.
-
flex-direction: column: Changing the direction from the default row to column stacks the boxes vertically.
Now, your layout dynamically adapts based on screen size—an essential feature for modern web applications.
Real-World Application in Java Web Apps
When building Java web applications, you often use frameworks like Spring or JavaServer Faces (JSF). The principles of Flexbox remain the same. You can use similar markup in JSF by utilizing Facelets or other template engines. Here’s an example using JSF:
<ui:composition xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:ui="http://java.sun.com/jsf/facelets">
<h:form>
<div class="container">
<div class="box">Box 1</div>
<div class="box">Box 2</div>
<div class="box">Box 3</div>
</div>
</h:form>
</ui:composition>
Integrating The CSS
The same styles.css
file can be linked here, ensuring that the styling remains consistent throughout your application.
Further Learning
To understand the intricacies of CSS layout properties further, consider reading about CSS Grid Layout. While Flexbox is ideal for a one-dimensional space arrangement, Grid excels in two-dimensional layouts.
In addition, if you're struggling with div arrangements or related layout questions, take a look at the article titled Struggling to Arrange Three Divs Side by Side in Desktop View. The fundamentals discussed in that article will complement what you've learned here.
Closing the Chapter
Mastering Flexbox is a vital skill for any web developer. Its ability to create flexible, responsive layouts makes it an invaluable tool in your toolkit. By following this guide, you should now have a solid foundation for aligning three divs side by side in desktop views, along with tips for ensuring your layout remains user-friendly on mobile devices.
Flexbox is a game changer. Keep exploring its various properties, experiment with different layouts, and enhance your Java web applications with beautiful, fluid designs. Happy coding!
Checkout our other articles