Understanding CORS in Java: Overcoming Cross-Origin Issues
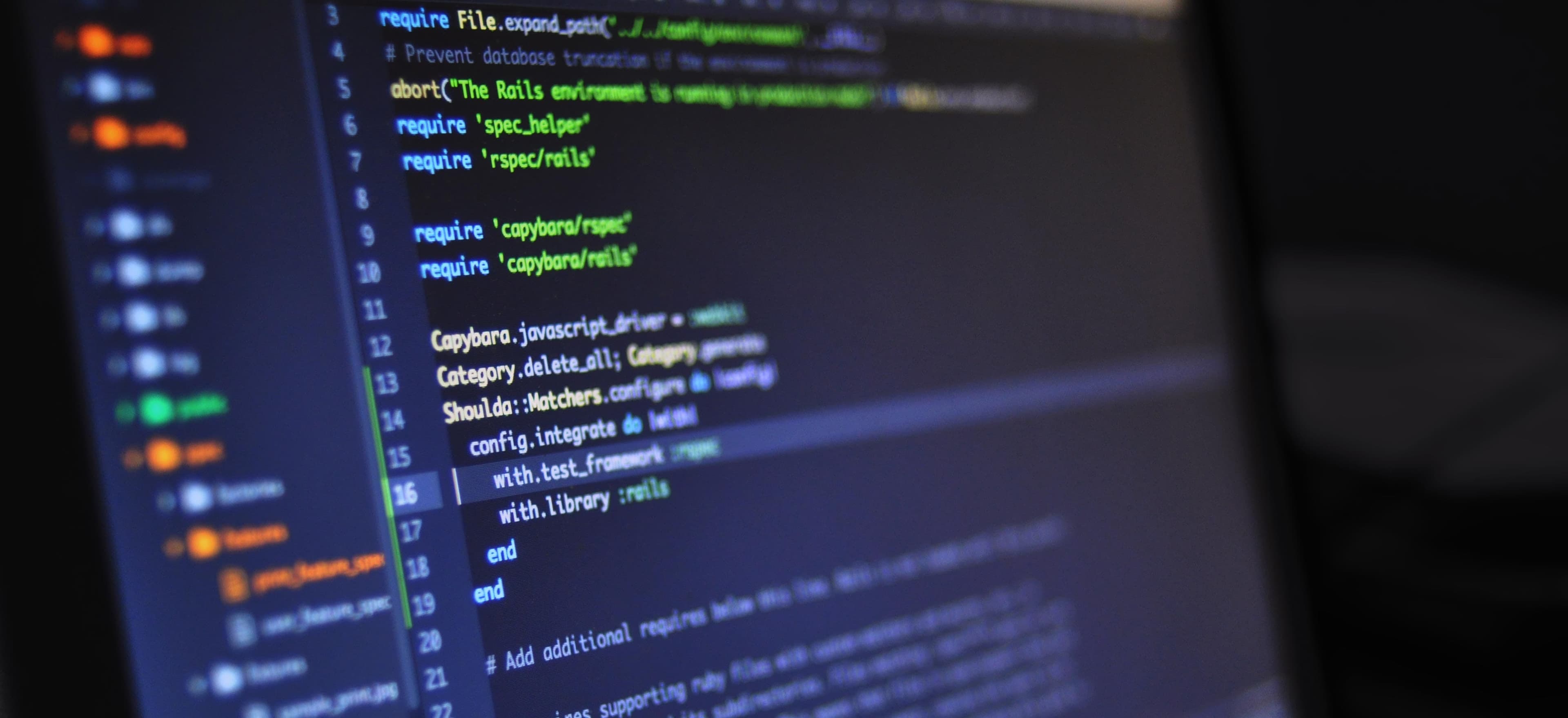
- Published on
Understanding CORS in Java: Overcoming Cross-Origin Issues
In the world of web development, Cross-Origin Resource Sharing (CORS) has become a buzzword, often raising questions and concerns among developers. CORS is a mechanism that allows restricted resources on a web page to be requested from another domain outside the domain from which the first resource was served. In this blog, we will explore what CORS means, its significance, and how to implement it in a Java application.
Table of Contents
- What is CORS?
- Understanding the Same-Origin Policy
- Why is CORS Important?
- Implementing CORS in Java
- Example: A Simple REST API with CORS
- Testing CORS
- Conclusion
What is CORS?
CORS is a protocol that allows web applications running at one origin to make requests to resources from a different origin. This is crucial for enabling APIs to communicate with web apps securely. It facilitates resource sharing between different domains while ensuring security through specific HTTP headers.
In practical terms, when you attempt to make a request to a different domain, such as an API hosted elsewhere, the browser checks the CORS policy before proceeding with the request. If the policy is not satisfied, the browser blocks the request.
Understanding the Same-Origin Policy
Before diving deeper, it’s important to understand the Same-Origin Policy. This security measure restricts how documents or scripts loaded from one origin can interact with resources from another origin. The same-origin policy is defined by the scheme (protocol), host (domain), and port.
For example:
- https://example.com:443
- https://example.com:80
Both of these are considered different origins, even if they share the same domain name.
This policy is essential for maintaining the security and integrity of web applications, preventing unwanted behavior such as data theft or cross-site scripting attacks.
Why is CORS Important?
CORS is critical because it provides a way to securely allow cross-origin requests while maintaining the integrity of the web. By enabling CORS, you give your application the capability to request resources from multiple sources, making it more versatile and connected.
For example, a front-end application hosted on https://myapp.com
may need to fetch data from an API hosted on https://api.myapp.com
. Without CORS, this request would be blocked. Allowing such interactions broadens the horizon for developers and creates a more interconnected web.
For a deeper understanding of CORS and the security implications associated with it, check out the article titled "Demystifying CORS: Solving Cross-Origin Resource Sharing."
Implementing CORS in Java
Implementing CORS in Java typically involves configuring your Java application server or your APIs. The implementation can vary based on whether you are using Spring Boot, Java Servlets, or another framework.
CORS Configuration in Spring Boot
Spring Boot offers a straightforward way to enable CORS globally or for specific endpoints.
Global CORS Configuration
To enable CORS globally, you may use the addCorsMappings
method from the WebMvcConfigurer
interface. Here's an example:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/api/**") // Specifies the URL pattern
.allowedOrigins("https://myapp.com") // Allows only this origin
.allowedMethods("GET", "POST") // Allows these HTTP methods
.allowedHeaders("*") // Allows any headers
.allowCredentials(true); // Allows cookies
}
}
Commentary: The addMapping
method defines which endpoints will be accessible. The allowedOrigins
specifies which domains can call your API. It is a good practice to restrict this to known origins to mitigate security risks.
CORS Configuration for Specific Controllers
You can also handle CORS on a finer level, applying it only to specific controllers.
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@CrossOrigin(origins = "https://myapp.com") // CORS for this specific controller
public class MyController {
@GetMapping("/api/data")
public String getData() {
return "Hello, CORS-enabled world!";
}
}
Commentary: The @CrossOrigin
annotation allows you to specify the CORS policy at the controller or action level, offering flexibility in situations where different endpoints may require different policies.
Example: A Simple REST API with CORS
Let's build a basic REST API using Spring Boot that supports CORS.
-
Spring Boot Application Setup Create a Spring Boot application and add the following dependencies in your
pom.xml
.<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency>
-
Create a Controller
@RestController @CrossOrigin(origins = "https://myapp.com") public class HelloWorldController { @GetMapping("/api/hello") public String helloWorld() { return "Hello, World!"; } }
-
Run the Application After building your project, run the Spring Boot application.
-
Test the API You can test this API using Postman or a simple fetch request in your front-end application.
fetch('http://localhost:8080/api/hello') .then(response => response.text()) .then(data => console.log(data)) // Expected output: "Hello, World!" .catch(error => console.error("Error:", error));
Testing CORS
Once you've implemented your API, testing CORS is essential to ensure that your configurations work as intended. You can do this by using browser developer tools, or tools like Postman that allow you to manipulate request headers.
-
Using Developer Tools Open the Chrome Developer Tools (F12) and navigate to the "Network" tab. Make a request from a different origin and check the response headers to see if CORS headers such as
Access-Control-Allow-Origin
are present. -
Simple Fetch Test Write a simple JavaScript fetch to a different origin and monitor for CORS errors in the console.
Bringing It All Together
CORS is a powerful mechanism that allows safe cross-origin requests. In Java, particularly with frameworks like Spring Boot, implementing CORS is straightforward yet critical for developing web applications that interact with multiple domains. Through this blog, we have delved deep into understanding CORS, how it works, and how to set it up in a Java application.
If you're interested in expanding your knowledge, remember to read the article on "Demystifying CORS: Solving Cross-Origin Resource Sharing." Embracing CORS will help you create robust applications that are ready for the evolving ecosystem of the web. Happy coding!
Checkout our other articles